Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial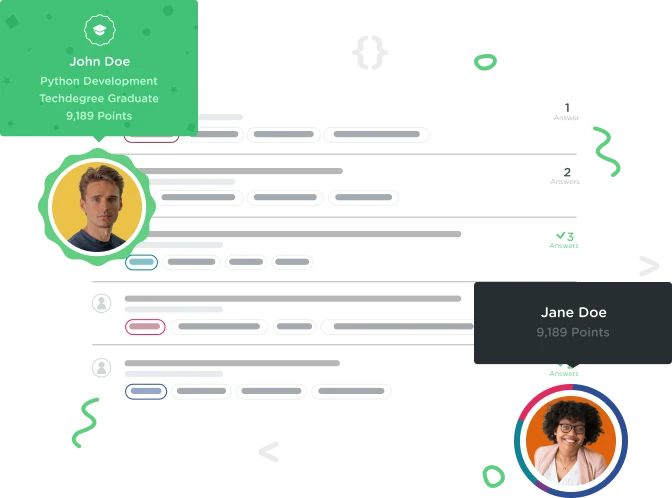
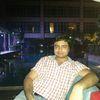
KnowledgeWoods Consulting
5,607 PointsI want to upload video file with progress bar.
Hi, Thanks in advance. I want to upload a video file in php with its progress status.
19 Answers

Philip G
14,600 PointsHi Chetan,
This Code should work for you:
upload_form.html:
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script>
<script>
/* Script written by Adam Khoury @ DevelopPHP.com */
/* Modified by Philip Graf */
/* Video Tutorial: http://www.youtube.com/watch?v=EraNFJiY0Eg */
/* Edit Thread: https://teamtreehouse.com/forum/i-want-to-upload-video-file-with-progress-bar*/
function _(el){
return document.getElementById(el);
}
function uploadFile(){
var file = _("file1").files[0];
if(typeof file === "undefined") {
_("status").innerHTML = "ERROR: Please browse for a file before clicking the upload button";
_("progressBar").value = 0;
return;
}
$.get('file_upload_parser.php?getsize', function(sizelimit) {
if(file.type !== "video/mp4") {
var typewarn = "ERROR: You have to select a MP4-File";
_("status").innerHTML = typewarn;
_("progressBar").value = 0;
return;
}
if(sizelimit < file.size) {
var sizewarn = "ERROR: The File is too big! The maximum file size is ";
sizewarn += sizelimit/(1024*1024);
sizewarn += "MB";
_("status").innerHTML = sizewarn;
_("progressBar").value = 0;
return;
}
var formdata = new FormData();
formdata.append("file1", file);
formdata.append("size", file.size);
var ajax = new XMLHttpRequest();
ajax.upload.addEventListener("progress", progressHandler, false);
ajax.addEventListener("load", completeHandler, false);
ajax.addEventListener("error", errorHandler, false);
ajax.addEventListener("abort", abortHandler, false);
ajax.open("POST", "file_upload_parser.php");
ajax.send(formdata);
});
}
function progressHandler(event){
_("loaded_n_total").innerHTML = "Uploaded "+event.loaded+" bytes of "+event.total;
var percent = (event.loaded / event.total) * 100;
_("progressBar").value = Math.round(percent);
_("status").innerHTML = Math.round(percent)+"% uploaded... please wait";
}
function completeHandler(event){
_("status").innerHTML = event.target.responseText;
_("progressBar").value = 0;
}
function errorHandler(event){
_("status").innerHTML = "Upload Failed";
}
function abortHandler(event){
_("status").innerHTML = "Upload Aborted";
}
</script>
</head>
<body>
<h2>HTML5 File Upload Progress Bar Tutorial</h2>
<form id="upload_form" enctype="multipart/form-data" method="post">
<input type="file" name="file1" id="file1"><br>
<input type="button" value="Upload File" onclick="uploadFile()">
<progress id="progressBar" value="0" max="100" style="width:300px;"></progress>
<h3 id="status"></h3>
<p id="loaded_n_total"></p>
</form>
</body>
</html>
This evening I will send you an further optimized version of the Code.
