Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial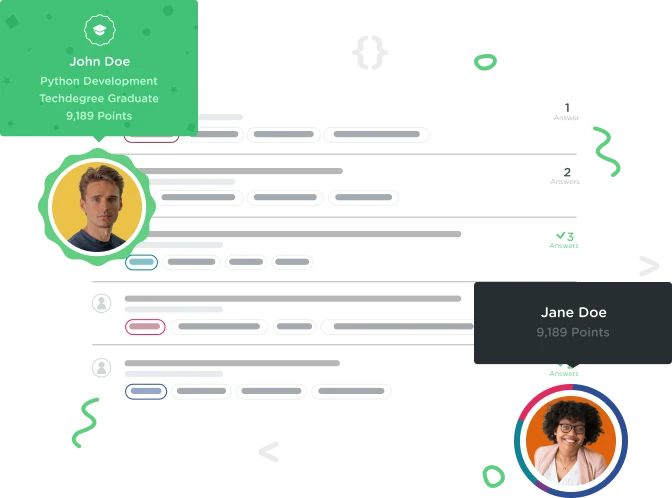

Subham Roy
270 PointsI can't resolve this task
can u give me the answer of this task?
struct Post{
var title = "iOS Development"
var author = "Apple"
var tag = "Swift"
func description() {
print("(title) by \(author). Filed under \(tag)")
}
}
let firstPost = Post()
let postDescription = firstPost.description()
1 Answer
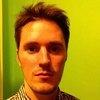
Brendan Whiting
Front End Web Development Techdegree Graduate 84,736 PointsFirst of all, they defined a struct called Tag for you - it's gone from your code, but you still need it.
Then they want you to define another struct called Post. Remember that defining the struct is just creating the abstract scaffold of the thing - you aren't actually creating a real life instance yet, so you're not going to give any of the properties values yet. The syntax for your Post struct should be very similar to the Tag struct. (And one of the properties in the Post struct is actually of type Tag - this is why you still need that Tag code - otherwise it won't know there's such thing as a Tag).
Then they want you to create an instance of the Post struct called firstPost. You're using its built in initializer method, Post(), which will take all of the real values of the properties as arguments. The really tricky part is that one of your properties is a Tag, which itself needs to be initialized with a value.
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
}
let firstPost = Post(title: "iOS Development", author: "Apple", tag: Tag(name:"Swift"))
In the code above I initialized firstPost and its Tag property all in one line, but you could also break it up into bits like this:
let firstTag = Tag(name: "Swift")
let firstPost = Post(title: "iOS Development", author: "Apple", tag: firstTag)