Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial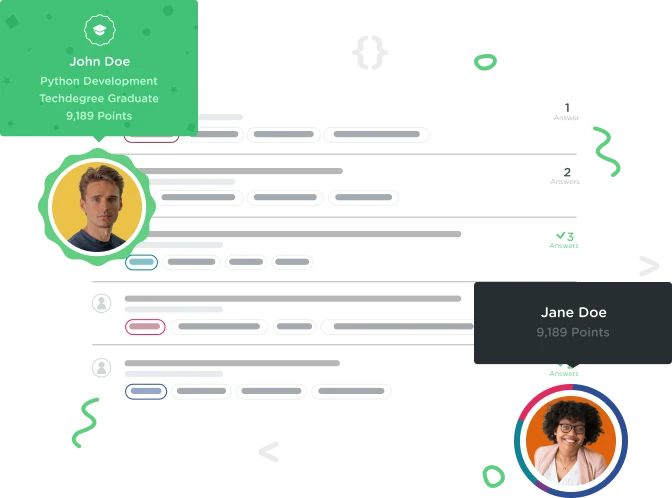

Nas Jones
7,849 PointsIf else statement error
https://w.trhou.se/y161k1smru. This is a snapshot of my workspace i'm still not done with everything yet. For question four and answer four in the if else statement i have a logical or operator. Maybe i didn't do it right but whenever i finish the five questions i always get 4 out of 5 right instead of all five. The correct answer to question 4 is "24" but i wanted to also put "twenty four" as an option too. I don't know why i'm getting four out of five correct, i'm assuming it's the logical or operator done incorrectly.
2 Answers
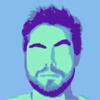
Cameron Childres
11,818 PointsHi Nas,
You're very close! The logical operator is done just fine, the issue here is the string "twenty four":
fourthQuestion.toUpperCase() === "twenty four"
Here you're taking the input for fourthQuestion, converting it to upper case, and comparing it to a lower case string. An answer of "twenty four" is turned in to "TWENTY FOUR" and compared against "twenty four".
If you change "twenty four" to upper case your code should work as intended:
fourthQuestion.toUpperCase() === "TWENTY FOUR"

Nas Jones
7,849 PointsI see, but would this be the best way to ensure that no matter what the user enters either lower or uppercase it will be correct?. Or is there a better way of making sure of that?.
Nas Jones
7,849 PointsNas Jones
7,849 PointsHey, thank you for the help i appreciate it. That worked and fixed it, just curious the solution you gave me I'm assuming it takes care of both lower case and upper case situations correct?. I know the ".toUpperCase()" converts it to upper case but I'm confused as to why i couldn't leave the string as "twenty four" doesn't it convert it to uppercase anyways?. I'm a little confused of the whole sequence when using ".toUpperCase()"
Cameron Childres
11,818 PointsCameron Childres
11,818 PointsFor sure! When you use a method like ".toUpperCase()" it only applies to what it's directly attached to. It won't have any affect on the string on the other side of the comparison operator:
In this set up the variable fourthQuestion will always be converted to uppercase before being checked. It's because of this we need the answer to be in uppercase to match:
No matter what mix of cases are given to fourthQuestion, they'll always be converted to uppercase before being checked:
Finally, if you wanted to leave the answer as "twenty four" you could instead use the ".toLowerCase()" method to ensure things match:
It's worth remembering that "===" is a comparison operator and does not assign or change anything about the string on the right at all, it just checks if the left and right side match and returns either true or false.
I hope this helps to clear things up -- if you've got any questions ask away :)