Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial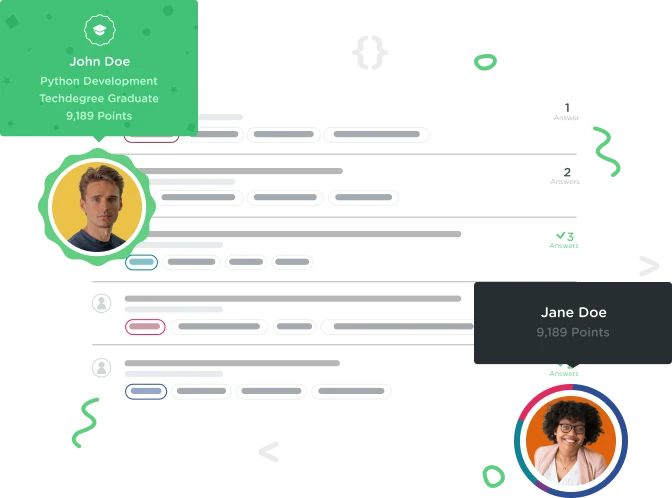

Itsik Dangoor
Front End Web Development Techdegree Student 5,330 Pointsis it better to declare the char display variable outside the for each loop?
hi everybody,
two Q's:
it is better to declare the display variable outside the for each loop? that way the JVM(I guess) won't create each interation new variable with the same name?
by the way what is happening with the "old" variable when we declaring new variable in each loop with the same name?
public String getCurrentProgress() {
String progress = "";
char display;
for (char letter : mAnswer.toCharArray()) {
display = '-';
if (mHits.indexOf(letter) >= 0) {
display = letter;
}
progress += display;
}
return progress;
}
thank you
3 Answers
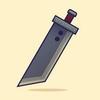
Allan Clark
10,810 PointsThe variable does not need to be declared outside of the for each loop because it is remains the same and does not need to persist after the loop is over. That being said the difference is negligible.
The same thing that happens with all variables that have reached the end of their scope, it is dereferenced and junked by the JVM. When the next loop starts up a new copy of the variable is created and stored in memory.
If you wanted to cut down on the repeated variable junking and remaking you could declare and set the value of the variable outside the loop. This would however require a tweek to the structure of the method. This solution should give you the same results without the need for creating or setting that variable each time.
public String getCurrentProgress() {
String progress = "";
char display = '-';
for (char letter : mAnswer.toCharArray()) {
if (mHits.indexOf(letter) >= 0) {
progress += letter;
} else {
progress += display;
}
}
return progress;
}
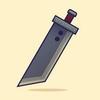
Allan Clark
10,810 Points2 The same thing that happens with all variables that have reached the end of their scope, it is dereferenced and junked by the JVM. When the next loop starts up a new copy of the variable is created and stored in memory.
So yes machine cycles are taken up by the JVM repeatedly going through dereferencing and junking the variable. The difference is negligible because the variable will always be at the top of the stack, popped off and recreated, something the JVM is designed to do very quickly. Also it doesn't take up more memory because it will get assigned the same memory slot each time. You would have to be recreating a ton of variables, or have bigger optimization issues elsewhere for it to become an issue.
The example I provided was to show you how this particular for-each loop could be structured to prevent having to repeatedly junking the variable. In certain situations this restructure will not be possible.

Itsik Dangoor
Front End Web Development Techdegree Student 5,330 Pointshi, thank you for the replay.
but my real question is not about the logic programming aspect but really what is going on the hardware side. if we are taking this code to a bigger system scenario, imagine it. when variable is been created over and over again inside the loop is it taking more time and other resources if we would have done it outside the loop?
Adiv Abramson
6,919 PointsAdiv Abramson
6,919 PointsSeems like the display variable always equals '-'. If so, then shouldn't it be declared as a constant with the keyword final, e.g.
private final char display = '-'; ?
Thank you
Allan Clark
10,810 PointsAllan Clark
10,810 PointsIt certainly can be a constant since it does not change. It doesn't need an access modifier (private or public) because it is not a member variable. So the declaration would look like this:
It doesn't necessarily need to be a constant because the variable has such a short lifespan (it is junked at the end of the method). However if you have a large complex method that does a lot of changing variables, declaring it a constant will make sure it is not changed.