Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial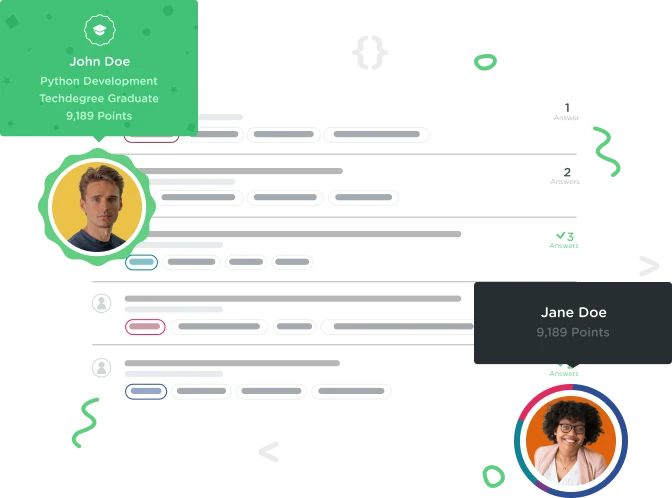

Luke Anderson
1,530 PointsI can't figure out how to make my function return the right String.
When I run the code in xCode I get, "iOSDev by Apple. Filed under Tag(name: "Swift")" instead of what it should be, "iOSDev by Apple. Filed under Swift" My issue is that I can't figure out how to get the function to print "name", from within the struct Tag. without specifying the struct Tag within the var "tag". Help would be very appreciated!
Best, Luke.
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
func description() ->String {
return "\(title) by \(author). Filed under \(tag)"
}
}
let firstPost = Post(title: "iOSDev",author: "Apple", tag: Tag(name:"Swift"))
let postDescription = firstPost.description()
4 Answers

Ryan Huber
13,021 PointsIn your description method you are currently passing in the tag property. You need to pass in the name property of the tag. For instance:
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let firstPost = Post(title: "iOSDev",author: "Apple", tag: Tag(name:"Swift"))
let postDescription = firstPost.description()

Gabriel Kieruzel
Courses Plus Student 19,655 PointsAlso you can print out:
struct Tag { let name: String }
struct Post { let title: String let author: String let tag: Tag
func description() ->String {
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let firstPost = Post(title: "iOSDev",author: "Apple", tag: Tag(name:"Swift")) let postDescription = firstPost.description()
print("(firstPost.description())") // <-- will print: "iOSDev by Apple. Filed under Swift"

Gabriel Kieruzel
Courses Plus Student 19,655 PointsSorry I forgot about formatting in my previous answer:
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
func description() ->String {
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let firstPost = Post(title: "iOSDev",author: "Apple", tag: Tag(name:"Swift"))
let postDescription = firstPost.description()
print("\(firstPost.description())") //<--- will print: "iOSDev by Apple. Filed under Swift"

Luke Anderson
1,530 PointsI tried this and it didn't work, I even copy pasted the whole code and it won't go through. Any ideas why? the error message is that I need to "make sure you're declaring an instance method named description that returns a string.", which I am.

Gabriel Kieruzel
Courses Plus Student 19,655 PointsReally strange, anyway please ( just for testing purposes ) create new app project in Xcode:
File --> New Project --> OS X --> Application --> Command Line Tool
Then in created 'main.swift' copy and paste the code above straight into the file ( below created print("Hello, World!") statement)
Compile and run. In console pane in Xcode you should be able to see printed outputs .
Luke Anderson
1,530 PointsLuke Anderson
1,530 PointsThanks a lot.
Luke Anderson
1,530 PointsLuke Anderson
1,530 PointsNever mind haha it won't work, I tried this and it wouldn't say its correct, I even copy pasted the whole code and it won't go through. Any ideas why? the error message is that I need to "make sure you're declaring an instance method named description that returns a string.", which I am.
Ryan Huber
13,021 PointsRyan Huber
13,021 PointsIn my first answer I left out the majority of the code for brevity. I updated the answer with the full code for the example. When I paste this code in the answer is correct. Let me know if you have issues with it.
Luke Anderson
1,530 PointsLuke Anderson
1,530 PointsI just tried the updated code and it still won't work, I'm afraid the issue may be with the treehouse website and not with your code.
Ryan Huber
13,021 PointsRyan Huber
13,021 PointsSorry. You need a space between the -> and
String
. Give that a try. That definitely worked for me. I just copy and pasted it from here into the challenge.Luke Anderson
1,530 PointsLuke Anderson
1,530 PointsThank you so much that got it to work.