Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial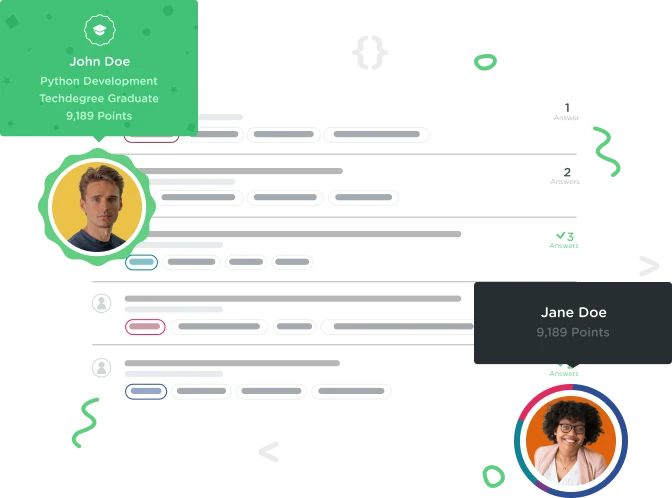

Alexander Nowak
498 PointsImplementing challenge
Okay, so let's use our new isFullyCharged helper method to change our implementation details of the charge method. Let's make it so it will only charge until the battery reports being fully charged. Let's use the ! symbol and a while loop. Inside the loop increment mBarsCount.
HI guys, I am really struggling with this question, I don't fully understand the video, I have watched it many times but its not clicking for me. Could somebody show me the answer to this questions and explain why thats the answer?
Thank you so much!
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_ENERGY_BARS;
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
while (mBarsCount ) {
}
}
}
3 Answers

Shezan Kazi
10,807 PointsOr even better:
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
}

Shezan Kazi
10,807 PointsHi Alex,
try something like this:
while (mBarsCount ! MAX_ENERGY_BARS) {
mBarsCount++;
}

Shezan Kazi
10,807 PointsYou always use void if the function does not return a value. Since charge only "checks" and increments there is no return value.
Shezan Kazi
10,807 PointsShezan Kazi
10,807 PointsBasically you want to check if the Battery is fully charged. This is what the method isFullyCharged checks.
The method charge checks if the battery still has to be charged. This is the case if the battery isNotFullyCharged. isNotFullyCharged is the same as !isFullyCharged. Just as for example "true" is the same as "!false" or notRed is the same as !Red. Is that clear to understand?
Alexander Nowak
498 PointsAlexander Nowak
498 PointsHi Shezan. Yes that makes perfect sense thank you :) Why do we need the term 'void' in 'public void charge() {
Thank you!