Best Regards,
Philip

Philip G
14,600 PointsPHP won't do this alone. You need Javascript for that, because PHP is always processing the code first and then giving out the output. Have a look at this good example:
https://www.developphp.com/video/JavaScript/File-Upload-Progress-Bar-Meter-Tutorial-Ajax-PHP
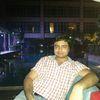
KnowledgeWoods Consulting
5,607 PointsHi, It's working fine. But I want to send hidden value through the same form to the "file_upload_parser.php" file and I am unable to do that. Please help me out.
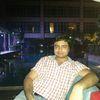
KnowledgeWoods Consulting
5,607 PointsHi, I am able to send the hidden value. Now, the problem is when I upload the big file it shows an error "unknown file1". Please help.

Philip G
14,600 PointsHi Chetan,
First, sorry for the late response, but my internet connection is very bad at the moment. Can you please provide me the code you are using? Sorry if I got my wires crossed, but which "big file" do you mean? Do you mean the video file?
Regards, Philip
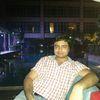
KnowledgeWoods Consulting
5,607 PointsHi Philip, Thanks for the reply. I am using the same code as given in the link above. Yes, I am uploading the video file of 169 Mb. It's not showing that file size is big or something else but it's showing an error "undefined index file1". And also earlier the same code was showing the progress in color but now it's not showing the progress also. it's just directly display the message, that video has been uploaded.
Please Help me out.
Here is my code. // Code for upload_form.php <!DOCTYPE html> <html> <head> <script> /* Script written by Adam Khoury @ DevelopPHP.com / / Video Tutorial: http://www.youtube.com/watch?v=EraNFJiY0Eg */ function _(el){ return document.getElementById(el); } function uploadFile(){ var file = _("file1").files[0]; // alert(file.name+" | "+file.size+" | "+file.type); var formdata = new FormData(); formdata.append("file1", file); var ajax = new XMLHttpRequest(); ajax.upload.addEventListener("progress", progressHandler, false); ajax.addEventListener("load", completeHandler, false); ajax.addEventListener("error", errorHandler, false); ajax.addEventListener("abort", abortHandler, false); ajax.open("POST", "file_upload_parser.php"); ajax.send(formdata); } function progressHandler(event){ _("loaded_n_total").innerHTML = "Uploaded "+event.loaded+" bytes of "+event.total; var percent = (event.loaded / event.total) * 100; _("progressBar").value = Math.round(percent); _("status").innerHTML = Math.round(percent)+"% uploaded... please wait"; } function completeHandler(event){ _("status").innerHTML = event.target.responseText; _("progressBar").value = 0; } function errorHandler(event){ _("status").innerHTML = "Upload Failed"; } function abortHandler(event){ _("status").innerHTML = "Upload Aborted"; } </script> </head> <body> <h2>HTML5 File Upload Progress Bar Tutorial</h2> <form id="upload_form" enctype="multipart/form-data" method="post"> <input type="file" name="file1" id="file1"><br> <input type="button" value="Upload File" onclick="uploadFile()"> <progress id="progressBar" value="0" max="100" style="width:300px;"></progress> <h3 id="status"></h3>
</form> </body> </html>
// code for file_uplaod_parser.php
<?php $fileName = $_FILES["file1"]["name"]; // The file name $fileTmpLoc = $_FILES["file1"]["tmp_name"]; // File in the PHP tmp folder $fileType = $_FILES["file1"]["type"]; // The type of file it is $fileSize = $_FILES["file1"]["size"]; // File size in bytes $fileErrorMsg = $_FILES["file1"]["error"]; // 0 for false... and 1 for true if (!$fileTmpLoc) { // if file not chosen echo "ERROR: Please browse for a file before clicking the upload button."; exit(); } if(move_uploaded_file($fileTmpLoc, "test_uploads/$fileName")){ echo "$fileName upload is complete"; } else { echo "move_uploaded_file function failed"; } ?>

Philip G
14,600 PointsHi Chetan,
First I would change upload_form.php
to upload_form.html
, but that doesn't really matter. Why did you remove
<p id="loaded_n_total"></p>
from the upload form? I think that's the reason of the issue with the progress. The upload of the big file doesn't work because the default PHP POST Limit is 8388608 Bytes = 8 Megabytes. You have to update your post_max_size
and upload_max_filesize
in php.ini to a larger value (Like 200M), then it will work.
Regards,
Philip
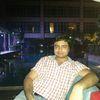
KnowledgeWoods Consulting
5,607 PointsHi, Philip Thanks for replying. Progress Bar is working fine now. This I know, when I want to upload big size file I have to update the php.ini file. My question to you was something else. I don't want to upload such big file to my server. But if somebody tries to upload such bigger file,it should display the message that file is too big and it cannot be uploaded. Instead of displaying the message, it's showing an error "Undefined Index file1". Please Help me out.

Philip G
14,600 PointsHi Chetan,
I have edited the whole Code to fit your needs. This is my result:
upload_form.html
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script>
<script>
/* Script written by Adam Khoury @ DevelopPHP.com */
/* Modified by Philip Graf */
/* Video Tutorial: http://www.youtube.com/watch?v=EraNFJiY0Eg */
/* Edit Thread: https://teamtreehouse.com/forum/i-want-to-upload-video-file-with-progress-bar*/
function _(el){
return document.getElementById(el);
}
function uploadFile(){
var file = _("file1").files[0];
if(typeof file === "undefined") {
_("status").innerHTML = "ERROR: Please browse for a file before clicking the upload button";
_("progressBar").value = 0;
} else {
$.get('file_upload_parser.php?getsize', function(sizelimit) {
if(sizelimit > file.size) {
var formdata = new FormData();
formdata.append("file1", file);
formdata.append("size", file.size);
var ajax = new XMLHttpRequest();
ajax.upload.addEventListener("progress", progressHandler, false);
ajax.addEventListener("load", completeHandler, false);
ajax.addEventListener("error", errorHandler, false);
ajax.addEventListener("abort", abortHandler, false);
ajax.open("POST", "file_upload_parser.php");
ajax.send(formdata);
} else {
var sizewarn = "ERROR: The File is too big! The maximum file size is ";
sizewarn += sizelimit/(1024*1024);
sizewarn += "MB";
_("status").innerHTML = sizewarn;
_("progressBar").value = 0;
}
});
}
}
function progressHandler(event){
_("loaded_n_total").innerHTML = "Uploaded "+event.loaded+" bytes of "+event.total;
var percent = (event.loaded / event.total) * 100;
_("progressBar").value = Math.round(percent);
_("status").innerHTML = Math.round(percent)+"% uploaded... please wait";
}
function completeHandler(event){
_("status").innerHTML = event.target.responseText;
_("progressBar").value = 0;
}
function errorHandler(event){
_("status").innerHTML = "Upload Failed";
}
function abortHandler(event){
_("status").innerHTML = "Upload Aborted";
}
</script>
</head>
<body>
<h2>HTML5 File Upload Progress Bar Tutorial</h2>
<form id="upload_form" enctype="multipart/form-data" method="post">
<input type="file" name="file1" id="file1"><br>
<input type="button" value="Upload File" onclick="uploadFile()">
<progress id="progressBar" value="0" max="100" style="width:300px;"></progress>
<h3 id="status"></h3>
<p id="loaded_n_total"></p>
</form>
</body>
</html>
file_upload_parser.php
<?php
/* Script written by Adam Khoury @ DevelopPHP.com */
/* Modified by Philip Graf */
/* Video Tutorial: http://www.youtube.com/watch?v=EraNFJiY0Eg */
/* Edit Thread: https://teamtreehouse.com/forum/i-want-to-upload-video-file-with-progress-bar*/
$ini_PostSize = preg_replace("/[^0-9,.]/", "", ini_get('post_max_size'))*(1024*1024);
$ini_FileSize = preg_replace("/[^0-9,.]/", "", ini_get('upload_max_filesize'))*(1024*1024);
$maxFileSize = ($ini_PostSize<$ini_FileSize ? $ini_PostSize : $ini_FileSize);
$file = (isset($_FILES["file1"]) ? $_FILES["file1"] : 0);
if(isset($_GET["getsize"])) {
echo $maxFileSize;
exit;
}
if (!$file) { // if file not chosen
if($file["size"]>$maxFileSize){
die("ERROR: The File is too big! The maximum file size is ".$maxFileSize/(1024*1024)."MB");
}
die("ERROR: Please browse for a file before clicking the upload button");
}
if($file["error"]) {
die("ERROR: File couldn't be processed");
}
if(move_uploaded_file($file["tmp_name"], "test_uploads/".$file["name"])){
echo "SUCCESS: The upload of ".$file["name"]." is complete";
} else {
echo "ERROR: Couldn't move the file to the final location";
}
?>
Best Regards,
Philip
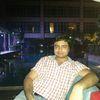
KnowledgeWoods Consulting
5,607 PointsHi Philip, It's still not working. When I am uploading the file of 169 Mb. It's displaying wrong message. It's showing browse the file instead of displaying large file size. And also I want to upload only mp4 format. If user tries to upload some other format like image or any format, it should display the message that only mp4 videos are allowed. Please Help. I need this code.
