Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial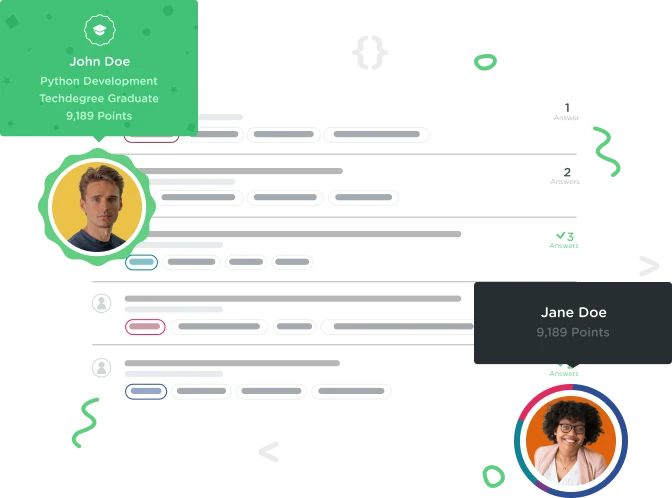
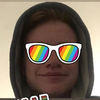
Nils Garland
18,416 PointsI have some PHP login system errors...
So, I have successfully connected to my database and I can use the table 'users' to log in. But when I add a user to the table looking like this:
ID:1 Username: Something password: somespwd
So that look ok right? But it only seems to use the password of the first table. So say I added another user like this:
ID:1 / Username: Something / password: somepswd
ID:2 / Username: Somethingelse / password: otherpswd
Then if I try to log in I can go:
username(input): something
password(input): somepswd
then it works.
Then if I go:
username(input): Somethingelse
password(input): otherpswd
it doesen't work because the I have to use the first password (somepswd) to get logged in.
So that was my main issue, bu then I thought about the fact that I could use WHATEVER username I want right? So I tryed it and the reuslt was that I logged in kind of like this:
username(input):notevenindb
password(input):somepswd
IT WORKS because it has the password of the first password in the table.
here is the code, I hope you take the time to look at this. thank you <3
code:
<?php session_start(); if(isset($_POST['login'])) { include_once("db.php"); $username = strip_tags($_POST['username']); $password = strip_tags($_POST['password']);
$username = stripslashes($username);
$password = stripslashes($password);
$username = mysqli_real_escape_string($conn, $username);
$password = mysqli_real_escape_string($conn, $password);
$password = md5($password);
$sql = "SELECT * FROM `users`";
$query = mysqli_query($conn, $sql);
$row = mysqli_fetch_array($query);
$id = $row['id'];
$db_password = $row['password'];
if($password == $db_password) {
$_SESSION['username'] = $username;
$_SESSION['id'] = $id;
header("location: loggedin.php");
} else {
echo "You didn't enter the correct details!";
}
}
?>
1 Answer

Jaro Schwab
8,957 PointsHey. In your SQL Query, you're fetching everything from the user's table. Then, you assign the id and the password from the first row to the variable. So, these values will always be the first records you have in your db. If you now do a conditional statement where you compare the users input and the db_password value, this doesent really make any sense. -> $db_password will always be: somepswd (first record in db).
So, my suggestion: Change the SQL Query to something like this:
SELECT * FROM `users` WHERE `username` = $username AND `password` = $password LIMIT 1
So, if you now get a Result back, you know that there is a usere with this username and this password. Otherwise, if you won't get a result back, the user with this password doesn't exists..
Note: the mysqli fetch array method doesn't loop over the table. It just returns the first record! if you'd like to get all records back, you need to do this with a loop. Something like this:
while ($row = mysql_fetch_array($result)) {
...
}
Hope this Helps :)
Nils Garland
18,416 PointsNils Garland
18,416 PointsThanks for the answer Jaro, it worked! :)