Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial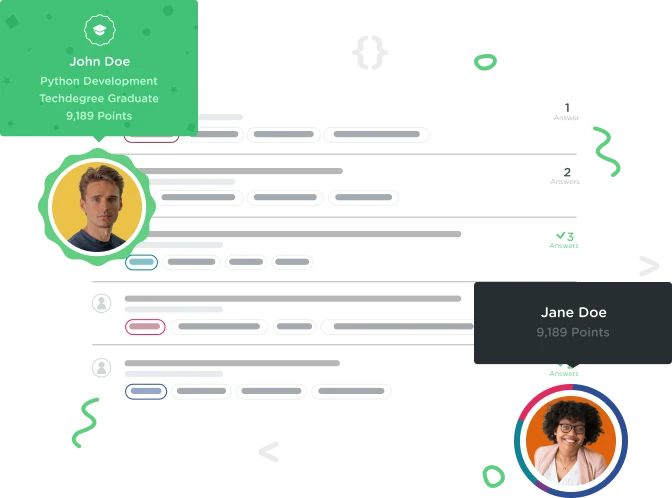

Jeff Shephard
784 PointsI'm stuck!
Been stuck on this question: "Create a new function named max which accepts two numbers as arguments (you can name the arguments, whatever you would like). The function should return the larger of the two numbers. β¨HINT: You'll need to use a conditional statement to test the 2 parameters to see which is the larger of the two."
Here's my answer:
function max(mine, yours) { var larger = Math.max( parseInt(mine), parseInt(yours) ); } if ( parseInt(mine) < parseInt(yours) ) { return parseInt(mine); } else if ( parseInt (mine) < parseInt(yours) ){ return parseInt(yours); }
Any help would be greatly appreciated!
Thanks,
Jeff
2 Answers

Jeff Shephard
784 PointsThanks for your help!
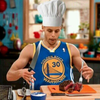
0yzh σ
17,276 PointsHey Jeff,
It looks like you have your conditional statement outside of your function. The challenge wants you to create a function that takes two arguments that are numbers and returns the larger of the two. To do that you need to put your if/else statement inside the function code block, you also don't need the variable larger because you can compare the two number arguments in your conditional statement. You can try something like this:
function max(a,b) {
if (a > b) {
return a;
}
else {
return b;
}
}
Or ...
function max(a,b) {
if (parseInt(a) > parseInt(b)) {
return a;
}
else {
return b;
}
}