Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial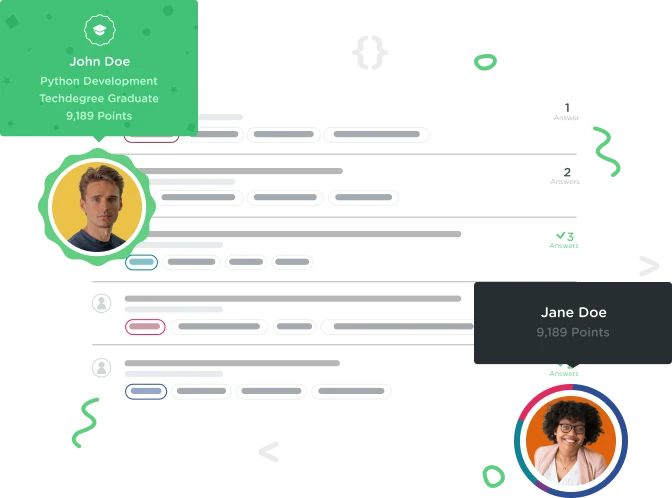

robert toole
4,587 PointsIs it preferred to use a for loop here instead of the more recently learned for in loop?
I completely understand the lesson and the answer, but I am slightly confused as to why a standard for loop was used in the solution. Of course there is more than one correct answer and using a for loop works ( as seen in the solution video ). Why not use the more recently used 'for in' loop to further expand on the immediately previous lessons? I'm sorry if this sounds like I prefer once answer or want to prove myself right as that is not the case and I was more wondering if the 'for in' was not used here because the standard for loop is preferred in most cases?
Also if this helps for context this is the code I used to achieve the same results:
for ( let prop in petObjects ) {
html.innerHTML +=
`
<h2>${ petObjects[ prop ].name }</h2>
<h3>${ petObjects[ prop ].type } | ${ petObjects[ prop ].breed }</h3>
<p>Age: ${ petObjects[ prop ].age }</p>
<img src="${ petObjects[ prop ].photo }" alt="${ petObjects[ prop ].breed }">
`
}
1 Answer

Shawn Jones
15,579 PointsFor "in" loops are used to loop over keys in an object whereas traditional for loops are used to loop over items in an array.
Let's say I had an object where I wanted to print out the keys and values of the object. I only have one object, not multiple. In this case, I would use a "for in" loop because I can loop over each key that exists in the object and format how it's printed like so:
let person = {
firstName: "Shawn",
lastName: "Jones"
}
// print every key in the object without calling every key
for (prop in person) {
console.log(`${prop}: ${person[prop]}`);
}
Printed to the console:
firstName: Shawn
lastName: Jones
With the example you posted, It's better to use a traditional for loop because I don't want an individual key/value pair every iteration, I want the entire object. Also, I imagine the element you were looping over is an array of objects, not a single object with multiple keys. Let's take the example I made above and make multiple person objects and throw them into an array:
people = [
{firstName: "Shawn", lastName: "Jones"},
{firstName: "Maria", lastName: "Jones"}
];
Now, in order to access keys and print out their values, I need to get to the individual object first. The traditional for loop or the new for "of" loop is best for this:
for (let i = 0; i < people.length; i++) {
console.log(`firstName: people[i].firstName}`);
console.log(`firstName: people[i].lastName}`);
}
// or use "for of" loop
for (let person of people) {
console.log(`firstName: person.firstName}`);
console.log(`firstName: person.lastName}`);
}
Sidnote: you can try and combine the "for in" loop and the "for" loop of your choosing if it makes sense for the problem you're trying to solve:
for (let person of people) {
for (prop in person) {
console.log(`${prop}: ${person[prop]}`);
}
}
I hope that helps!
robert toole
4,587 Pointsrobert toole
4,587 PointsThank you for taking the time to explain traditional for and for 'in' standard use convention. This cleared up why the instructor did not use the for 'in' in the solution video as you were correct in assuming it was an array of objects. Thanks again Shawn this helped a lot.