Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial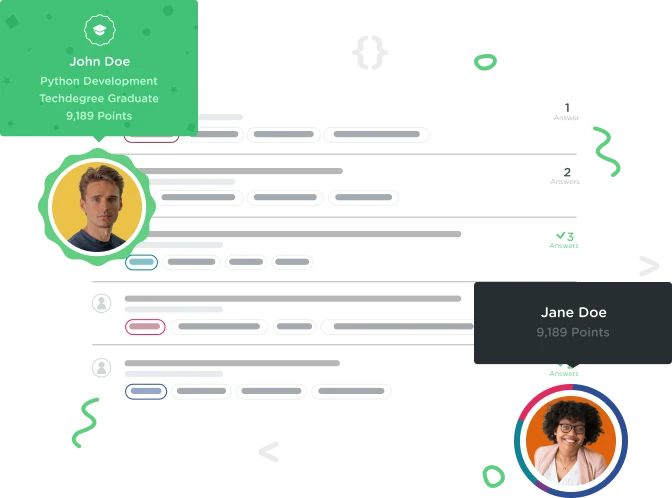

Noelle Acheson
610 PointsI'm told I didn't catch the exception? Not sure what I'm doing wrong...
I'm following the format given in the lecture (I think?), but I'm not catching the exception.
public class Main {
public static void main(String[] args) {
GoKart kart = new GoKart("yellow");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
try {
kart.drive(40);
}
catch (IllegalArgumentException iae) {
System.out.println("Too many laps");
}
kart.drive(2);
}
}
3 Answers
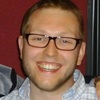
Victor Learned
6,976 PointsSo when you use a try catch statement you want all the code that could throw an exception that you specifically want to handle in your catch to be within the try.
In the text of the challenge it states that the drive method will throw an exception and thus all calls to the drive method need to be within your try. In you code you have one that is not. Either remove it or move it into the Try.
public static void main(String[] args) {
GoKart kart = new GoKart("yellow");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
try {
kart.drive(40);
kart.drive(2); //not sure you need it but if you do place here.
}
catch (IllegalArgumentException iae) {
System.out.println("Too many laps");
}
}
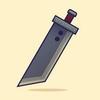
Allan Clark
10,810 PointsYou have an extra line in there. kart.drive(2); that was given as boiler plate code. Your try/catch syntax i perfect, just have an uncaught exception after it.

Noelle Acheson
610 PointsThank you Allan! You were right, the kart.drive(2) wasn't necessary...
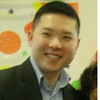
Youngchul Kim
4,258 PointsThis challenge seems to force you to use %s for the IllegalArgumentException variable. So in your case, you may need to further modify the print statement as follow (after revising your code as others mentioned above):
System.out.println("Too many laps %s", iae);

Noelle Acheson
610 PointsThank you Youngchul!
Noelle Acheson
610 PointsNoelle Acheson
610 PointsThanks so much! It helped! I got it!