Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial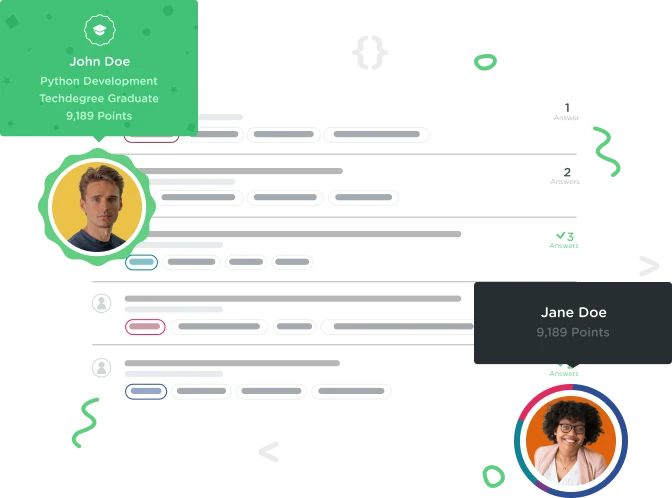

Sam DeClerk
3,444 PointsI'm lost
I'm not sure how to go about tackling this. If I should pass variables from the previous function into the next recursively, or declare an entirely different test. I feel like I'm over complicating this question. How does the variable declared as "int" pass into the next function, or does it?
package com.teamtreehouse.vending;
import org.junit.Test;
import static org.junit.Assert.*;
public class CreditorTest {
@Test
public void addingFundsIncrementsAvailableFunds() throws Exception {
Creditor creditor = new Creditor();
creditor.addFunds(25);
creditor.addFunds(25);
assertEquals(50, creditor.getAvailableFunds());
}
@Test
public void refundingReturnsAllAvailableFunds() throws Exception {
Creditor creditor = new Creditor();
creditor.addFunds(10);
int refund = creditor.refund();
assertEquals(10, refund);
}
@test
public void refundingResetsAvailableFundsToZero() throws Exception {
Creditor creditor = new Creditor ();
creditor addFunds(0);
assertEquals(0, creditor.getAvailableFunds());
}
package com.teamtreehouse.vending;
public class Creditor {
private int funds;
public Creditor() {
funds = 0;
}
public void addFunds(int money) {
funds += money;
}
public void deduct(int money) throws NotEnoughFundsException {
if (money > funds) {
throw new NotEnoughFundsException();
}
funds -= money;
}
public int refund() {
int refund = funds;
funds = 0;
return refund;
}
public int getAvailableFunds() {
return funds;
}
}
1 Answer

Boban Talevski
24,793 PointsHi Sam,
I think you are indeed over complicating this task. As far as I understand (still didn't get time to get the hang of testing in a separate project), a test is a single unit of execution isolated from the outside world (including other tests), so there's no need to think of passing variables from one test to another or assuming any relationships between the tests.
I got the impression that that's the whole point of creating tests, isolating a single functionality in a single test, without depending on other tests. All tests should be separate and not depend on each other. Otherwise, how would we know which functionality in our app is broken if one test failure creates some domino effect and causes several other tests to fail. Or if two separate functionalities are tested in a single test, how would we know which part of our app has a bug since we are testing more than one in a single test (this particular issue is the one addressed in this task).
So, in this task, you just need another test with the same Arrange and Act parts, while the Assert part of the existing test will be split between the two tests, the existing one - refundingReturnsAllAvailableFunds will keep the assertEquals(10, refund);
part and the new one - refundingResetsAvailableFundsToZero will take care of assertEquals(0, creditor.getAvailableFunds());
.
In other words, this is how those two tests should look like:
@Test
public void refundingReturnsAllAvailableFunds() throws Exception {
Creditor creditor = new Creditor();
creditor.addFunds(10);
int refund = creditor.refund();
assertEquals(10, refund);
}
@Test
public void refundingResetsAvailableFundsToZero() throws Exception {
Creditor creditor = new Creditor();
creditor.addFunds(10);
int refund = creditor.refund();
assertEquals(0, creditor.getAvailableFunds());
}