Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial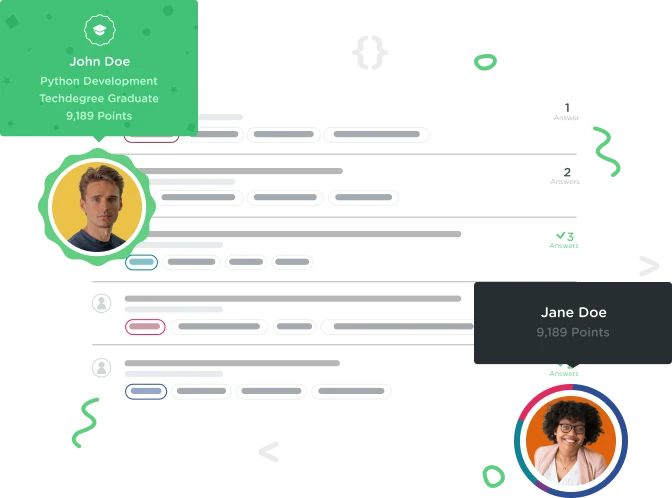

saurabh yadav
3,367 Pointshow to use conditional statement for this purpose
argument related problem
function max(5,9){
if (5>9){alert('true')}
else{alert('false')}
return max;
}
2 Answers
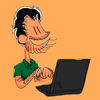
Juan Martin
14,335 PointsHello Saurabh,
You're almost there! The errors I see are the following:
-- For Task 1 --
1) In order to create the max function, you must include the parameters that the function needs, and these parameters are variables names, not numbers.
2) When comparing both arguments passed into the parameters, the function must "return" the one that's greater than the other.
Here's a solution:
function max(number1, number2) {
var maxNumber;
if (number1 > number2) {
maxNumber = number1;
} else {
maxNumber = number2;
}
return maxNumber;
}
--- For Task 2 --
1) After the function is created, you must call it and include the 2 numbers you want to compare. Also, you have to display the results in an alert dialog.
Here's how you can solve it:
function max(number1, number2) {
var maxNumber;
if (number1 > number2) {
maxNumber = number1;
} else {
maxNumber = number2;
}
return maxNumber;
}
alert(max(3,4));
Hope this helps!

Zachary Kaufman
1,463 PointsAh okay so the website will assign the two arguments. You just need to name them something. So when I did this project I had
function max(num1, num2)
but you can call them whatever you want. Don't use actual numbers, we want it so that later you can assign num1 or num2 whatever number is relevant to the program. (Also, your return values should return the numbers, not a boolean) Great job! You were so close, keep up the good work.