Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial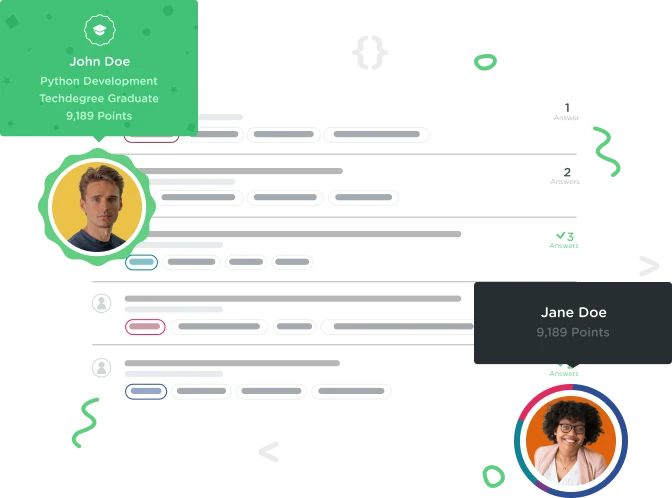

Dwayne Sauls
1,279 PointsI have inserted everything it asked.
Help please
function max(2,3){
if (2 > 3){
}
return max();
);
3 Answers
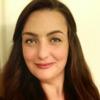
Jennifer Nordell
Treehouse TeacherHi there! I'm going to try giving some hints.
Your function should accept two parameters coming in which are variable names inside this scope. That means that you may not use any name starting with a number as it's not a valid Javascript variable name. Your function must be more generic than you are trying to make it. You are currently attempting to hard code the 2 and 3 as the numbers to be compared. This will not work.
Secondly, you have an if statement set up to compare two numbers. But this statement should be comparing the values of the two variables set up in the parameter list in the declaration of the function. Also, it's missing any statement to say what happens if the first variable is greater than the second. It's here that you'd want to return the value of the first variable.
Third, this challenge will also require an else statement which is missing from your code. This is to say what happens if the first variable is not greater than the second. If it isn't it should return the second variable's value.
Fourth, you are trying to return a call to the function, but this will cause a recursion error. At this point you have a function that's returning a call to itself.
Fifth, you have an extraneous semicolon at the end of the function outside the curly braces.
I hope you can get it with these hints, but please let me know if you're still stuck!

Dwayne Sauls
1,279 PointsThanks. Jennifer Nordell
I have a question. What did u mean by.
"If you're simply returning the value of a variable, the parentheses are not needed."
By what u said. The parentheses is not needed so I didnt have to insert them in there at all and just use them in the conditional statement.
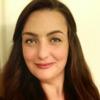
Jennifer Nordell
Treehouse TeacherYou typed this in your code above:
return firstNum();
What you should have typed was:
return firstNum;
The first variant tries to run the firstNum
variable as a function, but it isn't a function. This will actually produce a TypeError and say that the firstNum
is not callable. The parentheses after that firstNum
indicate that this is a function/method to be called/executed.
The second variant returns the value stored in the variable firstNum
.
Here are examples of function calls:
console.log("Hi there!"); //this calls the log method on the console object and passes a string
alert("Dwayne!"); //this invokes the alert function and passes in the string "Dwayne"
max(2, 100); //this runs your max function that you just defined and passes in the numbers 2 and 100
Note the parentheses used to call/invoke/execute a function. A set of empty parentheses simply means that you're calling a function that has no parameters and so takes no arguments. So when you said firstNum();
JavaScript starts trying to run firstNum
as a function, which isn't possible.
Hope this helps!
edited for technical correctness
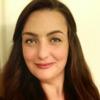
Jennifer Nordell
Treehouse TeacherHi there! No it's not returning a number. But you're much closer than you were! So I'm going to use your own variable names and show you what it's looking for.
function max(firstNum, secondNum){
if(firstNum > secondNum){
return firstNum;
} else {
return secondNum;
}
}
Remember that the parentheses indicate a call to a function/method. If you're simply returning the value of a variable, the parentheses are not needed.
Here we set up a function named max
. It takes two numbers. If the first number is greater than the second number, we return the value of the first number. Otherwise, we return the value of the second number.
Hope this clarifies things!
edited for additional note
Also, console.log is not required by either step of the challenge and could actually cause the challenge to break. Try not to do anything the challenge doesn't explicitly ask for.
Dwayne Sauls
1,279 PointsDwayne Sauls
1,279 PointsHi Thanks for the input. Jennifer Nordell
It says the max function is not returning a number. ///// function max(firstNum,SecNum){ if (firstNum > SecNum){ return firstNum();
console.log('It is greater than the second variable'); } else { console.log('It is not greater than the second variable'); } } console.log(max(4,9)); ///////