Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial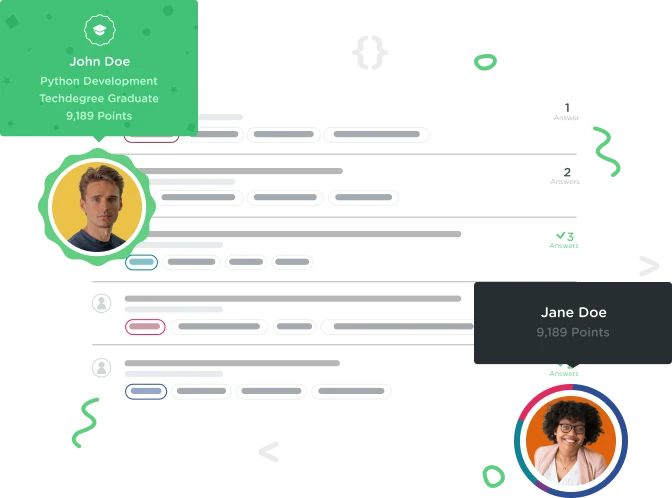

Abe Daniels
Courses Plus Student 2,781 PointsI get the concept, not the logic
So I asked for help on this question 3 times and I seem to be getting the same response. "Make your addItem have a default value of one." I have tried but to no avail can i figure out how to write this without getting 6+ errors.
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
/* Uncomment the line following this comment,
after adding a new method using method signatures,
to solve their request in ShoppingCart.java
*/
// cart.addItem(dispenser);
}
}
public class ShoppingCart {
public void addItem(Product item) {
addItem(item, 1);
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
4 Answers
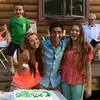
David Axelrod
36,073 PointsAhhh i think i remember. I think there are supposed to be 2 methods, same name but different params.
first one is the standard additem(item, value);
Then because of DRY you reference the first add item method in the second where it just take the product name. then hardcode the would be value arg to 1 and that should do it!

Abe Daniels
Courses Plus Student 2,781 PointsI figured out that it shouldn't be contained inside of that same method. I was adding it inside of the curly braces associated with addItem(Product item){inhere} not addItem(Product item){}outHere
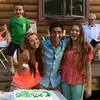
David Axelrod
36,073 Pointsgottcha! glad you figured it out!

Susan O'Shea
2,728 PointsI'm so glad I found this thread because I did this exact same thing, and could not see that I had put it inside the curly braces associated with addItem(Product item). XD

Zubeyr Aciksari
21,074 PointsHi Abe, let me walk you through the solution:
There 3 Java tabs in the challenge.
First, in the Example.java, you need to uncomment "// cart.addItem(dispenser); " part.
Then you need to click on ShoppingCart.java and add this code to there: public void addItem(Product addedItem) { addItem(addedItem, 1); }
I hope this helps!
All the best!

Nicholas Hebb
18,872 PointsYou're halfway there. In Java there are two ways to handle this.
You can have two or more methods (aka, functions) with the same name, as long as the parameters are different. The addItem(Product) method that you have only takes a Product parameter, and inside that method you are calling addItem(Product, Integer) - which currently isn't defined. So, you just need to create that addItem(Product, Integer) method within the ShoppingCart class. Also, make sure that printf is not called in both.
-
You can list a parameter as optional by providing a default value:
public void addItem(Product item, Integer quantity = 1) { System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName()); }
A more in depth explanation can be found here: http://stackoverflow.com/a/12994104/19487