Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial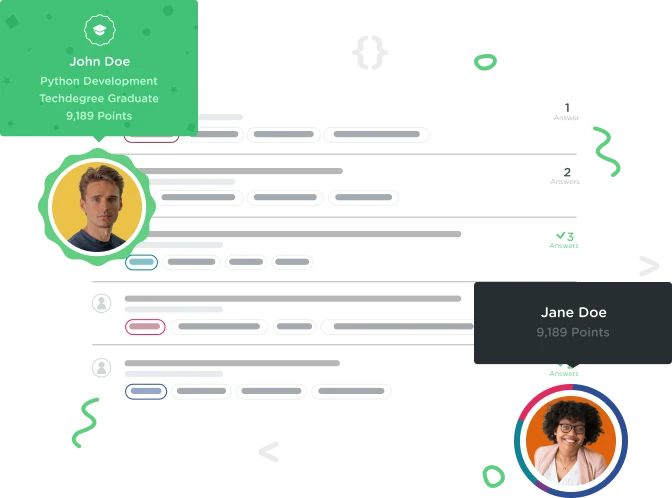

Connor Whyte
1,395 PointsI completed the address book task provided in the wrap up of C# basics. Is there anything I could have done better?
using System; using System.Linq; using System.Collections.Generic; namespace Address_Book { class Program { static void Main() {
var mainbook = new Entry[]
{
new Entry("Jonathan", "Banks", "03424234234", "a", "nohalfm23@gmail.com"),
new Entry("Will", "Arnett", "334847838322", "f", "wedemandtobetakenseriously@hotmail.co.uk"),
new Entry("Andy", "Bernard", "984783837838", "f", "AndyDeservedBetter@hotmail.co.uk"),
new Entry("Dylan", "Maxwell", "028372615273", "a", "innocentman@gmail.com"),
new Entry("Bryan", "Cranston", "382939000272", "f", "bettercallhal@gmail.com"),
new Entry("Ed", "Helms", "984783837838", "f", "AndyDeservedBetter@hotmail.co.uk"),
new Entry("Jimmy", "Mcgill", "392582386549", "a", "its_all_good@gmail.com"),
new Entry("Andy", "Dwyer", "395238384595", "cccccc", "BertMaclinFBI911@hotmail.co.uk"),
new Entry("Bojack", "Horseman", "398234562395", "f", "sadhorse2@hotmail.co.uk"),
new Entry("Butterscotch", "Horseman", "444444444444", "cccccc", "FreeChurro@hotmail.co.uk")
};
List<Entry> addressBook = new List<Entry>();
addressBook.AddRange(mainbook);
while (true)
{
//Get command from user then split it up into an array.
string entry = Console.ReadLine();
string[] splitentry = entry.Split(new char[] { ' ' });
//See what the command starts with
if (entry.ToLower().StartsWith("search"))
{
SearchEntry(addressBook, splitentry);
}
else if (entry.ToLower().StartsWith("add"))
{
AddEntry(addressBook, splitentry);
}
//If user types "quit" then the program stops.
else if (entry.ToLower().Trim() == "quit")
{
break;
}
else if (entry.ToLower().Trim() == "help")
{
Console.WriteLine("Type 'search' to search for an entry.\nType 'add' to add an entry (EXAMPLE: add [firstName] [surname] [phoneNumber] [address] [email])\nType 'view' to view the address book.\nType 'help' for a list of commands.\nType 'quit' to exit the program.\n");
}
else if (entry.ToLower().Trim() == "view")
{
View(addressBook);
}
}
}
static public void View(List<Entry> addressBook)
{
//Prints out each entry seperately as long as they are both valid and visible.
for (int i = 0; i < addressBook.Count; i = i + 1)
{
if (addressBook[i].valid && addressBook[i].visible)
{
Console.WriteLine(addressBook[i].details[0] + " " + addressBook[i].details[1] + "\t\t " + addressBook[i].details[2] + "\t " + addressBook[i].details[3] + "\t " + addressBook[i].details[4]);
}
else if (!addressBook[i].valid && addressBook[i].visible)
{
Console.WriteLine(addressBook[i].details[0] + " is not a valid entry. Please check over this entry.");
}
}
Console.WriteLine(" ");
}
static public void AddEntry(List<Entry> addressBook, string[] entry)
{
//Trys incase entry array is not big enough.
try
{
addressBook.Add(new Entry(entry[1], entry[2], entry[3], entry[4], entry[5]));
Console.WriteLine("New Entry Added: " + entry[1] + " " + entry[2] + " " + entry[3] + " " + entry[4] + " " + entry[5]);
}
catch { Console.WriteLine("New entry could not be added."); }
}
static public void SearchEntry(List<Entry> addressBook, string[] entry)
{
//Checks to see if the second word in the command equals one of the variable entries' strings.
string[] entries = { "firstname", "surname", "phone", "address", "email" };
if (entries.Contains(entry[1].ToLower()))
{
//Gives the int index the index of the second word in the command corresponding to the entries array
int index = Array.IndexOf(entries, entry[1].ToLower().Trim());
//Checks if each entry in the address book is equal to the third word in the command. If not, it will not show up.
foreach (Entry f in addressBook)
{
if (!(f.details[index] == entry[2]))
{
f.visible = false;
}
}
View(addressBook);
//Sets all the entries back to being visible
foreach (Entry f in addressBook)
{
f.visible = true;
}
}
else Console.WriteLine("Unrecognized type: " + entry[1]+"\n");
}
}
class Entry
{
public bool valid = true, visible=true;
public string[] details = new string[5];
public Entry(string FirstName, string LastName, string PhoneNumber, string Address, string Email)
{
try
{
details[0] = FirstName;
details[1] = LastName;
details[2] = PhoneNumber;
details[3] = Address;
details[4] = Email;
} catch
{
valid = false;
}
}
}
}
Apologies for the lack of colour and apologies if this is not where I should be asking this. If I should post this somewhere else please tell me if theres a better place for this.
1 Answer
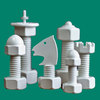
Steven Parker
241,970 PointsTo get proper formatting and syntax coloring, put three accents ("back-ticks") on the line before the code starts, followed by the letters "cs" to indicate the language. End the code block with another line with three accents on it.
I'd say you've done a great job on this task and have demonstrated a thorough understanding of the fundamentals. Cheers!
Keep up the good work, and happy coding!