Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial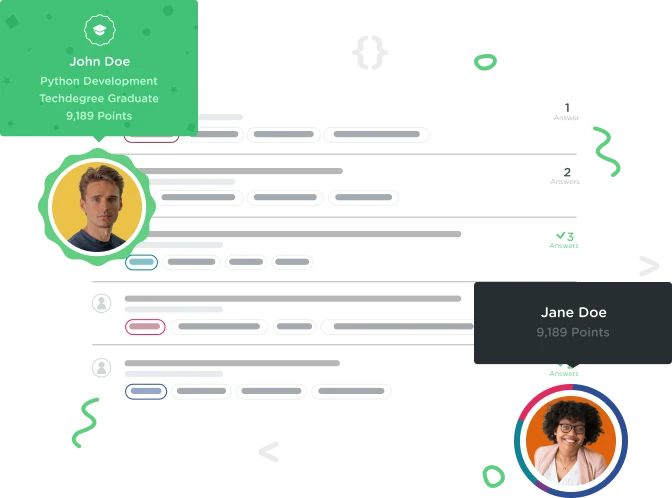

David Jones
1,681 PointsHow to start my for each loop
I have a few questions with this problem I am working on
Starting from the very beginning I was not sure what type(char int or String) to declare the method. I chose String because it seemed like that is how it would be used. Maybe this was the main issue?
I kept getting a bummer message asking if I forgot to create a method that could accept a char so I declared a char in the parameter named tile. But when I try to start to start my for each loop I get a compiler error saying that "tile" is not a good way to start even though that is the thing I want to pull out. Also I did not get a compiler error when "tile was declared in the loop instead of in the parameter of the method.
Since we need a counter it seemed like we need an int instead of a char (I was having trouble adding char numbers together). I wasn't sure how to reconcile this with the method type so I checked stackoverflow not sure if what I did was correct or if there was a way to do this without needing the method I used.
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
int counter;
public String getTileCount(char tile) {
for (tile : mHand.toCharArray()) {
counter++;}
return Integer.toString(counter);
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
}
2 Answers
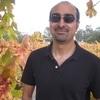
Kourosh Raeen
23,733 PointsHi David - A few observations:
Since the method is supposed to return the number of times a specific tile occurs in
mHand
the return type needs to beint
.You've declared the variable
counter
outside the method. Move that inside the method, before the for-each loop, and initialize it to zero.Choose a different name for the loop variable since you are already using
tile
for the method parameter.In the for-each loop you need to define the type of the loop variable:
for (char c : mHand.toCharArray()) {
}
- Inside the loop use and if statement to see if
tile
is equal to the current character in the array. If it is then incrementcounter
.
Hope this helps.
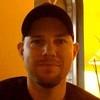
Jeremy Hill
29,567 PointsFirst thing you need to do is know what the method is supposed to return- notice that you have it returning a String when the name of the method is getTileCount(). Just looking at the method title it tells me that it should return a number: public int getTileCount()