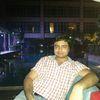
KnowledgeWoods Consulting
5,607 PointsHi Philip, It's now sowing an message that fie size is too big. Now only one problem is left that it should upload only mp4 videos. If any other format user tries to upload, then show the message, that only mp4 is allowed and no other format can be uploaded. Thanks a lot for help.

Philip G
14,600 PointsHi Chetan,
this is the code for the MP4-Filter:
file_upload_parser.php:
<?php
/* Script written by Adam Khoury @ DevelopPHP.com */
/* Modified by Philip Graf @ PhilipGraf.at */
/* Video Tutorial: http://www.youtube.com/watch?v=EraNFJiY0Eg */
/* Edit Thread: https://teamtreehouse.com/forum/i-want-to-upload-video-file-with-progress-bar*/
$ini_PostSize = preg_replace("/[^0-9,.]/", "", ini_get('post_max_size'))*(1024*1024);
$ini_FileSize = preg_replace("/[^0-9,.]/", "", ini_get('upload_max_filesize'))*(1024*1024);
$maxFileSize = ($ini_PostSize<$ini_FileSize ? $ini_PostSize : $ini_FileSize);
$file = (isset($_FILES["file1"]) ? $_FILES["file1"] : 0);
if(isset($_GET["getsize"])) {
echo $maxFileSize;
exit;
}
if (!$file) { // if file not chosen
if($file["size"]>$maxFileSize){
die("ERROR: The File is too big! The maximum file size is ".$maxFileSize/(1024*1024)."MB");
}
die("ERROR: Please browse for a file before clicking the upload button");
}
if($file["error"]) {
die("ERROR: File couldn't be processed");
}
if($file["type"] !== "video/mp4") {
die("ERROR: You have to select a MP4-File");
}
if(move_uploaded_file($file["tmp_name"], "test_uploads/".$file["name"])){
echo "SUCCESS: The upload of ".$file["name"]." is complete";
} else {
echo "ERROR: Couldn't move the file to the final location";
}
?>
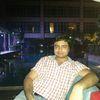
KnowledgeWoods Consulting
5,607 PointsHi Philip, Thanks a lot. The code is working fine. But I want that when it display the message that only mp4 video is uploaded. It should not display the bytes uploaded. It's also displaying the message "Uploaded 11073 bytes of 11073". If file is not uploaded then it should not show the total no. of bytes. I tried editing the code, but was unable to do that. Please help.
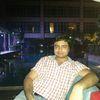
KnowledgeWoods Consulting
5,607 PointsHi, Philip. Thanks a lot for the reply. I will try this and reply to you.
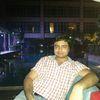
KnowledgeWoods Consulting
5,607 PointsHi Philip, Thanks a lot. Code is working absolutely fine. Thank you so much.

