Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial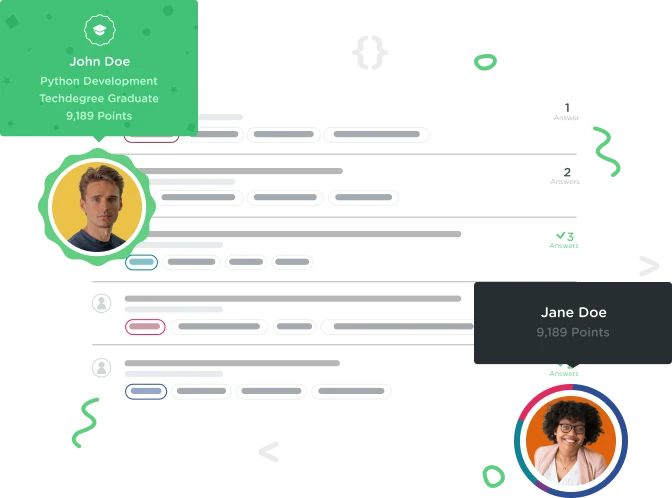
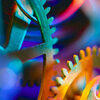
ja5on
10,338 Pointsis not a function error
headingColor.addEventlistener("click", function(){
document.getElementById("header").style.color ="blue";
});
The html button is:-
<h1 id="header">List</h1>
<button id="headingColor">Change Color</button>
I just can't find out why it wouldn't work,
5 Answers
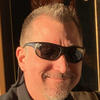
Peter Vann
36,429 PointsThere is no document.getElementsById. (Plural - which would suggest retrieving a collection of items. but ids should always be unique to a page.)
Its document.getElementById (Singular, NO "s" - Targets exactly one element only with that ID)
On the other hand, getElementsByClassName (Note: plural) works because you can have multiple elements with the same class name, thus you do want to retrieve a collection of elements. (And to be clear, getElementsByClassName will only retrieve one element, if there is only one element with that class name. But it still returns a collection (size: one element), so to use it you would still need to use it like this to target that element: getElementsByClassName('myUniqueClass')[0].
([0] being the first element in the targetted collection.)
And, of course, if your page/DOM has 3 elements with the class 'myAwesomeClass' to target the thrid element with that class name you would use: getElementsByClassName('myAwesomeClass')[2]
([2} being the third element in the targetted collection.)
Much like querySeletor targets only one element (the first one found).
But querySelectorAll will return a collection of elements.
Again, understanding/pinpointing such errors gets easier over time.
I get tripped up typos (bouncing a key or just typing essentially slightly dyslexically - sometimes my fingers respond to my brain's commands in the wrong order!?! LOL) and missing semicolons or forgetting a curly brace - often it is caused by copying and pasting code and forgetting to completely edit the pasted code properly - that happens to me ALL THE TIME!?!
Keep this in mind (because I mix this up all too often): with getElementById, you don't need the pound sign to target an id, just the name. But with querySeletor you do need the pound sign because it can target ids, classes, descendants, etc., so you have to be more specific.
Does that make sense?
Don't give up. I HATED JavaScript when I first started coding. I was used to Visual Basic, which was much easier to debug. Now I really enjoy it (JS, that is...). (VB had IntelliSense, so you could very easily step through code and mouse over variables to see values in real-time to catch errors.)
And as a result of toughing it out with JavaScript, hewever, I now am an expert at "attention to detail" (as tested on/by LinkedIn) because I have learned over time, getting your code exactly right the first time can save you a lot of frustrating debugging in the long run - so I really pay close attention to the details as I go.
I still make errors, but I can identify them way quicker now than I could years ago. I know better what, in general, to look for (what common and therefore likely errors are.)
And to be honest, I'd be LOST without Grammerly, actually - it catches A LOT of my typos!?!
I suggest getting comfortable with relying on the console in dev tools to help you debug - it can really help identify the source of an error.
Again, it will all get much easier as you progress and gain experience.
I hope that helps.
Stay safe and happy coding!
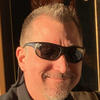
Peter Vann
36,429 PointsHi jas0n!
With pure vanilla JS - yea -that's pretty much how you would do it (to change an element's color by clicking a button, that is).
You could shorten the syntax a little by using JQuery though.
So with JQuery the code would be:
$('#headingColor').click(function() {
$('#header').css('color', 'blue');
});
You could also use on() instead of click():
$('#headingColor').on('click', function() {
$('#header').css('color', 'blue');
});
(Still JQuery, of course)
And of course, to use JQuery, you have to include the library in your project - so even though the code is a little more verbose, sometimes it's less of a hassle to just use vanilla JavaScript.
More info:
https://www.w3schools.com/jquery/default.asp
You could also shorten your JS by creating a function (to target and update the CSS) in JS and then use onclick in the HTML to trigger the function.
Something like this:
<!DOCTYPE html>
<html>
<body>
<header id="header">Color Change Test</header>
<input type="button" value="Click Me" onclick="makeElementColorBlue('header');">
<script>
const makeElementColorBlue = function(ID){
document.getElementById(ID).style.color = "blue";
}
</script>
</body>
</html>
Or this:
<!DOCTYPE html>
<html>
<body>
<header id="header">Color Change Test</header>
<input type="button" value="Click Me" onclick="makeElementsColorBlue('#header');">
<script>
const makeElementsColorBlue = function(ID){
document.querySelector(ID).style.color = "blue";
}
</script>
</body>
</html>
I hope that helps.
Stay safe and happy coding!
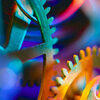
ja5on
10,338 PointsOh I'm only going through JS at the mo, but thanks, studying JS with the DOM. I do follow the Vids but I'm playing around with my codes.
Happy codes :-)
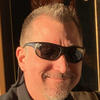
Peter Vann
36,429 PointsI added some possible HTML syntax, too...
;)
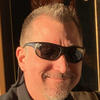
Peter Vann
36,429 PointsOver time you will get more finesse taking more verbose JS code and encapsulating it in your own functions to drastically shorten the syntax in the main parts of your app...
(And obviously, you can make much shorter function names - I made mine long for clarity!?!)
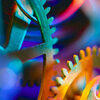
ja5on
10,338 Pointsconst myHeader = document.getElementById("myHeader");
const myButton = document.getElementById("myButton");
const myButton2 = document.getElementsById("myButton2");
myButton.addEventListener("click", function(){
myHeader.style.color = inputColor.value;
});
myButton2.addEventListener("click", function(){
document.getElementsByClassName("healthyFoods").style.fontsize = "20px";
});
I keep getting a Uncaught TypeError: document.getElementsById is not a function???
Its so disheartening everything i do is always errors
ja5on
10,338 Pointsja5on
10,338 PointsSo my bad.... I found this out with w3schools....
document.getElementById("headingColor").addEventListener("click", function(){
document.getElementById("header").style.color = "blue";
});
This now works but seems really long code just to change a heading color using a button or is it the best way?
I know it can be done with CSS way easier but i'm playing around with javaScript to help me learn :-)