Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial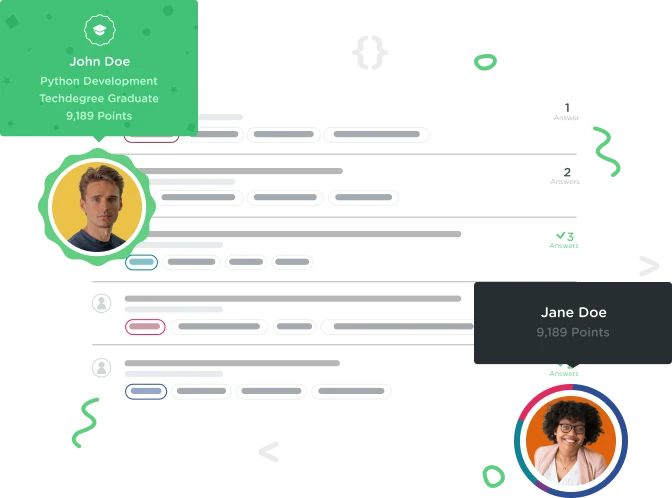

aaron quasius
260 PointsIs this a joke?
What is this even? I got it to print what it wants but then it tells me it was expecting a quote from patrick star? What
using System;
class Program
{
static string Quote(string phrase)
{
return ("\"When you learn, teach. When you get, give\"");
}
// YOUR CODE HERE: Define a Quote method!
static void Main(string[] args)
{
// Quote by Maya Angelou.
Console.WriteLine(Quote("When you learn, teach. When you get, give."));
// Quote by Benjamin Franklin.
Console.WriteLine(Quote("No gains without pains."));
}
}
10 Answers
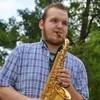
Broderick Lemke
13,483 PointsThe challenge states: Define a Quote method that accepts a string parameter. It should return that same string, but surrounded by double quotes. For example, Console.WriteLine(Quote("When you learn, teach. When you get, give.")) should print "When you learn, teach. When you get, give." (Note that the output is surrounded by quotes.)
The first sentence tells us to define a quote method that accepts a string parameter. We can do that as such:
static string Quote(string phrase){
}
The second sentence tells up to return the same string but surrounded by double quotes. We can go ahead and add this functionality by returning a double quote surrounding the phrase we pass in as a parameter.
static string Quote(string phrase){
return '"' + phrase + '"';
}
Is we continue reading they give us a test case that we can use to test. If we call the quote method with Quote("When you learn, teach. When you get, give.")
we should receive back the text "When you learn, teach. When you get, give."
. Note that is has the extra quotation marks around it. If we had just returned the phase like this:
static string Quote(string phrase){
return phrase;
}
Then we would get back the string When you learn, teach. When you get, give.
Notice there are no quotation marks.
This brings up a big point in programming: Testing. As I mentioned, they gave us a test case. This is a scenario where we know the results that we should get. We know if we pass in the string When you learn, teach. When you get, give.
we will get the string "When you learn, teach. When you get, give."
. We can also develop our own test cases. For example if I pass in the quote Aspire to inspire before we expire.
I can expect "Aspire to inspire before we expire."
to be returned. By following our logic in the function, I can see we will get it back. This helps to ensure we build our programs correctly. In Treehouse's testing to see whether you answer is correct or not, they are using a test case by calling the function Quote("Patrick, I don't think wumbo is a real word.")
and expecting to receive "Patrick, I don't think wumbo is a real word."
.
There error messages were not very helpful in explaining what is going wrong. In the real world we would add multiple test cases to this statement. For example we'd check that we get the Patrick Star quote, but we'd also test it with another quote to make sure that the value couldn't just be hard-coded. It seems to be an oversight in the testing, but hopefully this clears it up a bit.

