Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial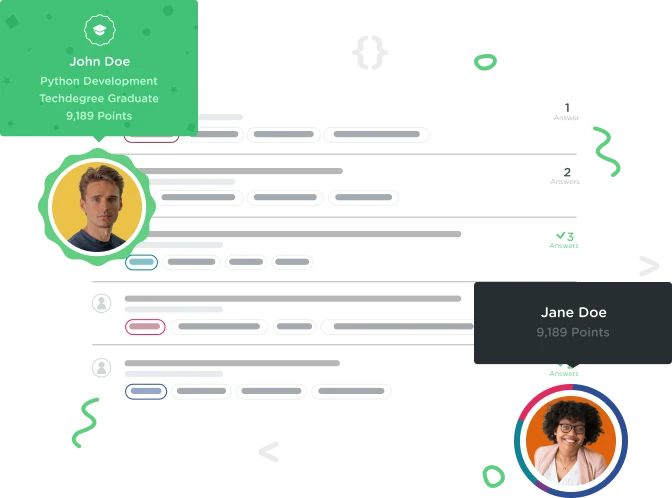

Jane Irwin
5,805 PointsIf we check in the first if statement to see if obj is a Point object, why do we need to cast it into a Point?
If it's already a Point, shouldn't we be able to compare it to other Points without casting?
2 Answers
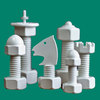
Steven Parker
231,126 PointsI'll take a guess.
Without seeing the code, I'm guessing this is a situation where you have a method that takes a generic object as a parameter, then inside the method the object is tested to see if it is a Point, and if so, it is cast to Point and assigned to a Point variable. Right?
So in that case, the casting isn't about changing the object, since as you mention, it is already a Point. But what the cast does is to make the class members of the Point available, and it also allows it to be used in ways that are only appropriate for a Point (for example: being passed to another method that requires a Point as a parameter).
Without the casting, you would only have access to class members that are common to all generic objects.

Jane Irwin
5,805 PointsThanks for your responses! ISorry -- first time asking a question in the forum; didn't know that it wouldn't automatically link to the lesson page in question. Here's the code:
https://teamtreehouse.com/library/intermediate-c/systemobject/objectequals
Challenge Task 1 of 1
Add an Equals method to the VocabularyWord class that returns true if the words of two VocabularyWord objects are the same.
using System; namespace Treehouse.CodeChallenges { public class VocabularyWord { public string Word { get; private set; }
public VocabularyWord(string word)
{
Word = word;
}
public override string ToString()
{
return Word;
}
public override bool Equals(object obj){
if(!(obj is VocabularyWord))
{
return false;
}
VocabularyWord that = obj as VocabularyWord;
return that.ToString() == this.ToString();
}
}
}
I think I partly understand... but I guess what I don't understand is why an object that's already a Point (or in this case, a VocabularyWord) would not already have access to the members of its class. Doesn't it automatically get access to its Parent class' members if it's created as an instance of the Parent class?
Thanks for your patience -- this is my first real experience with OOP and I'm trying to make sure I really have a handle on the fundamentals before proceeding forward in the lessons.
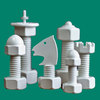
Steven Parker
231,126 PointsIt does automatically get access to the parent class. But what's happening here is since it was passed to the method as an object (not a VocabularyWord), it only has access the the parent (object) members until you cast it. It might be a VocabularyWord, but before casting the method code only sees it as an object and cannot use it to access members of VocabularyWord.
This is a significant OOP concept and I recognize your wish to understand it fully. I hope it makes more sense now.

Jane Irwin
5,805 PointsOhhhhh yes! Yeah, that makes sense now, and thanks for taking the time and effort to explain it to me.
Question -- if I'd passed in the var as a VocabularyWord object, would the method have access to all the parent class' methods, or would I have to cast it anyway? Is there any benefit to doing it that way or is it better to pass it to the method as an object and then cast?
Would something like this work?
public override bool Equals(VocabularyWord obj){ if(!(obj is VocabularyWord)) { return false; }
return obj.ToString() == this.ToString();
}
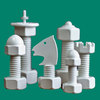
Steven Parker
231,126 PointsYes, that would work, but you could then eliminate the test for other types, since once you change the parameter any attempt to pass a different type would cause a compiler error.
And to your question, it has access to the parent class (object) members either way. It's only the VocabularyWord members that it doesn't have access to before casting when the parameter is declared as object. The difference between the argument types is that when the parameter is declared as an object, the method can be given any object as an argument and it will return a result. But if it is declared as a VocabularyWord, then it can only be passed objects of that type (or you get that compiler error I mentioned). In this case there's no choice to be made because this method is an override so the parameter type must match that of the original method.

Jane Irwin
5,805 PointsGot it! Thanks so much!
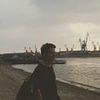
Calin Bogdan
14,921 PointsYou're overriding the Equals method from System.Object class which takes as parameter the 'object' type.
You can overload the method though:
public boolean Equals(Point point)
{
// check stuff
}
instead of overriding it. Notice that the 'override' keyword isn't there.
Steven Parker
231,126 PointsSteven Parker
231,126 PointsI'd be curious to take a look at this. Please provide a link to the course page you are referring to.