Philip G
14,600 PointsHi Chetan, Glad to help! This is the a little bit optimized Javascript:
upload_form.html
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script>
<script>
/* Script written by Adam Khoury @ DevelopPHP.com */
/* Modified by Philip Graf @ PhilipGraf.at */
/* Video Tutorial: http://www.youtube.com/watch?v=EraNFJiY0Eg */
/* Edit Thread: https://teamtreehouse.com/forum/i-want-to-upload-video-file-with-progress-bar*/
function _(el){
return document.getElementById(el);
}
function uploadFile(){
var file = _("file1").files[0];
if(typeof file === "undefined") {
_("status").innerHTML = "ERROR: Please browse for a file before clicking the upload button";
_("progressBar").value = 0;
return;
}
$.get('file_upload_parser.php?getsize', function(sizelimit) {
if(file.type !== "video/mp4") {
var typewarn = "ERROR: You have to select a MP4-File";
_("status").innerHTML = typewarn;
_("progressBar").value = 0;
return;
}
if(sizelimit < file.size) {
var sizewarn = "ERROR: The File is too big! The maximum file size is ";
sizewarn += sizelimit/(1024*1024);
sizewarn += "MB";
_("status").innerHTML = sizewarn;
_("progressBar").value = 0;
return;
}
var formdata = new FormData();
formdata.append("file1", file);
formdata.append("size", file.size);
$.ajax({
url: 'file_upload_parser.php',
data: formdata,
processData: false,
contentType: false,
type: 'POST',
success: function(data){
_("status").innerHTML = data;
_("progressBar").value = 0;
},
xhr: function() {
var ajax = new window.XMLHttpRequest();
ajax.upload.addEventListener("progress", function (event) {
_("loaded_n_total").innerHTML = "Uploaded "+event.loaded+" bytes of "+event.total;
var percent = (event.loaded / event.total) * 100;
_("progressBar").value = Math.round(percent);
_("status").innerHTML = Math.round(percent)+"% uploaded... please wait";
}, false);
return ajax;
},
error: function (xhr, ajaxOptions, thrownError) {
if(thrownError == "abort") {
_("status").innerHTML = "Upload Aborted!";
return;
}
_("status").innerHTML = "Upload Failed";
}
});
});
}
</script>
</head>
<body>
<h2>HTML5 File Upload Progress Bar Tutorial</h2>
<form id="upload_form" enctype="multipart/form-data" method="post">
<input type="file" name="file1" id="file1"><br>
<input type="button" value="Upload File" onclick="uploadFile()">
<progress id="progressBar" value="0" max="100" style="width:300px;"></progress>
<h3 id="status"></h3>
<p id="loaded_n_total"></p>
</form>
</body>
</html>
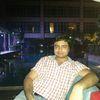
KnowledgeWoods Consulting
5,607 PointsHi Philip, Thanks for the code. When i run this code individually, it's running absolutely fine. But when i merge this code with my code I am getting an error when I am uploading the big file. It shows the progress bar, and then displays the message browse the file insted of displaying file size is big. I just added id value to the java script code and nothing else. Below is my code. uplaod_form. html - replace with manage-lesson-topic.php
// here is the code <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script> <script> /* Script written by Adam Khoury @ DevelopPHP.com / / Modified by Philip Graf @ PhilipGraf.at / / Video Tutorial: http://www.youtube.com/watch?v=EraNFJiY0Eg / / Edit Thread: https://teamtreehouse.com/forum/i-want-to-upload-video-file-with-progress-bar*/ function _(el){ return document.getElementById(el); } function uploadFile(){ var value = $("#topic_id").val(); var file = _("file1").files[0]; if(typeof file === "undefined") { _("status").innerHTML = "ERROR: Please browse for a file before clicking the upload button"; _("progressBar").value = 0; return; } $.get('file_upload_parser.php?getsize', function(sizelimit) { if(file.type !