Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial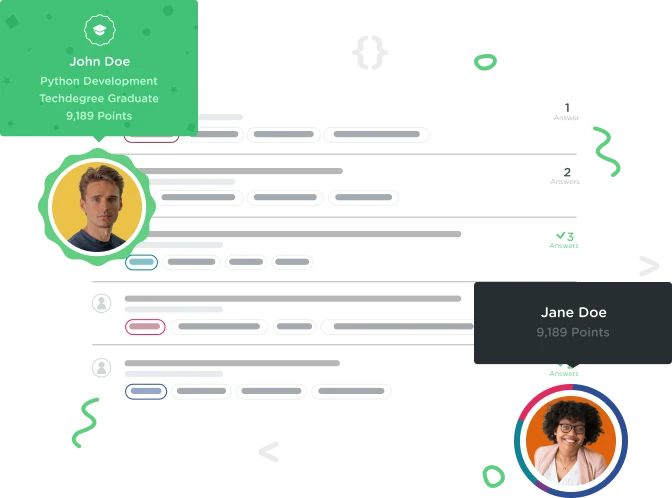
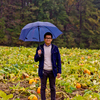
Mark Deguzman
5,196 PointsI'm stuck on this challenge in javascript.
Create a new function named max() which accepts two numbers as arguments. The function should return the larger of the two numbers. You'll need to use a conditional statement to test the 2 numbers to see which is the larger of the two.
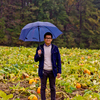
Mark Deguzman
5,196 PointsI'm lost sorry!
function max(smallerNum, largerNum) {
var largerNum = (12)
var smallerNum = (2)
if (largerNum > smallerNum) {
return largerNum;
}
}
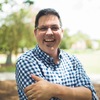
Dave McFarland
Treehouse TeacherMark Deguzman
To put code into a forum post use triple back ticks -- ``` — around the code. I fixed your code here, but in the future here's a forum discussion that describes how to add HTML, CSS, JavaScript or other code to the forum: https://teamtreehouse.com/forum/posting-code-to-the-forum
8 Answers
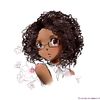
Gloria Dwomoh
13,116 PointsHi again Mark :) , first things first. Numbers don't go in parenthesis, parenthesis are for functions. Another thing to note is it is good to end javascript lines with semicolon. Lastly according to your code...what if the first number isn't bigger, you never know what the function takes as numbers and you shouldn't compare the numbers you have in the function as you want to compare the arguments given to the functions and not the variables you have put in them. The code you have written never compares the arguments that are passed to the function, it always keeps comparing the ones you have initialized in the function. You need something that can work with any set of arguments you give it. Something like this is more generalized and will work no matter what set of numbers you give the function
function max(num1, num2) {
if (num1 > num2) {
return num1;
}
else{
return num2;
}
}
I hope this helps.
Ps let me add this...
var largerNum = (12) /*This should be -> var largerNum = 12; if you are trying to create a
variable holding a number but you don't need this here */
what you have written above looks like an incomplete function, remember when we try to pass an argument to a function and we do something like...
max(3, 4) ? That parenthesis serves for functions but variables don't need one to be initialized.
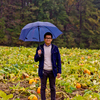
Mark Deguzman
5,196 PointsThanks gloria for clearing this up!
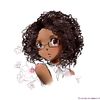
Gloria Dwomoh
13,116 PointsYou are welcome.

Jordan Green
10,767 PointsHere is another solution using ternary operator
function max(x, y){
return (x > y ? x:y)
};

Joshua David
5,494 PointsAren't you not supposed to use the semicolon on the end of a function?
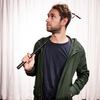
guymanno
1,477 PointsAs a few people have mentioned you shouldn't be passing actual numbers in to the function. My working solution is below. Not until you call the function at the very end will you need to give 'max' values...
function max(number1, number2){
if (number1 > number2){
return number1
}else {
return number2
}
}
max(2, 6);
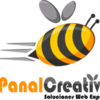
J. Alfonso Vélez Valdez
Courses Plus Student 4,511 PointsAt first I tried the following
function max(6, 2) {
if ( 6 > 2 ) {
return 6;
} else { return 2;
}
}
why was it wrong when using Integers?
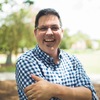
Dave McFarland
Treehouse TeacherThe function, max()
, requires parameters, which must be valid variable names. You've added literal numbers as the parameters, which won't work. Try this:
function max(a, b) {
if ( a > b ) {
return a;
} else {
return b;
}
}
console.log( max(6,2) );

Anand Mohan Duddella
Courses Plus Student 8,264 Pointsfunction max(x,y){ if (x > y){ return x; } else { return y; } } console.log (max(5,8));
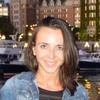
Elena Torrey
9,156 PointsThis worked for me:
function max (Num1, Num2) {
if (Num1>Num2) {
return Num1;
} else {
return Num2;
}
}
alert(max(14,18));
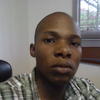
MUZ141139 Kenny Mpariwa
13,596 Pointsfunction max(num1, num2) { if (num1 > num2) { return num1; } else{ return num2; } }
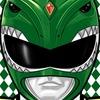
Randy Singh
Full Stack JavaScript Techdegree Student 1,462 PointsI had a similar problem to J. Alfonso above, where I was using actual integers. Heres what worked for me.
function max ( numberOne, numberTwo ) {
if ( numberOne > numberTwo ) {
return numberOne;
}
else {
return numberTwo;
}
}
max(4, 7);
Gloria Dwomoh
13,116 PointsGloria Dwomoh
13,116 PointsHi Mark, can you show us the code you have been working on?