Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial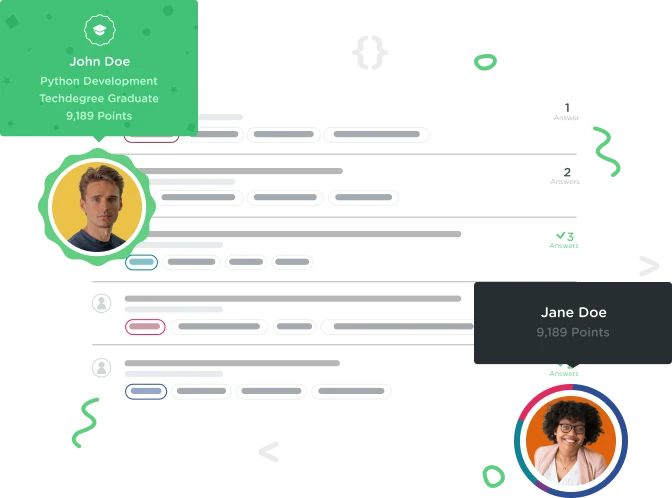
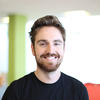
Kristian Woods
23,414 PointsI can't insert records into my localhost database
Hey, I'm trying to create a simple sign up form that logs users info onto my localhost database. However, for some reason, it only inserts the password into the database. Not the username or email..
<?php
include("inc/query.php"); <!-- DATABASE QUERY -->
?>
<!DOCTYPE html>
<html>
<head>
<title>Sign Up</title>
<link rel="stylesheet" type="text/css" href="main.css">
</head>
<body>
<div class="inner-form-bg">
<form action="welcome.php" method="post">
<h1 class="form-h1 sign-up-h1">Sign up!</h1>
<input type="text" placeholder="Username:" name="username" class="field-input req" required><br>
<input type="email" placeholder="Email:" name="email" class="field-input req" required><br>
<input type="password" placeholder="Password:" name="password" class="field-input req" required><br>
<button type="submit" class="submit field-input login-submit">Submit</button><br>
<button type="button" class="field-input new-acc-btn login-btn"><a href="index.php" class="create-acc back-to-login">Back to Login</a></button>
</form>
</div>
</body>
<footer>
</footer>
</html>
My database connection:
<?php
$dbhost = "localhost";
$dbuser = "root";
$dbpass = "root";
$dbname = "member_form";
$connection = mysqli_connect($dbhost, $dbuser, $dbpass, $dbname);
//Test database connection
if(mysqli_connect_errno()) {
die("Database connection failed: " .
mysqli_connect_error() . " (" .
mysqli_connect_errno() . ")"
);
}
?>
My query:
<?php
//check for form submission
if($_SERVER['REQUEST_METHOD'] == 'POST') {
$errors = array(); // start array
//check for username
if(empty($_POST['username'])) {
$errors[] = "You forgot to enter your username";
} else {
$username = mysqli_real_escape_string($connection, trim($POST_['username']));
}
//check for email
if(empty($_POST['email'])) {
$errors[] = "You forgot to enter your email";
} else {
$email = mysqli_real_escape_string($connection, trim($POST_['email']));
}
//check for password
if(empty($_POST['password'])) {
$errors[] = "You forgot to enter your password";
} else {
$password = mysqli_real_escape_string($connection, trim($POST_['password']));
}
if(empty($errors)) {
include("inc/dbconnection.php");
$sql_signUp = "INSERT INTO login (username, email, passcode) VALUES('$username', '$email', SHA1('$password'))";
$result = mysqli_query($connection, $sql_signUp);
if($result && mysqli_affected_rows($connection) == 1) {
//message success
echo "success! 1 row affected";
} else {
die("Database query failed! No rows affected...");
}
}
}
?>
1 Answer

Umesh Ravji
42,386 PointsYou are using $POST_ inside your trim methods, is this not throwing an error? I suspect the password is simply hashing an empty string or something.
Kristian Woods
23,414 PointsKristian Woods
23,414 Pointswhy would that be, though? the textbook I taken it from used that exact line of code
thanks
Kristian Woods
23,414 PointsKristian Woods
23,414 PointsI echoed back the sql statement, and it returned
INSERT INTO login (username, email, passcode) VALUES('', '', SHA1(''))