Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial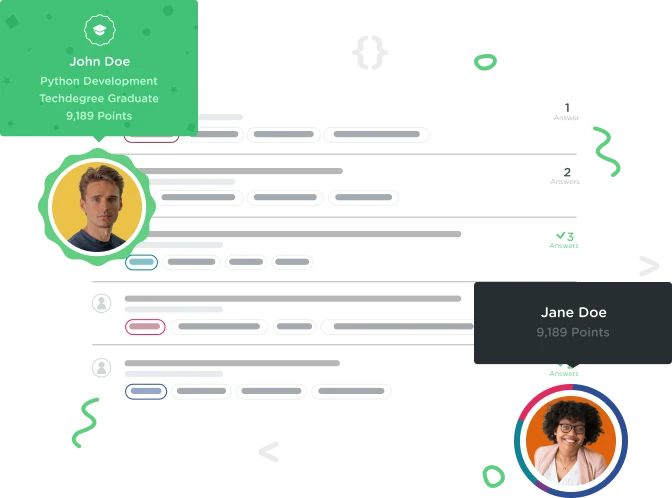
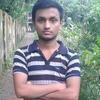
Niyamat Almass
8,176 PointsI have a problem
there is a error in my code.and the error is ./GoKart.java:25: error: cannot find symbol if (newCharge < 0) { ^ symbol: variable newCharge location: class GoKart 1 error
So,please solve the problem
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void load(int laps) {
int newCharage = mBarsCount - laps;
if (newCharge < 0) {
throw new IllegalArgumentException("Not enough battery remains");
}
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
3 Answers

Nikolay Mihaylov
2,810 PointsWell, the solution to the problem is the following:
if((mBarsCount - laps) < 0) throw new IllegalArgumentException();
You should put this in the drive method with the integeter argument.
public void drive(int laps) {
// Other driving code omitted for clarity purposes
if( (mBarsCount - laps) < 0 ) throw new IllegalArgumentException(); //Check the battery count and throw an exception if its below zero
mBarsCount -= laps;
}
You have to create a conditional statement in order to check the battery level. If its below zero you throw an exception using the IllegalArgumentException object. I would like to point out that it isn't required to use the full if else statement. Once you throw an exception the jvm exits the method and ignores all code after the throw statement.
You could also use the isBatteryEmpty method like that:
if( ( isBatteryEmpty() ) throw new IllegalArgumentException();
This is probably the better solution actually :).
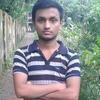
Niyamat Almass
8,176 PointsHi Nikolay Mihaylov I tried your code exactly but there is error .the error is ./GoKart.java:23: error: method drive(int) is already defined in class GoKart public void drive(int laps) { ^ 1 error

Nikolay Mihaylov
2,810 Points----> int newCharage = mBarsCount - laps;
You have declared a variable newCharage not newCharge. That is why it can't find it.
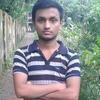
Niyamat Almass
8,176 Pointshey Nikolay Mihaylov after that they said that ''' Bummer! Are you sure you threw the IllegalArgumentException if the requested amount of laps would make the battery less than zero?'''
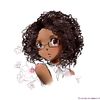
Gloria Dwomoh
13,116 PointsThe challenge asks you to throw the exception in the "drive" method not in the "load" method. So I suggest you start the challenge afresh and go to the drive method then throw and exception if the requested number of laps cannot be completed with the current battery level. In other words if the laps are more than the mBarsCount.
Niyamat Almass
8,176 PointsNiyamat Almass
8,176 Pointswow i solve the problem.thank you Gloria Dwomoh and Nikolay Mihaylov