Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial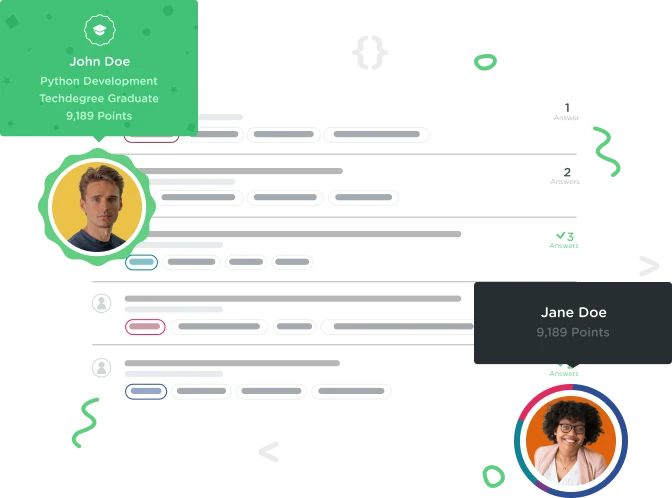
janeporter
Courses Plus Student 23,471 Pointsi don't know what else to do here...as i've never used the User class before...
Can't figure out the last task in this coding challenge. i've never used the User class before so i don't know what to do with it....
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public ForumPost(User author, String title, String description) {
mAuthor = author;
mTitle = title;
mDescription = description;
}
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getDescription() {
return mDescription;
}
// TODO: We need to expose the description
}
public class User {
private String mFirstName;
private String mLastName;
public User(String firstName, String lastName) {
// TODO: Set the private fields here
mFirstName = firstName;
mLastName = lastName;
}
public String getFirstName() {
return mFirstName;
}
public String getLastName() {
return mLastName;
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public Forum(String topic) {
mTopic = topic;
}
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
// Take the first two elements passed args
User author = new User("Jane","Porter");
// Add the author, title and description
ForumPost post = new ForumPost(User(), "java", "I am done");
forum.addPost(post);
}
}
12 Answers

rtholen
Courses Plus Student 11,859 PointsWell, we're both going to get an A for effort. :)
Your code looks correct so I just worked through the entire exercise myself and got it to pass with the following:
Try replacing
User author = new User("Jane","Porter");
with this:
User author = new User(args[0], args[1]);
which is what it suggests in the original exercise comment (somewhat cryptically):
// Take the first two elements passed args
// User author = new User();
Let's see what happens.
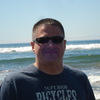
Mark Ihrig
19,966 PointsHello,
The exact same topic about the challenge was discussed on this thread. https://teamtreehouse.com/community/a-bug-i-have-added-a-constructor-and-it-still-asks-if-i-have-added-it
You are building the constructor within the Forum.java class.
In Forum.java you should have a string that looks like this
public Forum(String topic) {
mTopic = topic;
}
I hope this helps!

rtholen
Courses Plus Student 11,859 PointsYou're very close. The ForumPost in Example.java needs an author of type User, a title of type String, and a description of type String. You already created the author using your first and last name, so use that as the first parameter to ForumPost instead of User().
janeporter
Courses Plus Student 23,471 Pointshow do i use a User class. that wasn't covered in the course...my output looks like this:
Starting forum example... New post from Jane Porter about java. Author User@7d4991ad topic java post so glad to be done.
from this code:
Forum forum = new Forum("Java"); // Take the first two elements passed args User author = new User("Jane", "Porter"); // Add the author, title and description ForumPost post = new ForumPost( author,"java","so glad to be done"); forum.addPost(post);

