Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial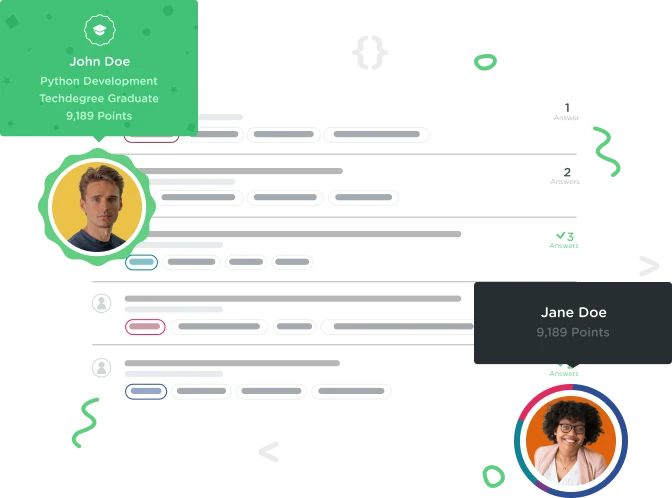

Chris Stringer
4,813 PointsI'm confused. Am I supposed to make sure that it does not say no or that it is only allowed to say no
I do not understand why my code is not going through, am I supposed to make sure this is only allowed to say no or say anything other than no?
// I have initialized a java.io.Console for you. It is in a variable named console.
String response;
boolean isValidWord;
do {
response = console.readLine("Do you understand do while loops? ");
isValidWord = (response.equalsIgnoreCase("no"));
};
3 Answers

andren
28,558 PointsYou are supposed to continue looping if the response is No (notice the capital N, programming is not case insensitive so matching the requested capitalization is essential). The problem (other than the case issue) is that your do not define a while condition for your do loop. Simply setting a Boolean to true won't make the do loop continue. You have to provide said Boolean as a condition for the loop like this:
String response;
boolean isValidWord;
do {
response = console.readLine("Do you understand do while loops? ");
isValidWord = (response.equalsIgnoreCase("No"));
} while (isValidWord); // A do loop always needs a while condition attached to it.
You can also shorten the code a bit by not storing the boolean in a variable and instead using its value directly as a condition like this:
String response;
do {
response = console.readLine("Do you understand do while loops? ");
} while (response.equalsIgnoreCase("No")); // This works exactly like the example above.
Variables are often not necessary if you only need to use the value in one place in your code. Its when you start repeating the same value, or need to store a value for use later in the program that variables becomes really useful.
Edit: I just realized that you use equalsIgnoreCase
which means that the case issue actually doesn't matter at all. I made this post shortly before going to bed so I'll have to blame that little brain fart on being a bit sleep deprrived.
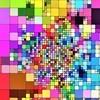
james south
Front End Web Development Techdegree Graduate 33,271 Pointsi think it is just supposed to loop, asking for the input, until it gets an answer. so i think it would be something like DO - prompt for response - WHILE response is empty. a do-while loop will execute at least one time, so there will be at least one prompt for a response, and if it isn't given, the loop continues until a response is given. at that point the while condition fails and you move on.

Chris Stringer
4,813 PointsThank you guys, I appreciate and took from what you all told me. //Declare a String variable named response, then initialize it using double quotes String response ="";
// The do while loop keeps asking the same question until "yes" is entered //So we want to keep repeating when "no" is typed // Exit the loop when "yes" is entered
do{ response = console.readLine("Do you understand do while loops?"); // "yes" or "no" is stored in the response variable // when someone types "no" the loop repeats // when someone types "yes" the loop is exited }while(response.equalsIgnoreCase("no"));
//After the loop exits, the following line prints to the console console.printf("Because you said %s, you passed the test!",response); //task 3

andren
28,558 PointsThis is more or less correct, the only thing I would point out is that there is nothing special about the word yes
, any word at all other than no
will cause the loop to exit, not just the word yes
.
Also after a night of sleep I realize that you used equalsIgnoreCase
in your code, meaning that my comments on matching the case being important was in fact wrong. I apologize for that. I''ll have to blame it on being a bit sleep deprived at the time I made this post.