Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial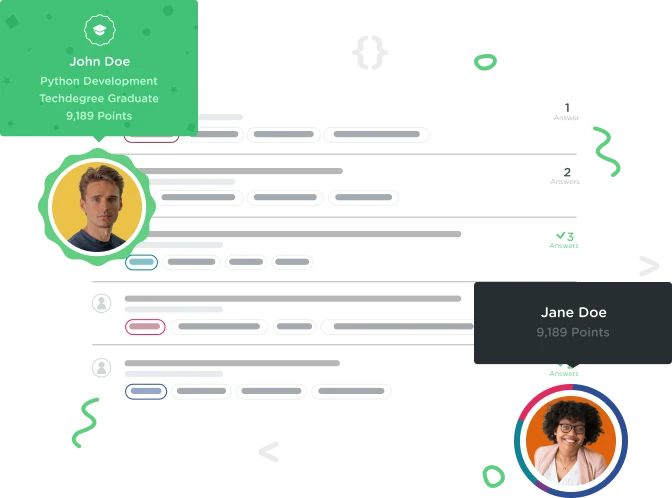

chevano gordon
3,399 PointsI'm frustrated someone please tell me what i'm doing wrong
public class ScrabblePlayer {
private String mHand;
private String mHits;
private String mMisses;
public ScrabblePlayer() {
mHand = "";
mHits = "";
mMisses = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public boolean applyGuess(char letter)
{
boolean isHit = mHand.indexOf(letter) >= 0;
if(isHit)
{
mHits+= letter;
}
else
{
mMisses +=letter;
}
return isHit;
}
public int getTileCount(char lets)
{
int counter = 0;
for(char letter : mHand.toCharArray())
{
if(mHand.indexOf(lets) >= 0)
{
counter++;
}
else
{
counter = 0;
}
}
return counter;
}
}
public class ScrabblePlayer {
private String mHand;
private String mHits;
private String mMisses;
public ScrabblePlayer() {
mHand = "";
mHits = "";
mMisses = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public boolean applyGuess(char letter)
{
boolean isHit = mHand.indexOf(letter) >= 0;
if(isHit)
{
mHits+= letter;
}
else
{
mMisses +=letter;
}
return isHit;
}
public int getTileCount(char lets)
{
int counter = 0;
for(char letter : mHand.toCharArray())
{
if(mHand.indexOf(lets) >= 0)
{
counter++;
}
else
{
counter = 0;
}
}
return counter;
}
}
10 Answers
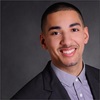
Mario Blokland
19,750 PointsHi chevano gordon,
what you are currently doing is, searching for the first occurrence of a character in a word. This is not what you want. Lets say the word is 'office' and the character is f
. This would return true
for every count in the loop. Since the word office
, has 6 characters, your counter would equal 6.
Now lets say you have made the correct if statement. With the same example I just made, your counter would return 0, even though it found the character 'f' two times, but because the last character of office
is not a f
, would return false, then go to your else statement and initialize the value of 0 to the variable counter
. Since the loop would be finished, your method would return 0.
What you want to do is something like this:
public int getTileCount(char tile) {
int counter = 0;
for(char letter : mHand.toCharArray())
{
if( ? ) // if letter the current letter of the word equals the tile
{
counter++;
}
}
return counter;
}
Let me know if you have some more questions or something wasn't clear.
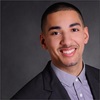
Mario Blokland
19,750 PointsHi mate,
sorry for the confusion :-). Had a long working day. Of course the word office
has 6 characters and only one c
. Just switch the c
with an f
. I will correct my post after this one.
If
conditions ALWAYS evaluate to true
or false
.
if (1 > 2) // false
if (1 < 2) // true
if ('f' == 'c') // false
if ('f' == 'f') // true
// Also, every number but 0 equals true
if (0) // false
if (3) // true
if (-1) // true
...
Lets get to your question: Why would your counter be equal to 0, since it already found a matching character (if your if condition was right) ?
You have to think about what your method does in every step.
Lets take again, the word office
. I will write some pseudocode and take your previous method with a correct if condition.
public int getTileCount(char tile) { // lets take 'f' as the tile
int counter = 0;
for(char letter : mHand.toCharArray())
{
if( the current letter of the word equals the tile )
{
counter++;
}
else
{
counter = 0;
}
}
return counter;
}
/**
* 1. letter: is 'o' equal to 'f'? false => counter = 0 (counter is 0)
* 2. letter: is 'f' equal to 'f'? true => counter++ (counter is now 1)
* 3. letter: is 'f' equal to 'f'? true => counter++ (counter is now 2)
* 4 letter: is 'i' equal to 'f'? false => counter = 0 (the current value 2, will be overwritten and counter is now 0 again)
* 5. letter: is 'c' equal to 'f'? false => counter = 0 (counter stays 0)
* 6. letter: is 'e' equal to 'f'? false => counter = 0 (counter stays 0)
* Loop finished: return counter (0)
*/
Can you see now why the value would be 0 at the end if your else statement is counter = 0
?
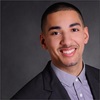
Mario Blokland
19,750 PointsHi Chevano,
yes, you could leave the else statement there and set it equal to the current value (counter = counter). But, the else statement is unnecessary in this case. counter = counter
is the same as leaving counter as it is. This means, we don't need the else statement at all. You can just remove it from your code and nothing will change if nothing is found.
The if statement should evaluate this:
Is the current character of the word equal to the given character?
Let me help you a little bit more
public int getTileCount(char tile) {
int counter = 0;
for(char letter : mHand.toCharArray()) {
if( ? == ? ) {
counter++;
}
}
return counter;
}
Exchange the ? signs with variables. The tile is given as a parameter, the other one is the current letter of the word in the loop.

