Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial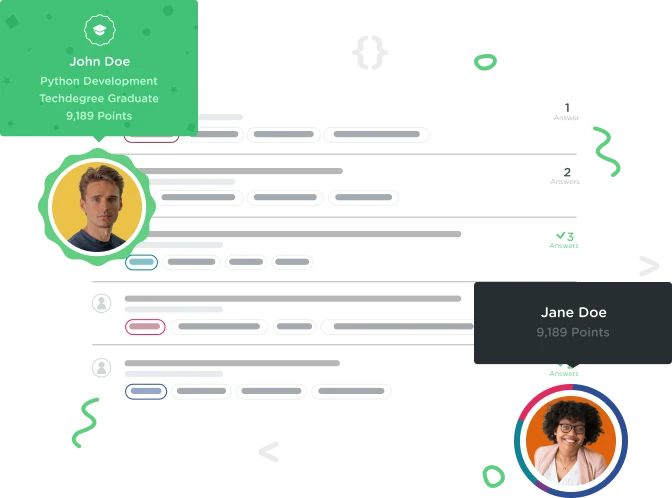

Pieter Bracke
4,078 PointsImage file upload not working properly
Hi, I am working on a login system which is working pretty good already. Now I decided to add the feature that on registering, a user is able to upload an image to set as his profile picture. My code is mostly done but it doesn't seem to work and I can't figure out why. In the code below I made 2 if statements regarding the image, first I am trying to check if the image is an actual image file and after that I am copying the uploaded image to a folder in my directory, the path gets stated in $avatar when the picture is uploaded and the form gets submitted. When I upload a picture I manage to pass the first if statement but then it gives me the else statement that the file did not got uploaded. When I try to upload a different extension then the ones which are allowed I get no error at all and the script returns a blank screen, no user data gets posted on any of these occasions.
<?php session_start(); ?>
<div class="popupscreen" id="registerpopup">
<div class="formwrapper">
<div class="login-form">
<form action="includes/register.php" method="POST" enctype="multipart/form-data">
<label class="popuplabel">First-name</label>
<input name="firstname" placeholder="first-name" class="popupinput" required />
<label class="popuplabel">Last-name</label>
<input name="lastname" placeholder="last-name" class="popupinput" required />
<label class="popuplabel">Email</label>
<input name="email" placeholder="email" class="popupinput" required />
<label class="popuplabel">Username</label>
<input name="username" placeholder="username" class="popupinput" required />
<label class="popuplabel">Password</label>
<input name="password" placeholder="password" class="popupinput" required/>
<label class="popuplabel">Upload profile picture</label>
<input type="file" name="avatar" accept="image/*" />
<button class="popupbutton" type="submit">Register</button>
</form>
<button onclick="closeregister()" class="popupbutton">Close</button>
</div>
</div>
</div>
<?php
require_once('../connect.php');
if(isset($_POST) AND !empty($_POST)){
$firstname = mysqli_real_escape_string($connection, $_POST['firstname']);
$lastname = mysqli_real_escape_string($connection, $_POST['lastname']);
$email = mysqli_real_escape_string($connection, $_POST['email']);
$username = mysqli_real_escape_string($connection, $_POST['username']);
$password = password_hash($_POST['password'], PASSWORD_DEFAULT);
$avatar = mysqli_real_escape_string($connection, 'avatars/'.$_FILES['avatar']['name']);
$extension = pathinfo($avatar, PATHINFO_EXTENSION);
if(in_array($extension, array('jpg', 'png', 'jpeg'))){
if(copy($_FILES['avatar']['tmp_name'], $avatar)){
$sql = "INSERT INTO `login` (Firstname, Lastname, Email, Username, Password, Avatar) VALUES ('$firstname', '$lastname', '$email', '$username', '$password', '$avatar')";
$result = mysqli_query($connection, $sql);
if($result){
$url = "../index.php";
$messageok = "User registration succesfull!";
echo "<script type='text/javascript'>alert('$messageok');</script>";
echo '<script>window.location = "'.$url.'";</script>';
}else{
$url = "../index.php";
$messagenok = "User registration failed!";
echo "<script type='text/javascript'>alert('$messagenok');</script>";
echo '<script>window.location = "'.$url.'";</script>';
}
} else{
$url = "../index.php";
$messageok = "Picture was not uploaded to database! plz try again";
echo "<script type='text/javascript'>alert('$messageok');</script>";
echo '<script>window.location = "'.$url.'";</script>';
}
}else{
$url = "../index.php";
$messageok = "Uploaded images must have one of following extensions, jpg, jpeg, png. Please try again!";
echo "<script type='text/javascript'>alert('$messageok');</script>";
echo '<script>window.location = "'.$url.'";</script>';
}
}?>
2 Answers

Fletcher Henneman
3,130 PointsI got the script to work on my end by using the
move_uploaded_files()
function rather than the copy()
function.
So, this line (#16):
if(copy($_FILES['avatar']['tmp_name'], $avatar)){
Should be:
if(move_uploaded_file($_FILES['avatar']['tmp_name'], $avatar)){
That should work, it you have any other errors comment or message me.
Here is the Connect.php script I used, along with the SQL table.
Connect.php
$servername = "localhost";
$username = "root";
$password = "";
// Create connection
$connection = new mysqli($servername, $username, $password, 'imgupload');
// Check connection
if ($connection->connect_error) {
die("Connection failed: " . $connection->connect_error);
}
echo "Connected successfully";
?>
SQL Pastebin Link

Fletcher Henneman
3,130 PointsAfter escaping all of the form fields, you can manually update the Session Variables.
$_SESSION['Firstname'] = $firstname;
$_SESSION['Lastname'] = $lastname;
$_SESSION['Email'] = $email;
Is that what you are looking for, or do you want to accomplish something different?

Pieter Bracke
4,078 PointsPerfect , I was looking to far on this one trying to set a whole new session, but this simple solution is great thanks a lot!

Fletcher Henneman
3,130 PointsCould you mark this question as answered please? And no problem have a good day.
Pieter Bracke
4,078 PointsPieter Bracke
4,078 Pointsyeah that did the trick :). But I am already having another little problem. Today I was working on an update script so my users could update their own data, This is finished and working, however one little problem the session variables don't refresh immediatly since the session gets set when they login. So to see the effect my user needs to login and logout how could I fix this? See code below: