Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial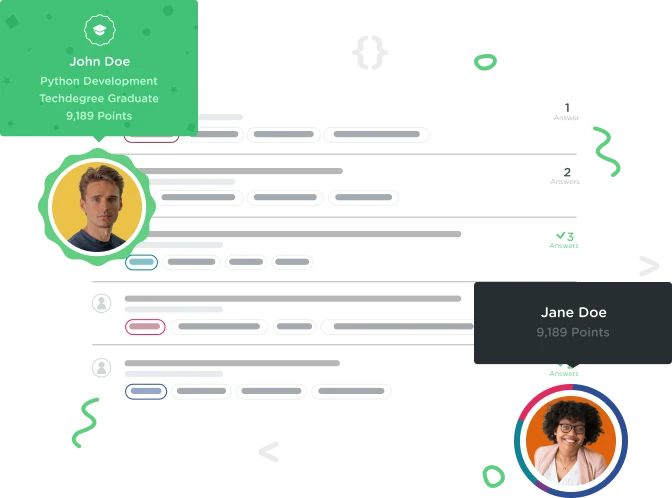

Maria Kochetygova
Courses Plus Student 1,784 PointsI am confused, and don't know how to fix it
What is wrong with my code?
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
int count;
int i=0;
Console.Write("Enter the number of times to print \"Yay!\": ");
count=Console.ReadLine();
while(i<count)
{
try{
int number =int.Parse(count);
}
catch (FormatException){
Console.WriteLine("You must enter a whole number.");
continue;
}
Console.WriteLine("Yay!");
i++;
}
}
}
}
4 Answers
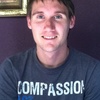
Eric Moody
12,373 Pointsusing System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
var i=0;
Console.Write("Enter the number of times to print \"Yay!\": ");
try
{
var count=Int32.Parse(Console.ReadLine());
while(i<count)
{
Console.WriteLine("Yay!");
i++;
}
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
}
}
}
}
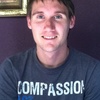
Eric Moody
12,373 PointsJust cleaned it up a bit and removed the extra variable. So you're setting count as an int, setting i = 0. Then you prompt the user for the number of times to print Yay. You then enter a Try/Catch. Inside your Try you put the assignment of the input to count which could actually be var count=Int32.Parse(Console.ReadLine()); if there isn't an exception you'll go into the while loop printing out Yay! the correct number of times. If there is an exception you'll write out the correct message for that.
Cheers....
For easier reading
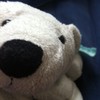
bothxp
16,510 PointsHi Eric,
I think that your use of var count is causing an error.
Program.cs(10,11): error CS0818: An implicitly typed local variable declarator must include an initializer
Program.cs(15,13): error CS0841: A local variable `count' cannot be used before it is declared
Program.cs(16,21): error CS0841: A local variable `count' cannot be used before it is declared
Compilation failed: 3 error(s), 0 warnings
I believe that you need to either initialise it to 0 so that the compiler knows that it is an int or just declare it using int
var count = 0;
int count;

Seth Kroger
56,413 PointsRemember that Console.ReadLine gives you a string, not an int. You have to take that string and convert it to an int for it to work.

Maria Kochetygova
Courses Plus Student 1,784 PointsI would appreciate if you could tell me how to fix it .

Seth Kroger
56,413 Pointsstring response = Console.ReadLine();
count = int.Parse(response);

Maria Kochetygova
Courses Plus Student 1,784 PointsUnfortunately, this is not working, I tried it before

Seth Kroger
56,413 PointsYou also need to take the try/catch block outside of the while loop and have the above inside the try block when you get to that part of the challenge. The only the loop should do is write "Yay!" and increment the loop counter.
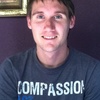
Eric Moody
12,373 PointsThanks, bothxp. Sorry, I just ran it and came back to edit. Guessed I missed that. Should be good now.

Maria Kochetygova
Courses Plus Student 1,784 PointsEric , Thank you so much it worked!
Eric Moody
12,373 PointsEric Moody
12,373 PointsIf you don't mind selecting the answer so other's can know this has been answered successfully.