Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial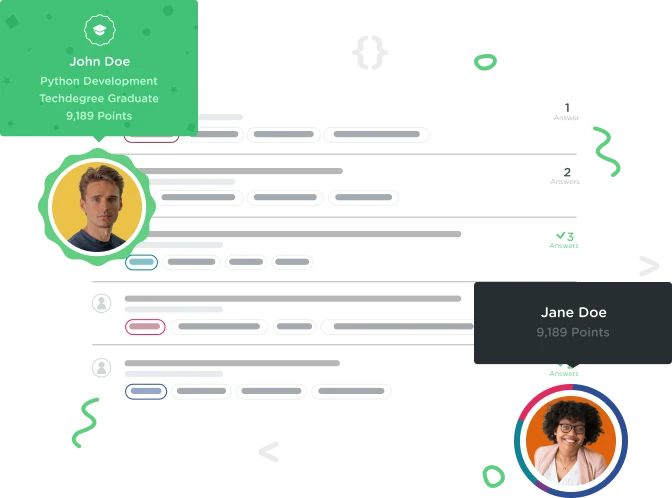
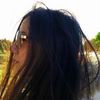
Vane Aroki
4,693 PointsInstance and class methods
I'm a bit confused about the differences between instance and class methods. Can someone help me please? Thank you :)
2 Answers
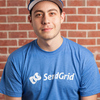
Dylan Shine
17,565 PointsHi Vane,
The difference between class methods and instances methods can be characterized with this example:
Pretend I have Class, Cat, that has the following method definitions:
class Cat
attr_reader :name
def initialize(name)
@name = name
end
def self.catNamedCharles
self.new("Charles")
end
def meow
puts "Meow"
end
end
cat = Cat.new("Dylan")
cat2 = Cat.catNamedCharles
puts cat.name # => "Dylan"
puts cat2.name #=> "Charles"
cat.meow #=> "Meow"
cat2.meow #=> "Meow"
I define three methods for my Cat class, the initialize method, a class method, self.catNamedCharles, and an instance method, meow.
Firstly notice how I prefix the class method with the word "self" in its definition. This tells the Ruby interpreter that it is a class method and should be called with the class. All this class method is doing is calling the classes "new" method and presetting the name to "Charles". When I instantiate cat2, I am simply assigning the return value of the Cat's class method, which in this case returns an instance of the class.
An instance method on the other hand is a method that an instance can call. Both cat and cat2 are instances of the Cat class, and therefore inherit the method, meow. I can call meow on either instance and for both cat objects they will puts "Meow".
In short, a Class method is a method you directly call on the class, prefixing it's method definition with the word self, while an instance method is a method you call on an individual instance of a class.
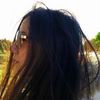
Vane Aroki
4,693 PointsAwesome explanation! Thank you very much Dylan :)