Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial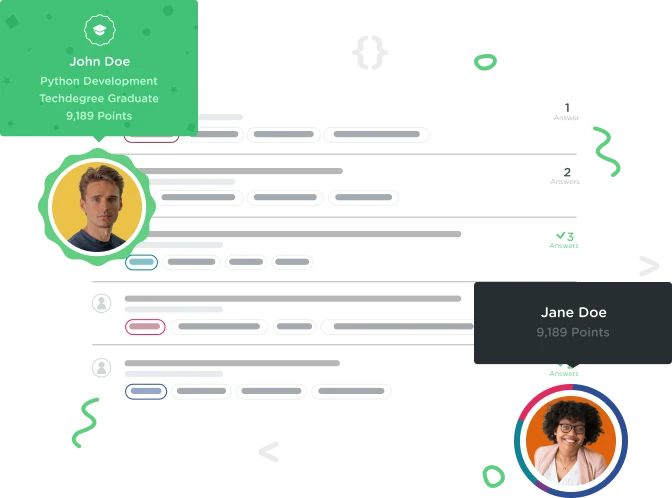

chaya aizenman
Courses Plus Student 405 Pointsi dont know how tp do this exercise. please advise
what am i doing wrong here?
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount(char Tile){
int counter = 0;
for (char letter: mHand.toCharArray())
{
if(mHand.indexOf(Tile)>=0)
{
counter = counter +1;
}
}
return counter;
}
}
3 Answers

Craig Dennis
Treehouse TeacherHow about checking if Tile is equal to letter?
Let me know if that hint doesn't do the trick ;)
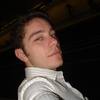
Caio Moretti Marques
Courses Plus Student 27,922 PointsHi Chaya,
You just need to compare the iteration with the argument you're passing to the method. Try to make this:
public int getTileCount(char tile) { int counter = 0;
for (char letter: mHand.toCharArray()) { if(letter == tile) { counter++; } }
return counter;
}
You see: you pass the tile you want to count in the method, and the for each loop will iterate and add 1 to the counter every time that the tile you passed as parameter will appear. Try that.

chaya aizenman
Courses Plus Student 405 PointsThanx
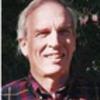
jcorum
71,830 PointsYou have this for the getTileCount() method:
public int getTileCount(char Tile){
int counter = 0;
for (char letter: mHand.toCharArray())
{
if(mHand.indexOf(Tile)>=0)
{
counter = counter +1;
}
}
return counter;
}
It compiles. But a couple of observations. First, you want to be really more careful about indentation. The rule is: indent after an opening curly brace, and outdent after a closing curly brace. It also works better if you use the Tab key rather than spaces.
Second, since mHand is a String, you can iterate over Strings. You don't have to try to turn it into an array first. Think of Strings as already being arrays of chars. That's not quite correct (they are a collection of chars), but close enough, in that you can use subscript notation when you iterate over them, and as they have a length() method rather than a length property.
public int getTileCount(char tile){ //names of formal parameters should start with lowercase letter
int counter = 0;
for (int i = 0; i < mHand.length(); i++) { //loop through the chars in the String
if(mHand.charAt(i) == tile){ //check each tile to see if it matches the one passed in
counter++;
}
}
return counter;
}
But the real issue is why doesn't your code work. What's the logic error. As the editor implies, your code compiles and runs, but gives the wrong result. If mHand is 'x' and 't', and tile is 't' your method returns 2.
You create a local variable letter
in your for-each loop but you never use it. Each time though the loop you are asking if
mHand.indexOf(Tile)>=0
In other words, if Tile is 't', each time through the loop you are asking if mHand contains a 't', and the answer, each time, if mTile does contain a 't', is yes. So if the String has 7 chars, one of which is 't', you would return 7.
chaya aizenman
Courses Plus Student 405 Pointschaya aizenman
Courses Plus Student 405 PointsYou are an amazing teacher! Thanx!