Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial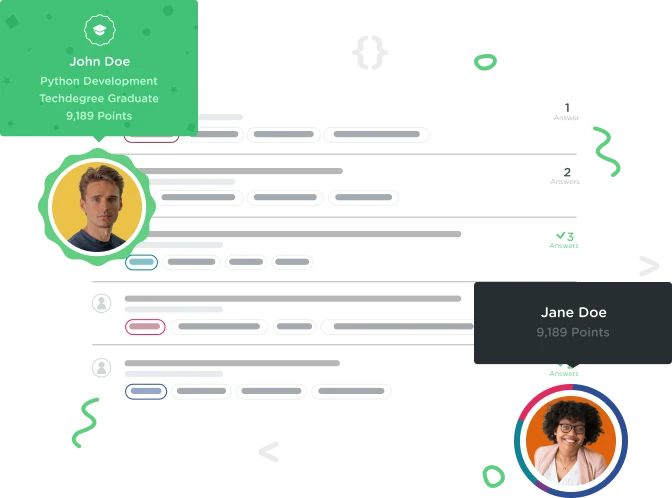
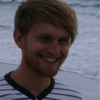
Daniel Schwemmlein
4,960 Pointsillegal argument exception from drive method
In this challenge I should put something into my drive method that says not enough bars left when there are not enough bars left to drive another lap. for some reason it won't work
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
int newAmount = mBarsCount - laps;
if (newAmount > 0) {
throw new IllegalArgumentException("Not enough battery remains");
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
5 Answers
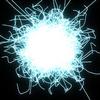
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey Daniel,
First off, we should decrement the mBarsCount after we check to see if there's enough battery left.
I see that you declared another variable, since we already have laps declared as an argument in the method parameters that won't be required, we can use our if statement like.
if (laps > mBarsCount) {
}
And then throw our method, I can see that you correctly threw the method, the only trouble this code is having is the if statement is throwing it off.
Overall your code should look something similar to.
public void drive(int laps) {
if (laps > mBarsCount) {
throw new IllegalArgumentException("Not enough battery remains");
}
mBarsCount -= laps;
}
Thanks let me know if this doesn't help. Just looks like you were slightly over thinking it.

Emmet Lowry
10,196 PointsHi Rob could you explain this a bit more for me please I am having a tough time understanding this and any help would be appriciated thx.
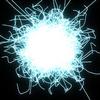
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey there Emmet,
Sure, happy to try. Basically what is going on is in this challenge is we are trying to catch errors before they become problems at run time.
What we are doing is adding an error that is tossed to a user in the event that they try to call drive with a larger amount of laps than what is called, let's say there are 4 bars left in the battery, if we weren't prepared for the error and someone called drive(5);
We'd want to make sure that we let the user know that there wasn't enough power in the battery.
Now we got the aim out of the way we can work on the code.
what we have is a method that accepts an int as a parameter, which is referencing how many laps we want to drive, since we put no control over the number that can be added in a user can put something like in like 36 laps, we'd want to toss them some error letting them know it wasn't going to work right?
So we start with our first check.
if (laps > mBarsCount) {
}
What we're doing right here is checking to see if there is enough battery (which we represent as the int value of mBarsCount), if the value passed into method, which is laps is too great we first want to make sure to throw an exception saying that there isn't enough battery first.
Notice that we do this test before we actually decrement laps this is to make sure that we don't have anything weird happen in our program by an odd parameter being added in.
The method would then return back true or false for the if statement, if the method was calling for a higher number of laps than what the cart had power for, we'd stop.
Otherwise, if not we have it continue on, where it decrements laps.
Thanks, I hope this helps.
However let me know if there's anything I can further clarify and I certainly will.

Emmet Lowry
10,196 PointsThanks so much Rob for the help and taking the time to explain it to me. I get what is trying to be achieved now. Thanks again.

Emmet Lowry
10,196 Pointshi Rob just thought about something. Not sure how the loop works could you give me a quick explanation and the difference between class variables and instant variables. Thanks for any answer.
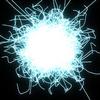
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey there Emmet
An if loop is pretty basic but very powerful thing it's syntax is basically
if (condition is true) { run this code }
So in this reference here's what java sees when we type If (laps > mBarsCount)
It first checks to see if the variable is true, if we have indeed calls a higher number of laps than the value stored in mBars Count.
If it validates that this is true that it's going to run our code in the if statement. Which is to to throw the exception, keep in mind this only runs if laps is greater than mBarsCount,
So if we calls this method with a value of one, all the code inside the if statement would be ignored, it wouldn't matter because the condition did not evaluate to true. In fact the entire loop is nessecary if we were able to assume that laps would lower than mBarsCount, unfortunately this is not an assumption that we can make, so we need to add it to cover all of our bases.
Let's take for instance another if loop. we'll put this method inside a method that returns true if the values are equal
public boolean isTrue(int x, int b) {
x = 2
b = 2
boolean isEven = false;
if (x = b) {
System.out.println("These values are equal");
isEven = true;
}
return isEven;
}
What happens in this if loop is they first check and see if the condition x = b is true, if it is than it will print out, that they are properly equal. and set the value of our boolean variable to true before returning it.
But let's say we changed the values to below.
public boolean isTrue(int x, int b) {
x = 3
b = 2
boolean isEven = false;
if (x = b) {
System.out.println("These values are equal");
isEven = true;
}
return isEven;
}
In the above example the examples are not true so the code inside the if block doesn't even run, we never see that they're equal and we never change the value, because the condition of x = b was never met, that's more or less an if block.
With that being said an instance variable and a class variable and an instance variable is if a single variable is shared between multiple classes, or if each class has it's own instance of that variable.
The easiest way would be with a bicycle example.
Let's say each bicycle has it's own color, which is great, so in every different bicycle class that we make we can have an instance variable where the value changes throughout the different classes, let's say we have 3.
Bike, MoutainBike, and DirtBike.
We could have 3 different instance variables for color in this setting, since we probably would like them all to have their own color, let's say Bike is blue, MoutainBike is Green, DirtBike is yellow. It works, and these are all instance variables because they change throughout different instances of our class.
Let's say however, we -really- like the color red, it's the best color and why would we ever need something else to be any color but red.
We could a create a single Class variable color by calling it static and declaring it red, it would look like below.
public static String ="red";
Then we could have each different class this variable because, we never plan to make another another color variable.
To try to put it simply, instance variables is a variable that is defined multiple times throughout each class with a differing value.
A class variable is a single variable that has a value that is shared between all of the classes of bicycle.
Thanks, I hope this helps.
Of course let me know if it doesn't.

Emmet Lowry
10,196 PointsThanks for response rob.I understand it now so thank you.