Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial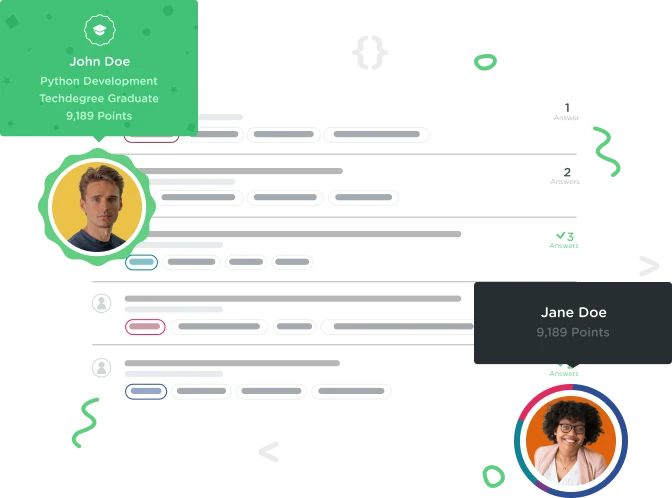

Heather Stone
5,784 Pointsincorrect contact.rb code
Hi, I'm on the Building an Address Book section of the Learn Ruby course and trying to debug some of my code. When I run contact.rb, I get the error message "undefined local variable or method "addresses". I checked my code and everything seems ok...the structure of that block mirrors the phone_number block. Any suggestions? Thank you!
require "./phone_number"
require "./address"
class Contact
attr_writer :first_name, :middle_name, :last_name
attr_reader :phone_numbers, :address
def initialize
@phone_numbers = []
@address = []
end
def add_phone_number(kind, number)
phone_number = PhoneNumber.new
phone_number.kind = kind
phone_number.number = kind
phone_numbers.push(phone_number)
end
def add_address(kind, street_1, street_2, city, state, postal_code)
address = Address.new
address.kind = kind
address.street_1 = street_1
address.street_2 = street_2
address.city = city
address.state = state
address.postal_code = postal_code
addresses.push(address)
end
def first_name
@first_name
end
def middle_name
@middle_name
end
def last_name
@last_name
end
def first_last
first_name + " " + last_name
end
def last_first
last_first = last_name
last_first += ", "
last_first += first_name
if !@middle_name.nil?
last_first += " "
last_first += middle_name.slice(0, 1)
last_first += "."
end
last_first
end
def full_name
full_name = first_name
if !@middle_name.nil?
full_name += " "
full_name += middle_name
end
full_name += " "
full_name += last_name
full_name
end
def to_s(format = "full_name")
case format
when 'full_name'
full_name
when 'last_first'
last_first
when 'first'
first_name
when 'last'
last_name
else
first_last
end
end
def print_phone_numbers
puts "Phone Numbers"
phone_numbers.each { |phone_number| puts phone_number }
end
def print_addresses
puts "Addresses"
addresses.each { |address| puts.address.to_s('short') }
end
end
2 Answers
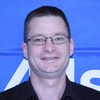
Seth Reece
32,867 PointsIn your def initialize try @addresses instead of @address.

John Kuzda
32,314 PointsOn line 6 your have : attr_reader :phone_numbers, :address It should be: attr_reader :phone_numbers, :addresses
And in your def initializer - on line 9 you have: @address = [] It should be: @addresses = []
That should take care of it!