Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial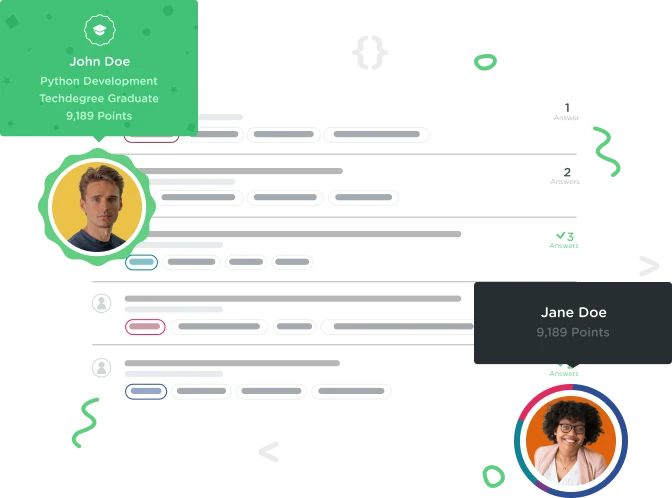
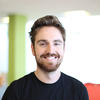
Kristian Woods
23,414 PointsI'm trying to insert records into my localhost database
Hello, I've created a database, and I'm trying to insert records via a form. However, the records aren't being sent to the database. I double checked the sql query by echoing it to the page, and it shows the statement as an empty string. I can't figure out why...
Sign up form:
<?php
include("inc/query.php"); <!-- DATABASE QUERY -->
?>
<!DOCTYPE html>
<html>
<head>
<title>Sign Up</title>
<link rel="stylesheet" type="text/css" href="main.css">
</head>
<body>
<div class="inner-form-bg">
<form action="welcome.php" method="post">
<h1 class="form-h1 sign-up-h1">Sign up!</h1>
<input type="text" placeholder="Username:" name="username" class="field-input req" required><br>
<input type="email" placeholder="Email:" name="email" class="field-input req" required><br>
<input type="password" placeholder="Password:" name="password" class="field-input req" required><br>
<button type="submit" class="submit field-input login-submit">Submit</button><br>
<button type="button" class="field-input new-acc-btn login-btn"><a href="index.php" class="create-acc back-to-login">Back to Login</a></button>
</form>
</div>
</body>
<footer>
</footer>
</html>
My database connection:
<?php
$dbhost = "localhost";
$dbuser = "root";
$dbpass = "root";
$dbname = "member_form";
$connection = mysqli_connect($dbhost, $dbuser, $dbpass, $dbname);
//Test database connection
if(mysqli_connect_errno()) {
die("Database connection failed: " .
mysqli_connect_error() . " (" .
mysqli_connect_errno() . ")"
);
}
?>
SQL statement:
<?php
//check for form submission
if($_SERVER['REQUEST_METHOD'] == 'POST') {
$errors = array(); // start array
//check for username
if(empty($_POST['username'])) {
$errors[] = "You forgot to enter your username";
} else {
$username = mysqli_real_escape_string($connection, trim($POST_['username']));
}
//check for email
if(empty($_POST['email'])) {
$errors[] = "You forgot to enter your email";
} else {
$email = mysqli_real_escape_string($connection, trim($POST_['email']));
}
//check for password
if(empty($_POST['password'])) {
$errors[] = "You forgot to enter your password";
} else {
$password = mysqli_real_escape_string($connection, trim($POST_['password']));
}
if(empty($errors)) {
include("inc/dbconnection.php");
$sql_signUp = "INSERT INTO login (username, email, passcode) VALUES('$username', '$email', SHA1('$password'))";
$result = mysqli_query($connection, $sql_signUp);
if($result && mysqli_affected_rows($connection) == 1) {
//message success
echo "success! 1 row affected";
} else {
die("Database query failed! No rows affected...");
}
}
}
?>
If this question isn't clear, just ask, and i'll try to explain in a different way
Thanks
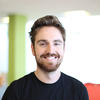
Kristian Woods
23,414 PointsYeah, I changed $_POST to the correct way. I then var_dumped $_POST['username'], and it returns the value entered into the field. I don't know why its not showing up in the query though
2 Answers

Umesh Ravji
42,386 PointsIs there somewhere your environment that you have turned error reporting off or something?
It looks like to me you are calling mysqli_real_escape_string() before you have included your connection script, so that method doesn't actually exist at that time.. and for some reason you are not being warned of this.
While I'm here, you don't have to escape the password because you are hashing it before you store it into the database (so you don't have to worry about it being database safe). I'd also like to bring up the sign up form, is it a PHP page or plain HTML?
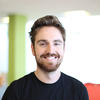
Kristian Woods
23,414 PointsHey, Umesh, I hadn't included my connection file properly. You were right. So, I changed it, and now it works properly. Thanks, man. I appreciate your help

Umesh Ravji
42,386 PointsThe obscure problems are always the fun ones :)
Umesh Ravji
42,386 PointsUmesh Ravji
42,386 PointsDid you try changing $POST_ to $_POST?
Try var_dump($POST_['username']) to see what it actually gets. My PHP version reports an error.