Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial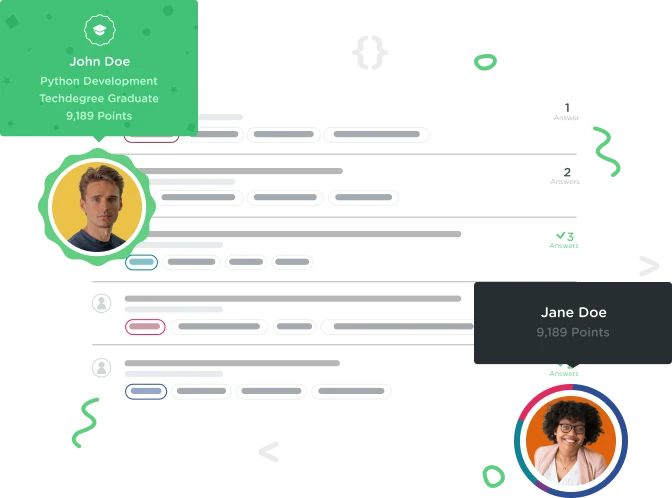
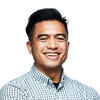
Neil Martin Orbase
4,137 PointsHow would I go about in enabling the same HTML text to display if a user clicks "cancel" on the prompt?
...as in, if a user clicks 'cancel ' on one or all of the pop-up prompts, how would I get the code to display the same result as a user that literally answered each question incorrect?
Also, is my code using let/const formatted/used correctly?:
const questions = [
["How many Disney resorts are there in the U.S.?", 2],
["What is Mickey Mouse's significant other's full name?", "MINNIE MOUSE"],
["Who is Goofy's son?", "MAX"],
["What animal is Daisy?", "DUCK"],
["What city in Japan is the Disney resort located in?", "TOKYO"],
["'Tooncity' is one of the attractions at Disneyland. (True or False?)", "FALSE"]
];
function print(message) {
let outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function buildList(arr) {
let listHTML = '<ol>';
for (let i = 0; i < arr.length; i++) {
listHTML += '<li>' + arr[i] + '</li>';
}
listHTML += '</ol>';
return listHTML;
}
let correctAnswers = 0;
let correct = [];
let wrong = [];
for (let i = 0; i < questions.length; i++) {
let question = questions[i][0];
let answer = questions[i][1];
let response = prompt(question);
if (typeof answer !== "string") {
response = parseInt(response);
}
if (typeof answer === "string") {
response = response.toUpperCase();
}
if (response === answer) {
correctAnswers += 1;
correct.push(question);
} else {
wrong.push(question);
}
}
let html = `You got ${correctAnswers} question(s) right.`;
html += '<h2>You got these questions correct:</h2>';
html += buildList(correct);
html += '<h2>You got these questions wrong:</h2>';
html += buildList(wrong);
print(html);
(quiz can also be viewed via my surge.sh static site: http://disney-mini-quiz.surge.sh) On this note ^, after just realizing that opening my static site via a new tab does not open up the prompt pop-up windows (a user would have to refresh)? It works just fine if I visit the site on the same browser window, but not via a "click to open in new tab and having to refresh to see the prompts".
Thank you!
2 Answers

Zimri Leijen
11,835 PointsIf you click cancel, you get null
as a value, if the input was empty, you'll get undefined (doesn't matter if you click "ok" or "cancel" in this case), in all other cases you get a string.
Knowing this, you can handle undefined
, null
and string
differently from each other.
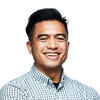
Neil Martin Orbase
4,137 PointsThank you, Zimri Leijen! As for the correct, wrong, question, and answer variables able to be const.. i think i understand, but not quite too sure... I used let because I was assuming that reassigning means any type of altercation at all, including the ability to put new values within those variables that are empty arrays at first, etc. etc. Am I getting the understanding of "reassignment" wrong?

Zimri Leijen
11,835 PointsReassignment is for example
let number = 4;
number = 2;
constants prevent those kinds of reassignments.
however, they do not prevent alteration.
This can lead to interesting behaviors because for example
const object = {color: 'blue', shape: 'triangle'}
object = {color: 'red', shape: 'square'} // TypeError: Assignment to constant variable.
object.color = 'red'; // this is perfectly fine.
so, while you can't directly modify the constant, you can modify the contents of it. This includes array methods like push, splice, pop, etc. And, as demonstrated, you can alter the properties of an object as well, as long as you don't change the object itself directly, but obviously, changing the properties does have an effect on the object!
There are ways to make objects immutable but that's way beyond the scope of this exercise.
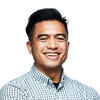
Neil Martin Orbase
4,137 Pointslet correctAnswers = 0;
const correct = [];
const wrong = [];
for (let i = 0; i < questions.length; i++) {
const question = questions[i][0];
const answer = questions[i][1];
let response = prompt(question);
if (response === null || response === undefined || response === '') {
console.log("ERROR:", "User skipped this question: " + question + " Refresh page to try again.")
if (response !== answer) {
wrong.push(question);
}
continue;
}
if (typeof answer === typeof 1) {
response = parseInt(response);
}
if (typeof answer === "string") {
response = response.toUpperCase();
}
if (response === answer) {
correctAnswers += 1;
correct.push(question);
} else {
wrong.push(question);
}
Thank you so much for pointing me to the right direction, Zimri Leijen! I updated my code per your guidance, and... voila! It works!
( http://disney-mini-quiz.surge.sh ) </--- if you wanna check it out! (feel free to use the console viewer as well =] )
Zimri Leijen
11,835 PointsZimri Leijen
11,835 Pointsabout the let/const, there's quite a few you can make a const.
correct, wrong, question and answer can all be const, because you never redefine them (mutating arrays can still be done even if the arrays are consts, constants in JavaScript are not immutable, a little counter-intuitive, but it is what it is).