Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial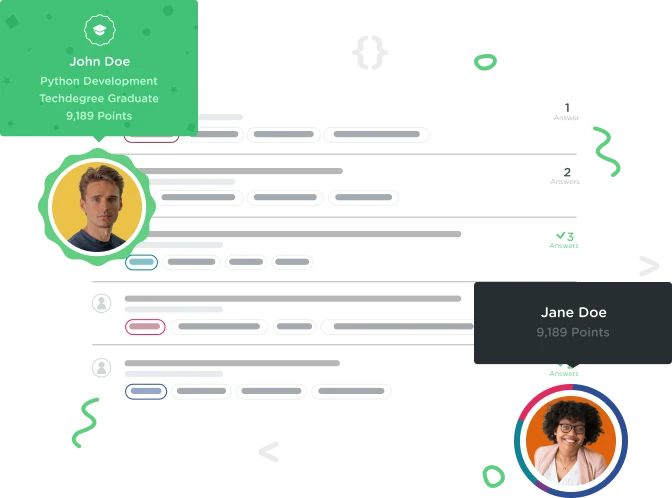

Preston Hill
5,766 PointsI apparently just don't understand.
This final code challenge will require you to use almost everything we've covered in this course. You’ll write a program to celebrate completing this course by printing “Yay!” to the screen a given number of times. Write a program that: Prompts the user to enter the number of times to enter “Yay!”. I've done this for you. Leave this as it is. Prints “Yay!” to the screen that number of times (Hint: You’ll need a loop for this. You’ll also need to keep track of how many times the loop has run). You can print them all on the same line or on separate lines.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
int times = Console.Write("Enter the number of times to print \"Yay!\": ");
} if (counter < times)
{
Console.Write("Yay!");
int counter = 1;
}
}
}
3 Answers
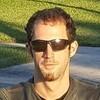
Joshua Kaufman
19,193 PointsThe first part can work like this
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
var response = Console.ReadLine();
int count = Int32.Parse(response);
for(int i = 0; i < count; i++)
{
Console.WriteLine("Yay");
}
}
}
}
I did 4 things here:
- Read the response in a var type variable, since ReadLine reads things as strings.
- Used another variable of type int to save the result of the Int32.Parse() function. I did this because converting the response from string to int is needed to run counting loops.
- Processed the response into a for loop. Inside of the first condition, I declared a basic int variable "i" and set it equal to 0 so that the count goes up by one until the count variable is reached. Just remember that 0 is the first time it runs. So if the user says 5. It will run at i = 0, 1, 2, 3, 4 (5 numbers total).
- Used Console.WriteLine to process the response that the prompt asked for. Technically, the assignment will let you also use Console.Write, but Console.WriteLine is a little bit neater in this case, so I used that.
It is also worth mentioning that you could have encapsulated the Console.WriteLine("Yay"); code using a while loop. If you want, I can show you how that works too.
Let me know if you want help with the next step (Hint: using an "if" conditional is needed, and possible a do-while loop).
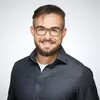
Raffael Dettling
32,999 PointsYou forgot to read the input with ReadLine and you need to convert the input type because you want an int type and the defaul type from Readline is string.
Convert code:
Convert.ToInt32(Console.ReadLine());
For the loop you can use a while or a for loop but you wanna decrease the counter number by 1 each time the loop runs. An if statment checks only ones if the statment is true and then jumps out in the next line of code.
Hope this helped you :)

Preston Hill
5,766 PointsYes, Thank you very much.
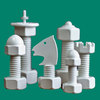
Steven Parker
231,096 PointsHere's a few hints:
-
Console.Write
doesn't return anything - you will need another function to get the user input (such as
Console.ReadLine
) - you will need to convert the input from a string into a number
- you will need to initialize your counter before you begin printing
- you will need some kind of loop (such as "while" or "for", instead of "if") to print multiple times
- inside the loop you will need to increment the counter
I'll bet you can get it now without a spoiler.

Preston Hill
5,766 PointsThank you very much. This was very helpful.
Preston Hill
5,766 PointsPreston Hill
5,766 PointsThank you, that makes perfect sense. For some reason it feels like the answers are on the tip of my tongue but wont come to me naturally.