Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial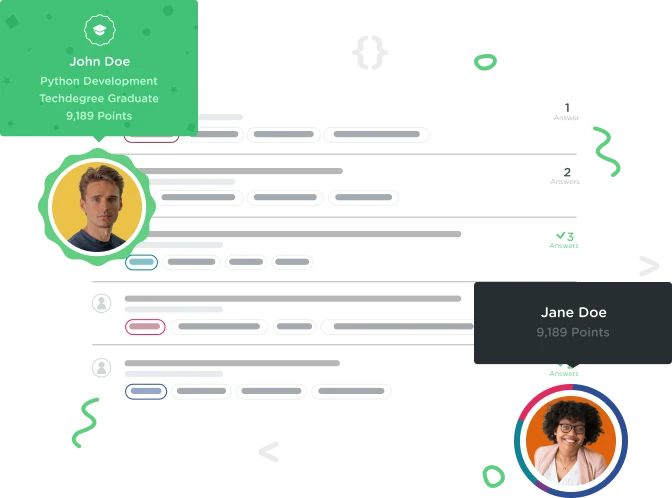

Taylor Porter
2,338 PointsHow to shorten all of your Or Operators (||) (If there IS a way)
After I completed The Or (||) Operator challenge, I thought how do you shorten this so I don't repeat myself.
def valid_command?(command)
if (command = "y") || (command = "yes") || (command = "Y") || (command = "YES") return "true"
end end
1 Answer
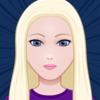
Angela Visnesky
20,927 PointsHi Taylor, You could shorten it by :
def valid_command?(command)
if command.upcase = "Y" || command.upcase = "YES"
return true
end
end
You could also do the same with downcase. I hope this helps!
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsThat's probably about the shortest you can get it using the || operator.
One further simplification could be:
Oh, I just realized that the code posted contains single equal signs. These should be double equal signs. We're doing an equality comparison here and not assignment.
I don't think it was taught in the course but arrays have an
include?
method that makes doing this sort of thing easier.This basically checks if
command
matches one of the elements in the array and returns true if it does or false otherwise.And you could also shorten the array down to 2 elements if you call the upcase or downcase method on command like Angela showed in her code.
Doing it like this would be especially useful if you had something like 10 commands to check against.