Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial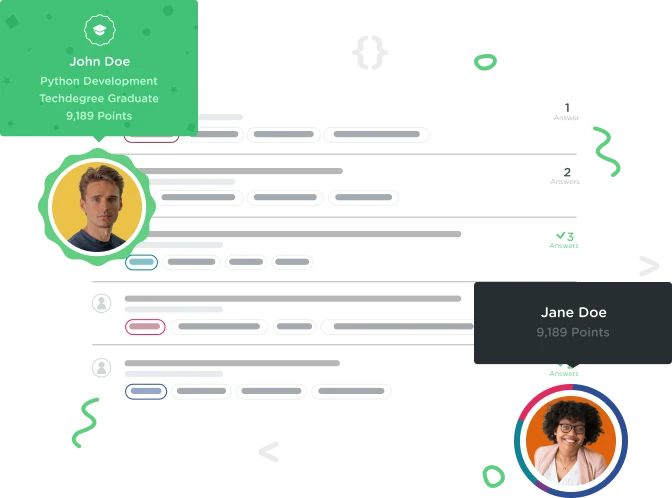

Chris Polito
1,028 Pointsi'm very confused
What am i doing?
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
try{
drive(9);
System.out.println("This should never happen");
} catch (IllegalArgumentException iae) {
System.out.println("Not enough battery remains");
}
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
2 Answers
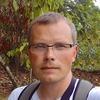
Ivan Kazakov
Courses Plus Student 43,317 PointsThis is a class describing an electric Go-Kart.
//It has 2 member variables:
private String mColor; //GoKart color
private int mBarCount; //GoKart current battery level
//a constant:
public static final int MAX_BARS=8; //which defines maximum battery capacity as 8 bars,
//and 6 methods:
drive() {
//drive 1 lap
}
drive(int laps){
//drive n-laps, each takes 1 bar of battery capacity
}
charge(){
//actually has a weird implementation in your example,
}
getColor() {
//returns GoKart's color
}
isBatteryEmpty() {
//returns whether the battery is empty, obviously
}
isFullyCharged() {
//returns whether the battery is fully charged
}
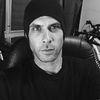
jack AM
2,618 PointsI see whats happening. The challenge is just asking you to write this logic in the "drive" method...,
--> if the current battery level is not empty, THEN go ahead and minus lap from mBarsCount.
--> else if mBarsCount is zero, then throw the IllegalArgumentException
(basically, if your battery is not yet empty, then you can do another lap. And you do another lap by allowing mBarsCount to subtract 1 from it. But if its 0, then you cant do another lap, so throw an error)
It would look something like this...
public void drive(int laps) {
if (!isBatteryEmpty()) {
...
} else {
throw new IllegalArgumentException("...");
}
}
or you can just as easily write it as...
public void drive(int laps) {
if (isBatteryEmpty()) {
throw new IllegalArgumentException("...");
} else {
...
}
}