Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial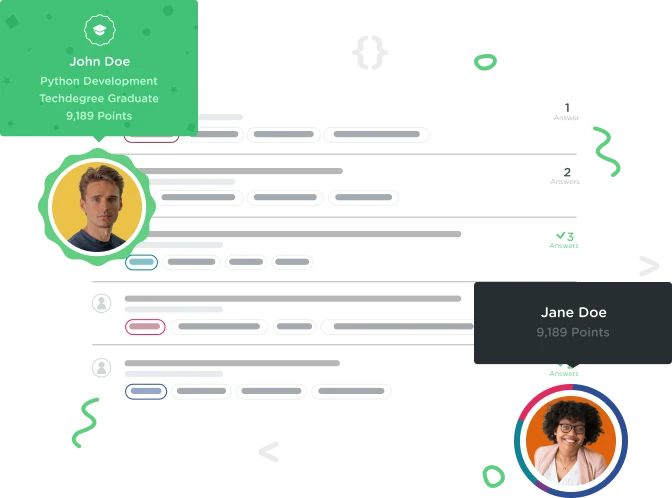
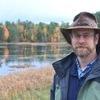
Ralph Findling
5,270 PointsI think I am getting the required output for this exercise, but it's not accepted. Is there a bug in the lesson?
Adding 5 of Cherry PEZ refill (12 pieces) to the cart. Adding 1 Yoda PEZ dispenser to the cart.
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
/* Uncomment the line following this comment,
after adding a new method using method signatures,
to solve their request in ShoppingCart.java
*/
cart.addItem(dispenser);
}
}
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity. Please imagine
lots and lots of code here. Don't repeat it.
*/
}
public void addItem(Product item) {
System.out.printf("Adding 1 %s to the cart.%n", item.getName());
/* Other code omitted for clarity. Please imagine
lots and lots of code here. Don't repeat it.
*/
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
3 Answers
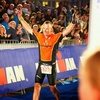
Steve Hunter
57,712 PointsHi Ralph,
Your issue is in the overridden method, addItem
.
There are two now; that's correct. One takes an item
and a quantity
the other takes just an item
and assumes the quantity
is 1. OK with that?
As the first addItem
method states, there's loads of code in there that we don't need to see - that's just for the sake of ease. That code will also need to be in your second method. But that is duplication of code which is a bad thing, right!
So, inside the new method, why not just call the existing one and add one item?
public void addItem(Product item) {
addItem(item, 1);
}
That does what we need it to - and uses the existing method with all its funky hidden code etc.
Does that make sense?
Steve.
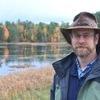
Ralph Findling
5,270 PointsSteve, thank you for the help! Yes, that does result in much nicer code. My solution was not really wrong, but not what they were looking for, right? Thanks for the help.
Ralph
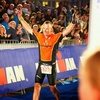
Steve Hunter
57,712 PointsI think the key thing to note here is the direct instruction in the code comments: Please imagine lots and lots of code here. Don't repeat it. As we can understand, the addition of an item to a shopping cart in an online shop requires all sorts of database read/write operations across many database tables. Maintaining these tables, and improving the website and shopping application, will involve modifying the code in these methods. If the two methods here have duplicated code, ignoring the D.R.Y. principles, there's twice the chance of mistakes which could be super-catastrophic for the retailer.
Your solution would have worked, yes, as long as you identified where in all the SQL the quantity of 1 was used and hard-coded that into the method. Given how opaque SQL is, especially when implemented in Java, the chances of getting that wrong are high! I'm not actually sure what the challenge compiler was testing that failed your code - I must assume it is the duplication of the System.out.printf
statement - dunno!
The main thing is you understand why the D.R.Y. principle exists and how small mechanisms like this can be used to make code easier to use, by overriding methods, but stay equally "easy" to maintain, by not duplicating code. Not an easy challenge, to be honest.