Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial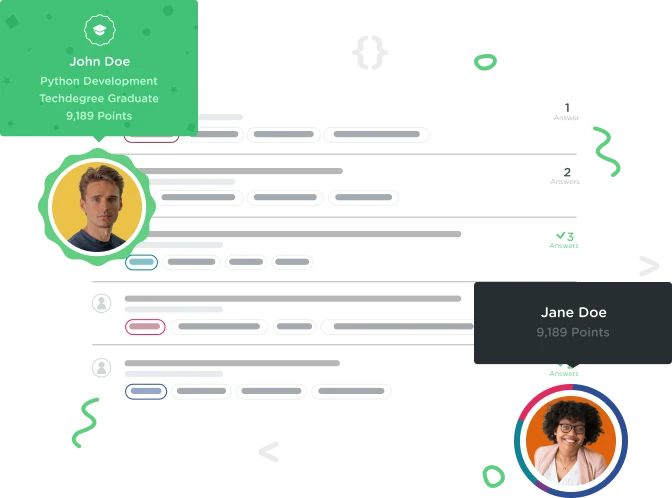
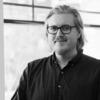
Jonathan Stigsby
8,271 PointsI made this very different... Anyone wanna review? :-)
Well I guess there's a few paths to Rome. Essentially I didn't count a score, I just made conditional statements where if answerOne === questionOne && ... and so on.
/*
1. Store correct answers
- When quiz begins, no answers are correct
*/
const questionOne = "iPaper";
const questionTwo = "30";
const questionThree = "Blonde";
const questionFour = "Yes";
const questionFive = "Cat";
// 2. Store the rank of a player
let playerRank = "";
// 3. Select the <main> HTML element
document.querySelector('main').innerHTML;
/*
4. Ask at least 5 questions
- Store each answer in a variable
- Keep track of the number of correct answers
*/
let answerOne = prompt("Where do I work?");
let answerTwo = prompt("How old am I?");
let answerThree = prompt("What's my haircolor?");
let answerFour = prompt("Am I taller than 180cm?");
let answerFive = prompt("What pet do I have?");
/*
5. Rank player based on number of correct answers
- 5 correct = Gold
- 3-4 correct = Silver
- 1-2 correct = Bronze
- 0 correct = No crown
*/
if (answerOne === questionOne && answerTwo === questionTwo && answerThree === questionThree && answerFour === questionFour && answerFive === questionFive) {
playerRank = "Gold";
} else if (answerOne === questionOne && answerTwo === questionTwo && answerThree === questionThree && answerFour === questionFour) {
playerRank = "Silver";
} else if (answerOne === questionOne && answerTwo === questionTwo && answerThree === questionThree) {
playerRank = "Silver";
} else if (answerOne === questionOne && answerTwo === questionTwo) {
playerRank = "Bronze";
} else if (answerOne == questionOne) {
playerRank = "Bronze";
} else {
playerRank = "No crown";
}
// 6. Output results to the <main> element
document.querySelector('main').innerHTML = playerRank;
1 Answer
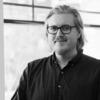
Jonathan Stigsby
8,271 PointsGreat input Sebastian, thanks! I remade it with a score count:
// This is a quiz game
/*
1. Store correct answers
- When quiz begins, no answers are correct
*/
const answerOne = "6";
const answerTwo = "4";
const answerThree = "no";
const answerFour = "yes";
const answerFive = "4";
// 2. Store the rank of a player
let rank = "";
let score = 0;
// 3. Select the <main> HTML element
document.querySelector('main').innerHTML;
/*
4. Ask at least 5 questions
- Store each answer in a variable
- Keep track of the number of correct answers
*/
const questionOne = prompt("What is 1+5?");
if (answerOne === questionOne.toLowerCase()) {
score += 1
}
const questionTwo = prompt("What is 2+2?");
if (answerTwo === questionTwo.toLowerCase()) {
score += 1
}
const questionThree = prompt("Is 2 > 5?");
if (answerThree === questionThree.toLowerCase()) {
score += 1
}
const questionFour = prompt("Is 6 > 2?");
if (answerFour === questionFour.toLowerCase()) {
score += 1
}
const questionFive = prompt("What is 2*2?");
if (answerFive === questionFive.toLowerCase()) {
score += 1
}
/*
5. Rank player based on number of correct answers
- 5 correct = Gold
- 3-4 correct = Silver
- 1-2 correct = Bronze
- 0 correct = No crown
*/
if(score === 5) {
rank = "Gold";
} else if (score >=3) {
rank = "Silver";
} else if (score >=1) {
rank ="Bronze";
} else {
rank = "No crown";
}
// 6. Output results to the <main> element
document.querySelector('main').innerHTML =
`Your score was: ${score}
which means your rank is: ${rank}`;
Sebastian Lofaro
Front End Web Development Techdegree Graduate 29,101 PointsSebastian Lofaro
Front End Web Development Techdegree Graduate 29,101 PointsDoes this always work? If "questionOne" is incorrect, but all the other questions are correct your code would never assign "playerRank" the value "Silver". According to your rules 3-4 correct = Silver. I guess I don't know enough about the coding challenge. I suppose this approach would work if the game is over after the first incorrect answer, in which case you only need to test if(answerFive === questionFive) else if (answerFour === questionFour) etc...
Also, it is important to be cognizant of future growth and reuse of your code. It would be better to have a data structure like an array or an object to store the correct status of each question. Then you can write a function to loop through each of the elements in that data structure. This would scale much better, because the number of questions can change and your testing mechanism is generic enough to immediately work with the new data set.