== "video/mp4") { var typewarn = "ERROR: You have to select a MP4-File"; _("status").innerHTML = typewarn; _("progressBar").value = 0; return; } if(sizelimit < file.size) { var sizewarn = "ERROR: The File is too big! The maximum file size is "; sizewarn += sizelimit/(1024*1024); sizewarn += "MB"; _("status").innerHTML = sizewarn; _("progressBar").value = 0; return; } var formdata = new FormData(); formdata.append("file1", file); formdata.append("size", file.size); $.ajax({ url: 'file_upload_parser.php?id='+value, data: formdata, processData: false, contentType: false, type: 'POST', success: function(data){ _("status").innerHTML = data; _("progressBar").value = 0; }, xhr: function() { var ajax = new window.XMLHttpRequest(); ajax.upload.addEventListener("progress", function (event) { _("loaded_n_total").innerHTML = "Uploaded "+event.loaded+" bytes of "+event.total; var percent = (event.loaded / event.total) * 100; _("progressBar").value = Math.round(percent); _("status").innerHTML = Math.round(percent)+"% uploaded... please wait"; }, false); return ajax; }, error: function (xhr, ajaxOptions, thrownError) { if(thrownError == "abort") { _("status").innerHTML = "Upload Aborted!"; return; } _("status").innerHTML = "Upload Failed"; } }); }); } </script> <script type="text/javascript" src="http://code.jquery.com/jquery-1.7.1.min.js"></script> <script type="text/javascript">
// hide lesson name edit div
function hide_lesson_edit_div() {
$("#hide_lesson_edit").fadeOut(300);
}
// hide lesson delete div
function hide_lesson_delete_div() {
$("#hide_lesson_delete").fadeOut(300);
}
// script for topics
function hide_topic_name_div() {
$("#topic_panel").fadeOut(300);
}
// hide edit topic name
function hide_topic_edit_name_div() {
$("#topicname_edit").fadeOut(300);
}
//
function hide_delete_topic_div() {
$("#hide_div_delete").fadeOut(300);
}
</script> </head> <body> <?php include('../../inc/db.php'); $id=$_GET["id"];
if(isset($_POST["add_lesson"])){
$lesson_name=$_POST["lesson_name"];
$module_id=$_POST["module_id"];
$insert = $conn->prepare("INSERT into lesson_details(module_id, lesson_name) VALUES('$module_id', :lesson_name)");
$insert->bindParam(':lesson_name', $lesson_name);
$insert->execute();
} else{ // edit lesson name if(isset($_POST["edit_lesson"])){ $lesson_name=$_POST["lesson_name"]; $lesson_id=$_POST["lesson_id"]; ?>
<form method="post" action="" id="hide_lesson_edit">
<input type="hidden" name="lesson_id" value="<?php echo $lesson_id;?>">
<input type="text" name="lesson_name" value="<?php echo $lesson_name;?>" />
<input type="submit" name="edit_lesson_name" value="Save" /> <a onClick="hide_lesson_edit_div()" style="cursor: pointer; text-decoration: underline;">Close </a>
</form>
<?php
}
if(isset($_POST["edit_lesson_name"])){
$lesson_name=$_POST["lesson_name"]; $lesson_id=$_POST["lesson_id"]; // update lesson name in the table $update=$conn->prepare("UPDATE lesson_details SET lesson_name= :lesson_name WHERE id=$lesson_id"); $update->bindParam(':lesson_name', $lesson_name); $update->execute();
} }
// delete lesson
if(isset($_POST["delete_lesson"])){
$lesson_id=$_POST["lesson_id"]; ?>
<form method="post" action="" id="hide_lesson_delete">
<input type="hidden" name="lesson_id" value="<?php echo $lesson_id;?>">
<h3> Are you sure, you want to delete the lesson???? </h3>
<input type="submit" name="confirm_lesson_delete" value="Yes" /> <a onClick="hide_lesson_delete_div()" style="cursor: pointer; text-decoration: underline;">Close </a>
</form>
<?php
}
if(isset($_POST["confirm_lesson_delete"])){
$lesson_id=$_POST["lesson_id"]; // delete lesson name in the table $delete=$conn->query("DELETE FROM lesson_details WHERE id=$lesson_id");
// delete topics also under that particular lesson // first select the details from the table to delete the video from the folder. first delete the video then delete everything form the table.
