Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial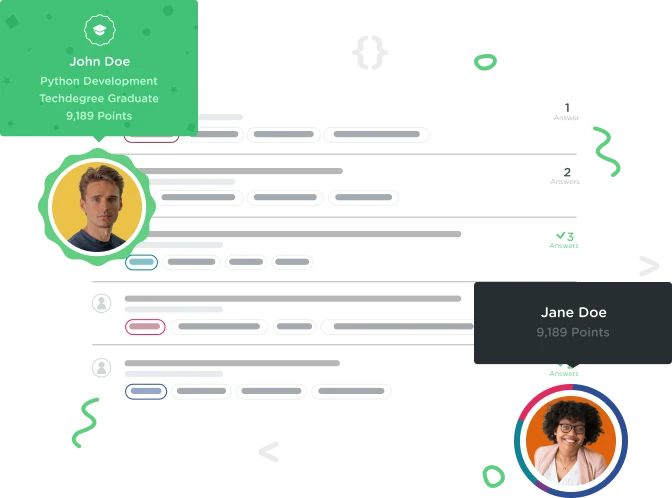

Unsubscribed User
Courses Plus Student 1,108 PointsI do not get this at all:(
function max(number1, number2) {
var number1;
var number2;
if (number1 > number2) {
return true;
else {
return false;
}
}
max(15, 10);
3 Answers
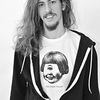
eck
43,038 PointsSo there are a few problems.
First, you should not declare number1 and number2 as variables* in your function when you already have them as parameters (When you name them in the brackets that means they are parameters) The parameters are given values when you run the function and pass values into the brackets. In your case, when you write max(15, 10), number1 is 15 and number2 is 10. *This is not causing a problem, but is just unneeded code.
Second, problem will actually cause an error. You are missing a closing curly brace for your if statement. You have if(){ else{}
but you need if(){} else{}
.
The last problem is that you are asked to return the larger of the two numbers you passed into the function but you are trying to return a Boolean (true or false) What you will want to do is return number1 if it is greater, or else just return number2.
Below is an example that should pass the first part of the code challenge, good luck!
function max(num1, num2){
if(num1 > num2){
return num1;
} else {
return num2;
}
}

Jacob Mishkin
23,118 PointsLet's break this down through the two questions
question 1. Create a new function named max() which accepts two numbers as arguments. The function should return the larger of the two numbers. You'll need to use a conditional statement to test the 2 numbers to see which is the larger of the two.
The first question is ask you to do four things:
- write a function named max();
function max();
- max should accept two arguments, so if there is arguments there must be parameters.
function max(a , b )//your parameters here, when you call the function you will use arguments.
- a conditional statement i.e.. if statement.
function max(a, b) {
if (a > b){
//do something here
}else {
//do something else here
}
}
- return the larger number
return a or b.
Questions 2. Underneath the max() function you just created, call it with two arguments and display the results in an alert dialog. You can pass the result of the function to the alert() method. For example, to display the results of the Math.random() method in an alert dialog you could type this: alert( Math.random( ) );
to call a function just pass in arguments where the parameters were:
alert(max(4. 2));
and there you go. Remember programing is a step by step process. look at the questions and find out each step needed to pass first then complete each step at a time. Doing it all at once can be overwhelming sometimes.
I hope this helps.

Unsubscribed User
Courses Plus Student 1,108 PointsThank you, an aha moment :) I should just trust myself more, I did this first .
eck
43,038 Pointseck
43,038 PointsI edited you post to display you JS code correctly. If you go to edit the post or view the Markdown Cheatsheet you can see how to do that yourself :)