Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial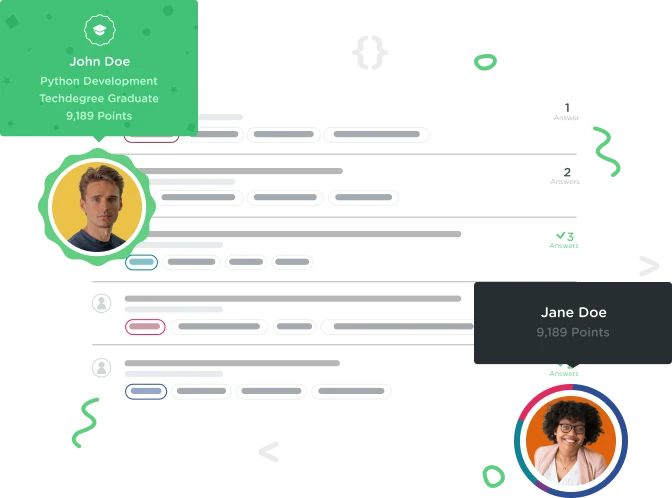

Mister Robot
621 PointsI am almost done finishing the c# basic course,, but I can't seem to figure out how to write loop for this objective?
please help and explain to me
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
}
}
}
2 Answers

andren
28,558 PointsThere are multiple ways you could write a loop of the type the challenge is requesting, the simplest way in my opinion is a for loop like this:
Console.Write("Enter the number of times to print \"Yay!\": ");
int timesToRun = int.Parse(Console.ReadLine()); //Get the user input and convert it to an int
for(int i = 0;i < timesToRun;i++) { //Start a for loop that runs until i is less than the input
Console.WriteLine("Yay!");
}
A for loop let's you define a variable for use in the loop, a condition to run the loop, and an action to do after the loop has run in one single line of code, above I create an index variable called i and set it to 0, then set the condition to i < timesToRun and then specify that I want i to increase by one each time the loop runs (i++).
You might think running the loop until i is less than the input would result in the loop running one times less than desired, but this is not the case due to the fact that i starts at 0, rather than at 1.
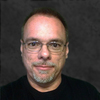
Jim Mason
4,026 PointsOK, did a little research and came up with the following that worked. I wanted to stay with stuff that had been discussed in the course so I used a while loop. The part I was missing initially was understanding how the iteration could be used. Anyway, here is what I came up with.
using System;
namespace Treehouse.CodeChallenges { class Program { static void Main() { Console.Write("Enter the number of times to print \"Yay!\": "); string entry = Console.ReadLine(); int timesToPrint = Int32.Parse(entry); int i = 0;
while(i < timesToPrint)
{
Console.WriteLine("Yay!");
i++;
}
}
}
}
Jim Mason
4,026 PointsJim Mason
4,026 PointsI am having the same problem and while I am sure the answer provided will work it really does nothing to help with understanding how to come up with the solution on my own. The for loop was not even discussed in any of the training so there is good reason I would have never thought of it.
My issue is I am completely new to coding and the basic course just flew by and nothing really stuck in my head. The challenge seems simple enough but for someone that has never coded the content in the course was delivered way to quickly and did not provide a solid base to build on.
That aside the task is to print Yay! for the value entered. I am assuming all the topics of "good" coding that were discussed during the course are not relevant for the challenge being there is nothing addressing entering text or non-integer values?
So, from a practical standpoint that would be educational and not simplest I would like to understand how one would approach this.
Any assistance would be greatly appreciated.
andren
28,558 Pointsandren
28,558 PointsWhile I use a for loop in my proposed solution I did so not realizing that for loops are not thought in the basics course here on Treehouse, I skipped that course on Treehouse since I already knew the basics of C# though my knowledge of Java (which is a very similar languages as far as the basics go) but as I said in my answer there are multiple ways of solving the challenge, using the for loop that I illustrated is the most concise solution but it can be converted to a regular while loop.
I would provide an answer using a while loop but I can see that you have already figured out how to do that on your own.
The best way to learn programming is to practice it as much as you can, and watch as many examples of how to do things as you can, that is at least the way that I tend to learn things.
Jim Mason
4,026 PointsJim Mason
4,026 PointsWell I am learning for sure and the answer you provided was much appreciated though without reviewing the functionality of a for loop it was completely lost on me. lol
In the end this is why I am here and everything helps even if it does not appear to at that moment.
andren
28,558 Pointsandren
28,558 PointsYeah had I known that for loops were not covered in the basics course then I would definitively not have used that as an example of the solution, I have now edited my answer to provide a short explanation of what a for loop is, hopefully that will clear up at least a little confusion.
There have definitively been times where I have watched stuff on here and not really gone away with much on an understanding of how it works, but after seeing it in practice a couple of times and playing around with it myself I usually start to get an understanding of it, good luck to you.