Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial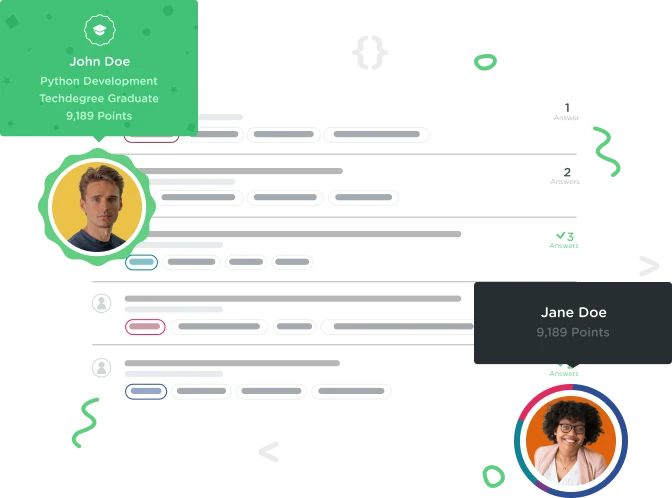
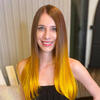
Alissa Kennedy
9,667 PointsIntegrating PHP with Databases / Fetching Many Relationships / Code Challenge Help
Question: $item is an array that contains details about the media item. $results is a PDOstatement object with our genre results. Fetch the associative array of $results to loop through, and add an additional "genres" array element to $item. This "genres" element should be an internal associative array with the key of genre_id and the value of genre.
Example $item array:
array(8) {
...
["genres"]=>
array(2) {
[3]=>
string(6) "Comedy"
[16]=>
string(6) "Sci-Fi"
}
}
So far, I have tried this:
<?php
include "helper.php";
/*
* helper contains the following variables:
* $item is an array that contains details about the library item
* $results is a PDOstatement object with our genre results.
*/
$item = $results->fetch(PDO::FETCH_ASSOC) {
["genres"]=>
array(2) {
[3]=>
string(6) "Comedy"
[16]=>
string(6) "Sci-Fi"
}
}
?>
I'm getting an error Bummer! syntax error, unexpected '=>' (T_DOUBLE_ARROW) in index.php on line 11
I've been stuck on this one for a bit, would appreciate any help! Thanks
2 Answers
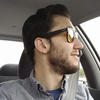
Benjamin Larson
34,055 PointsI feel like this is hard in large part because the problem setup and instructions are confusing. I'll try give some hints so as to not simply post the answer.
The example code in the teacher's notes of the previous video will be helpful as a guide:
<?php
while ($row = $results->fetch(PDO::FETCH_ASSOC)) {
$item[$row["role"]][] = $row["fullname"];
}
You'll need to loop through $results, keeping in mind that since you are using fetch, it will return the next row of results until there are no more rows. To capture and be able to "use" each row, you'll have to assign the associative array returned by
$result->fetch()
into a new variable, such as$row
.Inside the loop, you'll assign the value of the genre associated with the given row into a multi-dimensional array on $items["genres"][the genre_id associated with the given row].
You can var_dump($items) and hit the Preview button to see if the array elements are corresponding with the example $item array that is supplied at the top of the challenge.
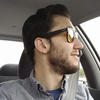
Benjamin Larson
34,055 PointsInside the $item['genres'] array, you want to end up with a nested array where the key is the $row['genre_id']
and the value is $row['genre']
.
A 2-dimensional array like this needs to have 2 indices, structured like `$items[][], but the second index in this example will contain an array value, thus introducing more brackets. It might be easier to visualize if it were put into a variable:
<?php
$genreID = $row['genre_id'];
$item['genres'][$genreID]
Sorry, I'm struggling to convey ideas for this one without going into lengthy descriptions. Concepts like multidimensional arrays are tougher to explain with simple text.
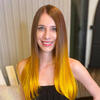
Alissa Kennedy
9,667 PointsThanks so much for all the help, I understand it a bit better.
I ended up getting this to work (from another thread)
$item["genres"][] = $row["genre_id"] = $row["genre"];
I'm not totally sure how it differs from the lines you shared. I probably need to go back and review the easier PHP a bit before going on.
Thanks again.
Alissa Kennedy
9,667 PointsAlissa Kennedy
9,667 PointsThanks for the pointers! I think I'm much closer now.
<?php
include "helper.php"; /*
while ($row = $results->fetch(PDO::FETCH_ASSOC)) { $item["genres"["genre_id"]][] = $row["genre"]; }
var_dump($item);
?>
I'm getting the error Bummer! Doesn't look like added anything to the $item["genres"] array.
Does this mean there is an issue with my fetch?
Should I also be calling $row on the first part of my internal associative array?
I'm not totally sure where all the variables go. It looks like I need "genre", "genre_id" and "genres"
Any more hints would be greatly appreciated! Thank you