Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial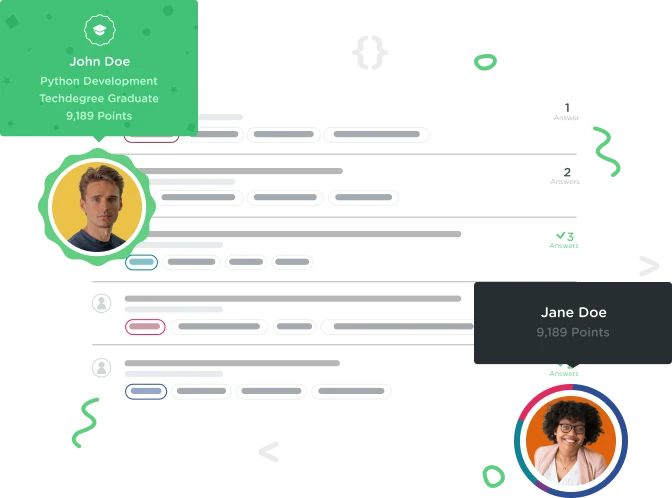

chazber
4,873 PointsI need help with IComparer implementation.
I am lost on this task. I really want to do it, but I'm not entirely sure on the steps that I need to take, logically.
1 Answer
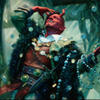
Daniel Medina
13,863 PointsHello chazber,
Congratulations on solving the challenge!
I was also curious about why you'd need a new Student()
too.
The short answer, from what I found, is that you need to use a new Student()
within your Sort()
method so that you are properly implementing the IComparer
interface. If you try to sort without any Student object, you'll get an InvalidOperationException.
Personally, I found this Stack Overflow post as the most helpful, particularly the answer with 207 votes:
https://stackoverflow.com/questions/3309188/how-to-sort-a-listt-by-a-property-in-the-object
I hope this helps!
chazber
4,873 Pointschazber
4,873 PointsOk, so I got it working by doing
My biggest confusion is why I have to instantiate a new student object????? I don't understand how one comes to that conclusion by reading the online documentation. Any advise?