$select = $conn->query("SELECT * FROM topic_details WHERE lesson_id=$lesson_id"); foreach($select as $value){ $video_save_name = $value["video_save_name"]; // delete video from the folder if (!unlink("uploads/videos/$video_save_name")) { echo ("Error deleting the video"); } // delete details from the table $delete = $conn->query("DELETE FROM topic_details WHERE lesson_id=$lesson_id");
}
}
// add a new topic if(isset($_POST["add_topic"])){
$lesson_id= $_POST["lesson_id"];
?>
<form method="post" action="" id="topic_panel">
<input type="text" name="topic_name">
<input type="hidden" name="lesson_id" value="<?php echo $lesson_id; ?>">
<input type="submit" name="add_topic_name" value="Save"> <a onClick="hide_topic_name_div()" style="cursor: pointer; text-decoration: underline;">Close </a>
</form>
<?php
} if(isset($_POST["add_topic_name"])){
$lesson_id=$_POST["lesson_id"];
$topic_name=$_POST["topic_name"];
// insert topic name and leson id in the table topic_details
$insert=$conn->prepare("INSERT into topic_details(lesson_id, topic_name) VALUES('$lesson_id', :topic_name)");
$insert->bindParam(':topic_name', $topic_name);
$insert->execute();
}
if(isset($_POST["edit_topic_name"])){
$topic_id=$_POST["topic_id"];
$topic_name=$_POST["topic_name"];
?>
<form method="post" action="" id="topicname_edit">
<input type="hidden" name="topic_id" value="<?php echo $topic_id;?>">
<input type="text" name="topic_name" value="<?php echo $topic_name;?>">
<input type="submit" name="update_topic_name" value="Save"> <a onClick="hide_topic_edit_name_div()" style="cursor: pointer; text-decoration: underline;">Close </a>
</form>
<?php
}
if(isset($_POST["update_topic_name"])){
$topic_name=$_POST["topic_name"];
$topic_id=$_POST["topic_id"];
// upadte topic name in the topic_details table
$update = $conn->prepare("UPDATE topic_details SET topic_name = :topic_name WHERE id=$topic_id");
$update->bindParam(':topic_name', $topic_name);
$update->execute();
} // for video if(isset($_POST["show_video_panel"])){ $topic_id=$_POST["topic_id"]; ?>
<form id="upload_form" enctype="multipart/form-data" method="post">
<input type="hidden" name="topic_id" id="topic_id" value="<?php echo $topic_id;?>" />
<input type="file" name="file1" id="file1"><br>
<input type="button" value="Upload File" onClick="uploadFile()">
<progress id="progressBar" value="0" max="100" style="width:300px;"></progress>
<h3 id="status"></h3>
<p id="loaded_n_total"></p>
</form>
<?php
}
// delete topic if(isset($_POST["delete_topic"])){
$topic_id=$_POST["topic_id"];
$video_save_name=$_POST["video_save_name"];
$video_path=$_POST["video_path"];
?>
<form method="post" action="" id="hide_div_delete">
<input type="hidden" name="topic_id" id="topic_id" value="<?php echo $topic_id; ?>" />
<input type="hidden" name="video_save_name" value="<?php echo $video_save_name;?>" />
<input type="hidden" name="video_path" value="<?php echo $video_path;?>" />
Are You Sure, you want to delete a topic???
<input type="submit" name="confirm_delete" value="Yes" /> <a onClick="hide_delete_topic_div()" style="cursor: pointer; text-decoration: underline;">Close </a>
</form>
<?php
} if(isset($_POST["confirm_delete"])){
$topic_id=$_POST["topic_id"];
$video_save_name=$_POST["video_save_name"];
$video_path=$_POST["video_path"];
// delete a topic
$delete= $conn->query("DELETE FROM topic_details WHERE id=$topic_id");
// delete video from the folder also
if($video_path !== ""){
unlink("uploads/videos/$video_save_name");
}
}
?>
<h3> Add a Lesson to the Module </h3> <form method="post" action=""> Lesson Name: <input type="text" name="lesson_name" /> <input type="hidden" name="module_id" value="<?php echo $id; ?>" /><br /> <input type="submit" name="add_lesson" value="Add Lesson"/> </form> <h2> Table Of Contents </h2> <table> <?php $lessons=$conn->query("SELECT * FROM lesson_details WHERE module_id=$id");
foreach($lessons as $lesson) {
