Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial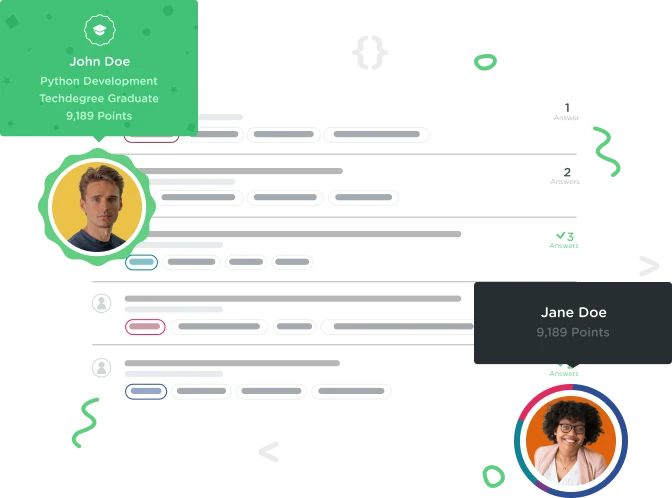

Marielle Quizon
2,830 PointsI'm not sure if I understand the instructions correctly that I can't provide the appropriate code for this challenge :(
I'm not sure if I understand the instructions correctly that I can't provide the appropriate code for this challenge :(
var max = function(num1, num2) {
if (num1 > num2) {
alert(num1 + " is greater than " + num2 + " .");
return num1;
}
else {
alert(num2 + " is greater than " + num1 + " .");
return num2;
}
}
max(2,5);
alert( max( ) );
3 Answers
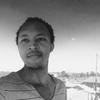
Dan Weru
48,030 PointsHey, you are doing fine up to the point you need to call the function. Below is the right way of doing it.
var max = function(num1, num2) {
if (num1 > num2) {
alert(num1 + " is greater than " + num2 + " .");
return num1;
}
else {
alert(num2 + " is greater than " + num1 + " .");
return num2;
}
}
/*
There are two ways of calling the function. You could call it directly in the alert,
or you could save it first in a variable, then pass the variable to the alert.
Either way will work.
*/
//OPTION 1
alert(max(2,5));
// OPTION 2
// OR you could save the result in a variable,
// then pass it to alert like below
/*
var maxOrMin = max(2,5);
alert(maxOrMin);
*/

Winston Quin
10,359 PointsI'm trying your suggestion and it's not working for me either. When you posted your answer, One Weru, you put your suggestions below the second curly brace at the bottom. Is that where the alert should be? I don't understand your answer. Could you show me the code in its entirety exactly as it is supposed to be entered?

Marielle Quizon
2,830 PointsHey winston, I got the answer correctly by changing my 'alert's inside my conditional statements to 'console.log' and tried One Weru suggestion in calling my function and it worked :) Here's a screenshot below:
Marielle Quizon
2,830 PointsMarielle Quizon
2,830 PointsThanksss! Great help! :)
Dan Weru
48,030 PointsDan Weru
48,030 PointsYou're most welcome
Marielle Quizon
2,830 PointsMarielle Quizon
2,830 PointsHey, Sorry to bother again, but I tried the codes you suggested. It still displays the same error "Did you pass 2 numbers to the
max()
function." :(Dan Weru
48,030 PointsDan Weru
48,030 PointsI tested the code from my browser. It works fine.
alert(max(2,5));
Marielle Quizon
2,830 PointsMarielle Quizon
2,830 PointsOhh. I got it now. Just a minor error inside my function. Thanks again! :D