Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial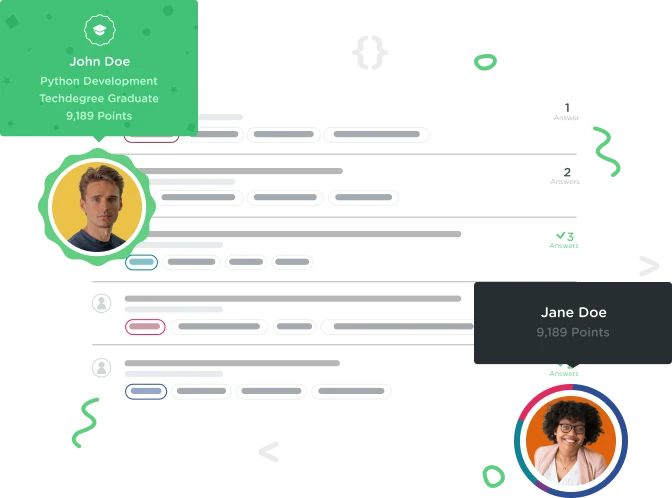
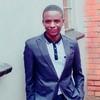
Ashford Makwarimba
6,844 PointsI am failing to get through this challenge where i have to use the while loop and the symbol !.
public class GoKart { public static final int MAX_ENERGY_BARS = 8; private String mColor; private int mBarsCount;
public GoKart(String color) { mColor = color; mBarsCount = 0; }
public String getColor() { return mColor; }
public void charge() { mBarsCount = MAX_ENERGY_BARS; }
public boolean isBatteryEmpty() { return mBarsCount == 0; }
public boolean isFullyCharged() { return mBarsCount == MAX_ENERGY_BARS; }
}
1 Answer
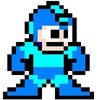
Robert Richey
Courses Plus Student 16,352 PointsHi Ashford,
Let's break down the instructions and figure out what we need to do.
let's use our new isFullyCharged helper method to change our implementation details of the charge method
This tells us we'll be using isFullyCharged
insdie the charge
method. Here's the charge method given to us:
public void charge() {
mBarsCount = MAX_ENERGY_BARS;
}
Currently, charge
will set mBarsCount equal to 8. However, the challenge is asking us to change this method so "it will only charge until the battery reports being fully charged."
We're also told to "use the !
symbol..." (which toggles boolean values) "...and a while
loop. Inside the loop increment mBarsCount
."
Let's start by adding a while loop to the charge method.
public void charge() {
while () {
mBarsCount = MAX_ENERGY_BARS;
}
}
Recall that isFullyCharged
will return a boolean value. It returns true
if mBarsCount
equals 8 or false otherwise. We can use this as the while's conditional.
public void charge() {
while (isFullyCharged()) {
mBarsCount = MAX_ENERGY_BARS;
}
}
This still doesn't seem quite right. We want this loop to run while mBarsCount
is not fully charged. That's where the !
symbol comes in.
public void charge() {
while (!isFullyCharged()) {
mBarsCount = MAX_ENERGY_BARS;
}
}
OK, now the loop conditional is setup correctly, but we still have one more problem to solve. Inside the loop, instead of setting it to MAX_ENERGY_BARS
for each loop iteration, we need to increment mBarsCount
by 1 for each loop iteration.
I'll let you try and figure that part out. If you're still having trouble, please leave a comment and I'll explain how to increment a variable.
Cheers