$lesson_name= $lesson['lesson_name'];
$lesson_id=$lesson['id']; ?>
<tr>
<td>
<h3> <?php echo "$lesson_name <br>";?> </h3>
</td>
<td>
<form method="post" action="">
<input type="hidden" name="lesson_name" value="<?php echo $lesson_name;?>">
<input type="hidden" name="lesson_id" value="<?php echo $lesson_id;?>">
<input type="submit" name="edit_lesson" value="Edit Lesson" />
</form>
</td>
<td>
<form method="post" action="">
<input type="hidden" name="lesson_id" value="<?php echo $lesson_id;?>">
<input type="submit" name="delete_lesson" value="Delete Lesson" />
</form>
</td>
<td>
<form method="post" action=""> <input type="hidden" name="lesson_id" value="<?php echo $lesson_id;?>"> <input type="submit" name="add_topic" value="Add Topic" /> </form> </td> </tr>
<?php
// select topic name
$topics=$conn->query("SELECT * FROM topic_details WHERE lesson_id=$lesson_id"); ?>
<?php
foreach($topics as $topic) {
$topic_id= $topic["id"];
$topic_name= $topic['topic_name'];
$video_save_name= $topic["video_save_name"];
$video_path = $topic["video_path"];
?>
<tr>
<td>
<?php echo "$topic_name";?>
</td>
<td>
<form method="post" action="">
<input type="hidden" name="topic_id" value="<?php echo $topic_id;?>">
<input type="hidden" name="topic_name" value="<?php echo $topic_name;?>">
<input type="submit" name="edit_topic_name" value="Edit Topic Name">
</form>
</td>
<td>
<form method="post" action="">
<input type="hidden" name="topic_id" value="<?php echo $topic_id;?>">
<input type="submit" name="show_video_panel" value="Add Video">
</form>
</td>
<td>
<form method="post" action="">
<input type="hidden" name="topic_id" value="<?php echo $topic_id;?>" />
<input type="hidden" name="video_save_name" value="<?php echo $video_save_name;?>" />
<input type="hidden" name="video_path" value="<?php echo $video_path;?>" />
<input type="submit" name="delete_topic" value="Delete Topic" />
</form>
</td>
</tr>
<?php
}
?>
<?php
}
?> </table> </body> </html>
//code for file_upload_parser.php
<?php
include('../../inc/db.php'); $topic_id = $_GET["id"];
/* Script written by Adam Khoury @ DevelopPHP.com / / Modified by Philip Graf @ PhilipGraf.at / / Video Tutorial: http://www.youtube.com/watch?v=EraNFJiY0Eg / / Edit Thread: https://teamtreehouse.com/forum/i-want-to-upload-video-file-with-progress-bar*/
$ini_PostSize = preg_replace("/[^0-9,.]/", "", ini_get('post_max_size'))(1024*1024); $ini_FileSize = preg_replace("/[^0-9,.]/", "", ini_get('upload_max_filesize'))(1024*1024); $maxFileSize = ($ini_PostSize<$ini_FileSize ? $ini_PostSize : $ini_FileSize); $file = (isset($_FILES["file1"]) ? $_FILES["file1"] : 0);
if(isset($_GET["getsize"])) { echo $maxFileSize; exit;
} if (!$file) { // if file not chosen
if($file["size"]>$maxFileSize){
die("ERROR: The File is too big! The maximum file size is ".$maxFileSize/(1024*1024)."MB");
}
die("ERROR: Please browse for a file before clicking the upload button");
} if($file["error"]) {
die("ERROR: File couldn't be processed");
} if($file["type"] !== "video/mp4") {
die("ERROR: You have to select a MP4-File");
} // chnage the name of the file to be uplaod $temp = explode(".", $file["name"]); $newfilename = "video". "$topic_id". '.' .end($temp); if(move_uploaded_file($file["tmp_name"], "uploads/videos/".$newfilename)){ echo "SUCCESS: The upload of ".$file["name"]." is complete"; } else { echo "ERROR: Couldn't move the file to the final location"; } ?>
Please help me out.
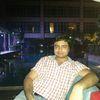
KnowledgeWoods Consulting
5,607 PointsHi, Philip It's showing total uploaded bytes also.

Philip G
14,600 PointsI'll reply to you soon

Philip G
14,600 PointsHi Chetan,
I think it gets to complicated to help you over Treehouse. Please contact me.
Looking forward to hear from you,
Philip
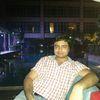
KnowledgeWoods Consulting
5,607 PointsHi, Philip. I have replied to you on your email.