Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial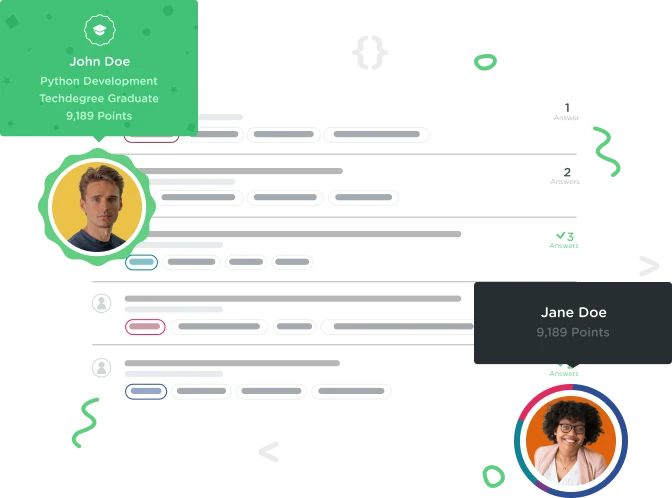

Abe Daniels
Courses Plus Student 2,781 PointsI am getting a bummer that doesn't make sense. "Did you add a new constructor that takes accepts a string?"
How do I go about adding this constructor? Constructors always seem repetitive and unnecessary to me, can someone help me find the use / purpose to them?
////In the Forum class, add a constructor that takes a String for the topic and sets the private field mTopic.
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
// TODO: We need to expose the description
}
public class User {
public User(String firstName, String lastName) {
// TODO: Set the private fields here
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
//what i put
public String topic() {
mTopic = "fruit";
return mTopic;
}
public void addPost(ForumPost post) {
/* When all is ready uncomment this...
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
*/
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
// Forum forum = new Forum("Java");
// Take the first two elements passed args
// User author = new User();
// Add the author, title and description
// ForumPost post = new ForumPost();
// forum.addPost(post);
}
}
1 Answer

mrbrutus
8,047 PointsI was really struggling with this one too. Thanks to Aaron up there, I was able to figure it out. Here is what it should look like:
public Forum (String topic) {
mTopic = topic;
}
Aaron Arkie
5,345 PointsAaron Arkie
5,345 PointsHello Abe! A constructors format is the following.