Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial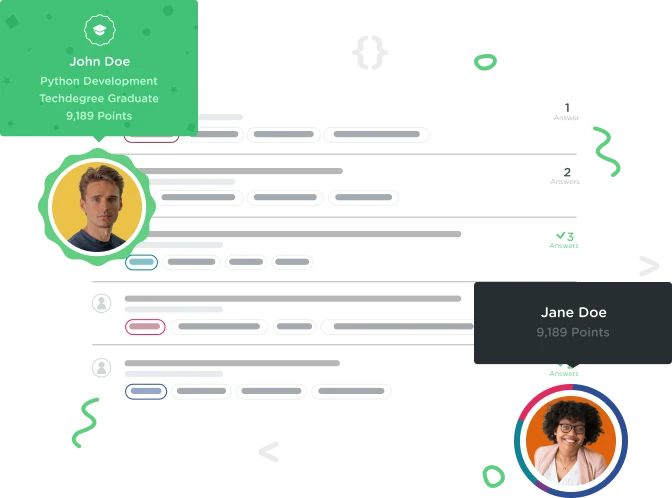

Yassin Sassi
3,571 PointsI am stuck for longer than I like to admit on the getTileCount() function
I don't even know where to start. Could someone just tell me what is wrong?
One of the biggest issues right now is, that I don't know how to use the tile variable in the for-each loop, which I already declared in the function arguments?
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public int getTileCount(char tile){
int tileCount;
char[] mHandArray = mHand.toCharArray();
for( tile : mHandArray ){
tileCount += 1;
}
return tileCount;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
}
1 Answer
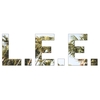
Lee Geertsen
Java Web Development Techdegree Student 16,749 PointsSo you have to make a function that takes a char/letter as input and that returns an integer equal to the number of times that the player has the given char in his hand. So you start by creating a count variable and setting it to 0. Then u use the 'for each' loop. So to be able to look for letters in the String mHand we first convert it to an array of chars using the '.toCharArray'. Then the 'for each' loop will run for each char in the array. In the loop you have to put an if statement to check if char that is giving in the function is equal to the char in the array. If it's the same letter then you increment the counter by 1. Finally you just return the count variable.
public int getTileCount(char letter) {
int count = 0;
for (char tile : mHand.toCharArray()) {
if (letter == tile) {
count += 1;
}
}
return count;
}