Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial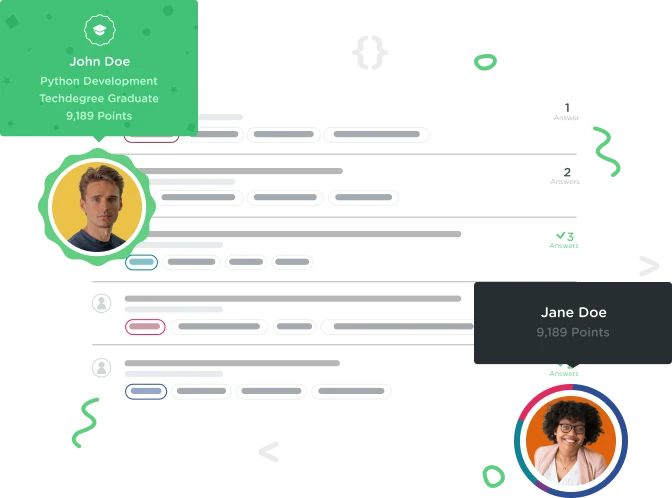

Dominick McCleary
3,012 Pointsi don't know what it is i am doing wrong.
can someone give me a few pointers on what i am doing wrong here please?
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
for(int i = 0; i < frogs.Length; i++)
{
return Frog[i];
}
}
}
}
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int TongueLength { get; }
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
1 Answer
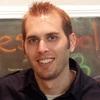
Rick Buffington
8,146 PointsYou've got a couple of things going on here. You are on the right track with your For loop, however you are trying to use the Name of the Class that is Frog instead of using the actual array of Frog objects. In your FrogStats.cs file, you need to add a variable that will add up all of your TongueLengths. Something like:
int total = 0;
Your FOR loop starts off correctly:
for (int i = 0; i < frogs.Length; i++)
{
However, because you are iterating through each item in the frogs
array, you need to use that to pull values and essentially add them into the total.
// Add each TongueLength to the total
total += frogs[i].TongueLength;
}
This will add up each TongueLength into the local variable total
. Once you have done that you close out your for loop and you need to return the average. The formula for that is <total>/<number of items>
so something like:
return total/frogs.Length;
In the end it will look something like:
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
int total = 0;
for(int i = 0; i < frogs.Length; i++)
{
total += frogs[i].TongueLength;
}
return total/frogs.Length;
}
}
}
Hope this helps!
Dominick McCleary
3,012 PointsDominick McCleary
3,012 Pointsthank you so much