rtholen
Courses Plus Student 11,859 PointsSorry to be "that guy", but are you sure your last post was all the code? From what was printed, it looks like some extra code is still lying around and also getting printed. You'll notice the initial output of "Starting forum example... New post from Jane Porter about java." is correct. What follows looks suspiciously like your original code where you passed in User() to the ForumPost() constructor.
You "use" the User class by
- storing a person's name in it
- retrieving various information from it later when needed, as when the "post" asked for the User first name and last name.
How one uses a class is determined by what public member functions are available and what they are documented to do. In the case of the User class, the only thing you can do with it is create it and later get the first and last name back from it by using the member functions getFirstName() and getLastName(). So when you see a new class, look at the member functions to determine how it can be used.
You're very close.
janeporter
Courses Plus Student 23,471 Pointsthe code i'm referring to is :
```Forum forum = new Forum("Java");
User author = new User("Jane", "Porter");
ForumPost post = new ForumPost(author,"java","so glad to be done");
forum.addPost(post);```
when i try to do new ForumPost(author.getFirstName(), "java", "so glad to be done");
i get a type incompatibility error. i don't understand why i would be required to use a type that wasn't covered in the course anyway. it would be so much easier to use a string type...but what do i know...

rtholen
Courses Plus Student 11,859 PointsIf this is your code:
Forum forum = new Forum("Java");
User author = new User("Jane", "Porter");
/*
Note author should passed into ForumPost, not author.getFirstName().
ForumPost just stores information, just like User. Not all of it will necessarily be used.
*/
ForumPost post = new ForumPost(author,"java","so glad to be done");
/*
When main tells the forum to add the post, the forum, in turn, asks the post for its author,
which, in turn, is asked for its first and last name for printing.
*/
forum.addPost(post);
it should print
"New post from Jane Porter about java."
The description "so glad to be done" isn't used or printed according to the code implementation you supplied. Is this what you get?
janeporter
Courses Plus Student 23,471 Pointsnope. I get an "I expected to see the names I passed in through the args. I do not. Hmmm." error. This is the print code in the Forum.java object:
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
System.out.printf(post.getDescription());
}
Is there something missing?
oh and here is the code from Example.java:
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
// Take the first two elements passed args
User author = new User("Jane","Porter");
// Add the author, title and description
ForumPost post = new ForumPost(author,"Java project","So glad to be finished");
forum.addPost(post);
}
}

rtholen
Courses Plus Student 11,859 PointsThis is the code you originally posted:
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
}
This is the code you last posted (reformatted for readability):
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
System.out.printf(post.getDescription());
}
They are not the same! Unless the exercise specifically asked you to add the (new) last print statement "System.out.printf(post.getDescription());" you probably need to take it out. I suspect their grading software isn't accepting this additional information, even though there is nothing wrong with the code (assuming your code that I can't see hasn't changed).
janeporter
Courses Plus Student 23,471 PointsHere is all of the code:
ForumPost.java
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public ForumPost(User author, String title, String description) {
mAuthor = author;
mTitle = title;
mDescription = description;
}
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getDescription() {
return mDescription;
}
// TODO: We need to expose the description
}
User.java
public class User {
private String mFirstName;
private String mLastName;
public User(String firstName, String lastName) {
// TODO: Set the private fields here
mFirstName = firstName;
mLastName = lastName;
}
public String getFirstName() {
return mFirstName;
}
public String getLastName() {
return mLastName;
}
}
Forum.java
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public Forum(String topic) {
mTopic = topic;
}
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n Post: %s\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle(),
post.getDescription());
}
}
Example.java
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
// Take the first two elements passed args
User author = new User("Jane","Porter");
// Add the author, title and description
ForumPost post = new ForumPost( author,"Java code challenge","So glad to be done." );
forum.addPost( post );
}
}
the output looks fine when i preview the results, but for whatever reason i'm still getting that "i expected to see the names i passed in through the args, but i do not. hmmm" error. do they expect it to glow pink or something?
janeporter
Courses Plus Student 23,471 Pointsoh, and when i removed the added code for the description in the printf ( in Forum.java), i received the same error.
janeporter
Courses Plus Student 23,471 Pointsok. that worked. wow. thank you.