Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial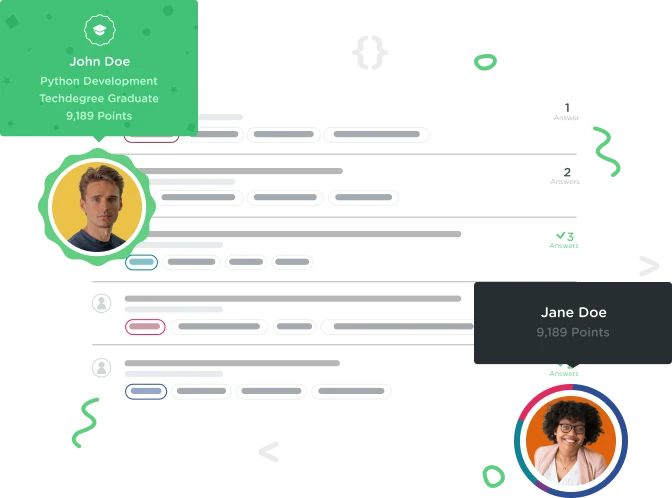

kevinkrato
5,452 PointsI'm butchering this JavaScript Function
I'm trying to come back and do a simple review of this section. I'm completely messing it up though. Where am I going wrong?
function getRandomNumber (lower, upper) {
return Math.floor(Math.random() * (upper - lower + 1)) + lower;
if (upper === isNaN || lower === isNaN) {
throw new Error('Please write a digit.');
} else if (lower >= upper) {
prompt('Your lower number was not lower than your higher value. Please enter a number lower than ' + upper +'.');
} else {
console.log(getRandomNumber(lower, upper));
}
}
var upper = prompt('Please choose a number');
var lower = prompt('Please choose an additional number lower than your previous selection.');
console.log(getRandomNumber(lower, upper));
3 Answers
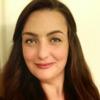
Jennifer Nordell
Treehouse TeacherHi there! I feel like when I point this out, you can continue making progress on this. When a return
statement is hit, the function ceases execution right there. Your return statement happens on the first line of your function. Any and all statements below that will never be executed.
Hope this helps!
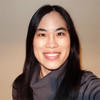
Diana Le
6,794 PointsThe way you're calling the isNaN function is different than what was in the lessons. It was doing some weird results when I ran a clean version of your code so I switched it to
isNaN(upper)
instead and that fixed the issue.
Also remember to convert the lower and upper variables to numbers in your function, using parseInt() or parseFloat() depending on if you want the user to input decimals. They're still processed as strings otherwise so you won't get your desired results.
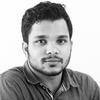
Abdulla Hussain
8,221 Pointsfunction getRandomNumber (lower, upper) {
/*
Use isNan() function here. MDN reference here:
https://mzl.la/2keIBqy
*/
if (isNaN(lower) || isNaN(upper)) {
throw new Error('Please write a digit.');
} else {
/*
You had this return statement at the top.
Program exits the function the moment return statement is hit.
Thus, I moved it to the bottom.
*/
return Math.floor(Math.random() * (upper - lower + 1)) + lower;
}
}
/*
That's the end of your function. Notice the IF statement below,
which allows you to give user a second chance to enter the amount
and UPDATES value of variable LOWER - if user fails, an error is thrown.
You may also notice all prompt dialogs have parseInt() function so input
values are instantly converted to integer.
*/
var upper = parseInt(prompt('Please choose a number'));
var lower = parseInt(prompt('Also, provide a second number less than ' + upper));
if (lower >= upper) {
lower = parseInt(prompt('Your lower number was not lower than your higher value. Please enter a number lower than ' + upper +'.'));
if (lower >= upper) {
throw new Error('Exiting the program since you could not provide a number less than your first value');
}
}
console.log(getRandomNumber(lower, upper) + ' it between the two numbers you provided.');
There may be a better way than this. The above method worked for me well. You may want to expand this by giving a 2nd chance when a text value is entered.