Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial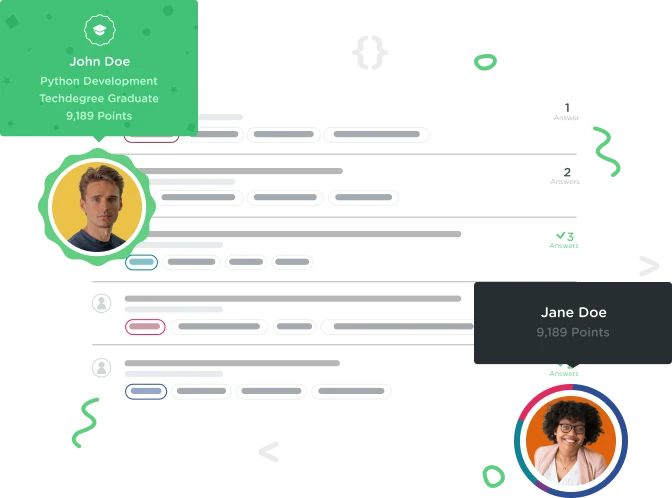
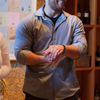
Jonathan Ankiewicz
17,901 Pointshow to return a map
This challenge doesn't seem to render completely with me... I feel lost at taking a map in from a parameter and returning it back
package sales
func HalfPriceSale(prices map[string]float64) map[string]float64 {
// YOUR CODE HERE
price :=
}
1 Answer

Joe Purdy
23,237 PointsMaps in Go are a key/value data store type. Since Go is a strongly typed language you need to be explicit in defining the type of a map's keys and its values.
Go has a really useful keyword called range
for iterating over a map value using a for loop. To use the range
keyword to iterate over a map you would write a for loop like this:
package main
import "fmt"
func main() {
prices := make(map[string]float64)
prices["OCKOPROG"] = 89.99
prices["ALBOÖMME"] = 129.99
prices["TRAALLÅ"] = 49.99
for key, value := range prices {
fmt.Printf("Key: %s\tValue: %v\n", key, value)
}
}
To see the output of this you can check it out in the Go Playground here: https://play.golang.org/p/FRhvZAZLC1
Expanding on this you can create a new map in your function and assign new values to this map while looping over a map passed to the function like in this DoublePrice
function:
package main
import "fmt"
func main() {
prices := make(map[string]float64)
prices["OCKOPROG"] = 89.99
prices["ALBOÖMME"] = 129.99
prices["TRAALLÅ"] = 49.99
doubled := DoublePrice(prices)
for key, value := range doubled {
fmt.Printf("Key: %s\tValue: %v\n", key, value)
}
}
func DoublePrice(prices map[string]float64) map[string]float64 {
doublePriced := make(map[string]float64)
for key, value := range prices {
doublePriced[key] = value * 2
}
return doublePriced
}
Once again this is interactive and you can run it here: https://play.golang.org/p/_BDb6J_xak
The important bit to examine is the DoublePrice
function:
func DoublePrice(prices map[string]float64) map[string]float64 {
doublePriced := make(map[string]float64)
for key, value := range prices {
doublePriced[key] = value * 2
}
return doublePriced
}
This function follows the same style as what the challenge uses for the HalfPriceSale
function. It takes a single argument named prices
which is a map with keys of type string and values of type float64. The function then returns a map with the same types for its keys and values.
Inside the function the first thing that happens is a new map is created with string keys and float64 values and assigned to the variable doublePriced
. Next there's a for loop that iterates over the range of the prices
map which was passed in as an argument to the function. Inside this for loop we assign values to the doublePriced
map created earlier using the key
variable for the key and the result of running a simple math operation doubling the value
.
Once the for loop finishes the doublePriced
map will have a key matching each key from the prices
map and the value in the doublePriced
map will be twice as much as what the value was in the prices
map. The last thing to do in the function is simply to return the doublePriced
map.
To solve the challenge for halving the prices you'd follow this same process, but you'll need to modify the value assignment in the for loop to halve the value instead of doubling it.
I hope that helps give you some fresh ideas and it's totally normal to get mixed up when starting work with maps for the first time in Go. They can be a complex data type to wrap your head around so I'd encourage re-watching the Maps lesson video a couple times to unravel how they work. It also helps to try experimenting with them in the interactive Go Playground or in the Tour of Go
Happy coding!
Jonathan Ankiewicz
17,901 PointsJonathan Ankiewicz
17,901 Pointssorry to be a pain, I actually resolved it. forgot to mark it here.
Joe Purdy
23,237 PointsJoe Purdy
23,237 PointsGlad to hear you sorted it out!