KRIS NIKOLAISEN
54,972 PointsWhat does this mean to you?
It should return that same string
You are to return whatever the checker passes in only surrounded by double quotes. That is what the parameter is for. One way to do this is:
static string Quote(string phrase)
{
return '"' + phrase + '"';
}

aaron quasius
260 PointsYou aren't understanding what I'm saying. NO WHERE IN THE DESCRIPTION DOES IT ASK FOR YOU TO RETURN A QUOTE FROM PATRICK STAR. It literally asks you to return Maya Angelou's quote. Which I did and it told me I did in the same line it tells me I'm wrong because they were expecting "Patrick I don't think wumbo is a real word" . So without changing a single line of code that I initially presented you, I simply deleted maya Angelou's quote and replaced it with the stupid Patrick star one the bummer message said it was expecting and it said it was complete and I got the points for it. So I don't really understand what you're trying to get at here. All I'm saying is this quiZ is terrible. It tells you to return something that they don't provide the info for.

KRIS NIKOLAISEN
54,972 PointsYour method returns "When you learn, teach. When you get, give" regardless of what is passed in. It's telling you that 'Patrick, I don't think wumbo is a real word.' was passed in and '"Patrick, I don't think wumbo is a real word."' should be returned.
"When you learn, teach. When you get, give" is just an example - not what is being tested.

aaron quasius
260 PointsLol the challenge is literally to define a quote method that accepts a string parameter. No where in their example does it ask for me to return anything about Patrick star. It tells me it should print "When you learn, teach. When you get, give." In double quotes. It states nothing about them expecting a quote from Patrick star.

aaron quasius
260 PointsSo how am I supposed to know that's what they want returned when in the method itself the two quotes presented as are one from Maya Angelou and Benjamin franklin. It doesn't state anywhere that it wants me to return Patrick stars quote until you check your work and the bummer message comes up.

aaron quasius
260 PointsAnd I literally just completed the challenge by typing return (\""Patrick, I don't think wumbo is a real word."\") how is one supposed to know that the answer is this when they ask you to return Maya Angelou's quote. How stupid

KRIS NIKOLAISEN
54,972 PointsI understand what you are saying. It literally says For example, when providing the Maya Angelou quote. That is just an example of what is returned when 'When you learn, teach. When you get, give.' is passed in. However for the challenge that is not the quote being passed in.
If 'xyz' is passed in then return '"xyz"'. If 'Patrick, I don't think wumbo is a real word.' is passed in then return '"Patrick, I don't think wumbo is a real word."' That's what 'It should return that same string' means in the description.

Tomas Schaffer
11,606 PointsHi
At first you are returning static string from method Quote and trying enter dynamicly the same string by invoking it in Main method. Which cannot work. You are entering value to argument parameter but never use it. Probably treehouse using Xunit for testing, this is reason why you have to return value dynamicly. I believe this Patrick sentence is just some funny way to say you are returning some value but not the right one.
I did it in this way and it works.
static string Quote (string phrase) { return ('"'+ phrase +'"'); }
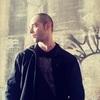
David Franco
3,252 PointsSpoiler Alert:
static string Quote(string quote) { return ("\"" + quote + "\"" ); }

harrison king
2,511 Pointsye

Jack Ernsberger
2,479 Pointshey harrison lmao

Giovanny De Jesus
9,662 PointsThis is poorley worded. Is not that one doesn't understand the concept Is that one gets stuck trying to figure out what's being asked.

Matt Fredericks
8,367 PointsThis exercise is garbage

KRIS NIKOLAISEN
54,972 PointsYou need to use the phrase
parameter to return what is being passed in to the Quote method surrounded by double quotes.

aaron quasius
260 PointsWhy does it tell me it printed out correctly? But then it says they were expecting it to print "Patrick, I don't think wumbo is a real word."
Tomas Schaffer
11,606 PointsTomas Schaffer
11,606 PointsHi
At first you are returning static string from method Quote and trying enter dynamicly the same string by invoking it in Main method. Wich cannot work. You are entering value to argument parameter but never use it. Probably treehouse using Xunit for testing, this is reason why you have to return value dynamicly. I believe this Patrick sentence is just some funny way to say you are returning some value but not the right one.
I did it in this way and it works.
static string Quote (string phrase) { return ('"'+ phrase +'"'); }