chevano gordon
3,399 PointsHi @Mario Blokland
I don't think I follow your logics completely, for instance you mentioned that the word office has 7 characters but to my knowledge it's 6 character and you said that if we loop through the word 'office' I will find two occurrences of the character c . I'm not sure how that is possible since the word office contains only one 'c' character. And you mentioned the boolean true and false, are these booleans needed in the code and while. The next thing that I am not certain about is why would my counter be equal to 0 since it already found a matching character.
Your information is quite helpful but I just need to know more to have a better understanding and I do enjoy your method of helping, please help me in understanding what is being asked in more detailed along with the explanation. thank you.

chevano gordon
3,399 PointsHI, @Mario Blokland,
Everything is finally making sense, and yes I understand why the counter in the else state can't be equal to 0. What make sense is setting that counter to be equal to counter = counter in the else statement where the count will remain the same as long as the statement is false and increment higher if a statement is found true. what I'm not getting now his the path that is require of me to take. in other words the if statement is not clear.
thank you again I am really seeing progress.

chevano gordon
3,399 PointsHi @Mario Blokland,
I am not certain but my guesses are if(mHand.indexOf(letter) == tile) || if(mHand.charAt(letter) == tile) I am really appreciative of your help and I am sorry for this taking as much time as it is.
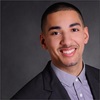
Mario Blokland
19,750 PointsHi chevano gordon,
this is no problem at all :-). I love to help! I always try to do this with much explanation instead of just presenting the answer. In my opinion one learns best when there is something to think about and to solve. After you solved the problem yourself, you will have a much better idea compared to copy/pasting an answer.
Just exchange the question marks and nothing else (no extra work needed) in the code of my last answer. Also if you look real close, you see two words in my last answer which look a little bit italic, those may also be variable names (?!). One of them you have already guessed. (tile
).
Extra Hint:
for (char currentCharacterOfTheWord : mHand.toCharArray()) {
// some code
}
currentCharacterOfTheWord would be the representation of each character in the loop. (It would iterate over each character). Thus:
public int getTileCount(char tile) {
int counter = 0;
for(char currentCharacterOfTheWord : mHand.toCharArray()) {
if( currentCharacterOfTheWord == tile ) {
counter++;
}
}
return counter;
}
would be correct in this case.
Do you know now which last variable you have to exchange with mHand.indexOf(letter) == tile) || if(mHand.charAt(letter) in your last comment?

chevano gordon
3,399 PointsHi @Mario Blokland,
I got the answer but I don't understand the logics behind it please demonstrate. the answer is " if(letter == tile) " . what i don't understand about it is that letter is the starting point right and my assumption of mHand.toCharArray() is a the answer being loop through . so the statement is basically saying its going from a starting character to the end of the set set letters stored in the real answer which is located in mHand. Please I need to understand. oh and I can't seem to thank you enough, you are a great helper.
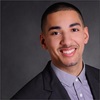
Mario Blokland
19,750 PointsHi chevano gordon,
great to hear that you made it through the task :).
You are totally right. The variable letter
is the starting point. It represents each character of the word, by iterating through each character with the help of the loop.
mHand holds the word. Lets say office
again. What mHand.toCharArray
does is the following.
['o', 'f', 'f', 'i', 'c', 'e'] (this is an array of characters)
Now the loop iterates 6 times (because the word has 6 characters, or in other words, the array has 6 items), with the variable letter
representing each character ('o' in the beginning of the loop and 'e' and the end of the loop).
So yes, everything you wrote was correct :-). Glad that you understood it.
And thank you for your kind words! If you have any other questions, don't hesitate to ask!

chevano gordon
3,399 PointsHi Mario,
I will surely keep you posted.

chevano gordon
3,399 PointsHi Mario Blokland,
Could you please help me understand what is going on here. I don't understand how a method signature works are what is its purpose. Please enlighten me, thank you in advance.
public class Example {
public static void main(String[] args) { ShoppingCart cart = new ShoppingCart(); Product pez = new Product("Cherry PEZ refill (12 pieces)"); cart.addItem(pez, 5); /* Since a quantity of 1 is such a common argument when adding a product to the cart, * your fellow developers have asked you to make the following code work, as well as keeping * the ability to add a product and a quantity. / Product dispenser = new Product("Yoda PEZ dispenser"); / Uncomment the line following this comment, after adding a new method using method signatures, to solve their request in ShoppingCart.java */ // cart.addItem(dispenser); }
} public class ShoppingCart {
public void addItem(Product item, int quantity) { System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName()); /* Other code omitted for clarity. Please imagine lots and lots of code here. Don't repeat it. */ } }