Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial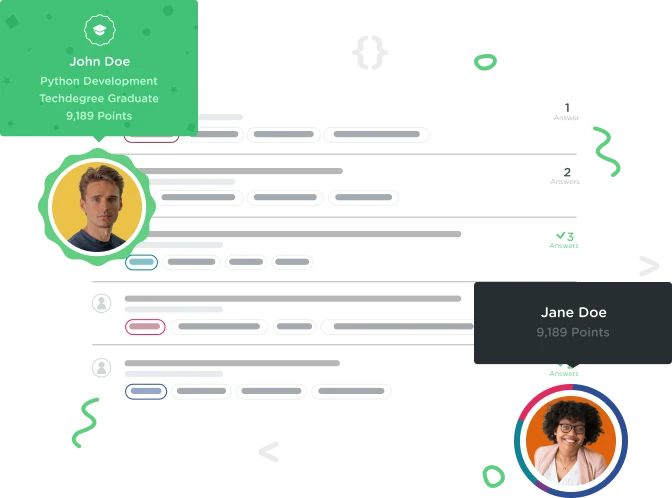
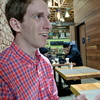
Leyton Parker
6,556 PointsI've completely stuck here and very frustrated. How can I call the function if it has no name?
I was stuck on step 1 of this code challenge and found the thread that gave the answer to it, when I wrote the code identically I still received a parse error. Only by copying and pasting directly from the thread was I able to move on.
- I don't understand how var max = function is naming the function 'max'
- I'm not sure how to call the function because max(); does not work (Task 1 no longer responding)
Please help. I was doing fine and now JavaScript just became very confusing.
var max = function(one, two) {
if (one > two) {
return one;
} else {
return two;
}
}
max();
alert( max(one, two) );
6 Answers
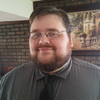
Michael Davidson
5,519 PointsHeya Leyton!
Okay so let me take a shot at your questions here. Let me know if I need to go into more detail.
To question#1:
When you say
var max = function(one, two) {
if (one > two) {
return one;
} else {
return two;
}
};
You're declaring a anonymous function, that is, a function that has no name. Instead of naming the function, you're assigning that function to the variable max.
So like if you did: var max = 1;
The browser would realize when you typed max in, it would from then on be interpreted as 1. In the same way if you type in max in your example, the browser knows you want to call your function.
And for question #2, your code works. Its fine, here is your issue.
In the last part of your code you have
max();
alert( max(one, two) );
By just putting in one, two, you're entering strings, so it won't parse right, try doing
max();
alert(max(1, 2))
Also I'm not sure why you're putting max() before the alert, all calling max before the alert would do is have it return the greater of the two numbers, but as you're not doing anything with that return, it just kinda sits there. No big deal it doesn't hurt anything, just wanted you to know.
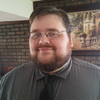
Michael Davidson
5,519 PointsHmmm... I'm not quite sure what you're asking, but I'm going to give it a shot, if this sounds completely left field, let me know I might be answering the wrong thing.
When you first write it out, the function itself
var max = function(one, two) {
if (one > two) {
return one;
} else {
return two;
}
};
the function(one, two) are the arguments you will pass to the function, this just tells the computer, when I call this function, it'll have 2 arguments that come with it.
So when you call max (the function) you'd pass what you want to see is larger, in example max(1, 2)
These numbers take the place of the arguments and it goes through your code at that point.
The strings in the function itself shouldn't matter, as they're stand-ins for the data you'll eventually pass to it.
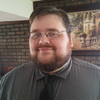
Michael Davidson
5,519 Pointsmessages got mixed up : reposted response below.

Jesse Lawson
11,159 Pointsarguments in the function are just variables that live in the function. when you call a function you define what you want those to be. like
function add(num1, num2) {
num1 + num2;
}
console.log(add(4, 10));
//logs : 14
num1 and num2 are variables that are established in the function(here, here) { and do stuff here; }
you can return info from a function or run a function in a function or do an if statement in a function.
javascript is just functions built on functions. console.log() is a function. so is alert(). you are calling them and passing arguments into them but they are 'built in' to JS.

Jesse Lawson
11,159 Pointsand about storing functions as variables like:
var rad = function(a, b){
return a - b;
}
//lets say you are calling another function and you need to pass the information stored in
//the variable rad. and the the wow() function does something with that information....
wow(rad);
//so the info from rad is being sent to wow and then something will happen with it
//there. The reason for all of this is because you are making a program that will do many
//different things based on input from the user. so not many things are set in stone they
// are set to do this function or that function or both based on how you want the system to flow
//As a programmer, you are programming that flow.
You may want a named function, and anonymous function, or a function stored in a var. depending on what is most effective and sustainable and understandable for your program and other programmers who may need to use your code.
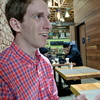
Leyton Parker
6,556 Pointsi think my confusion is with the names of the arguments.
could they just as well be called (first, second)?
when I tried function max(1, 2) {} I get an error, can you only use strings in an argument?
btw when I tried alert( max(1, 2) ); instead of with the strings it worked, thank you :)
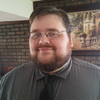
Michael Davidson
5,519 PointsOh, yeah the names can be whatever you want them to be. I usually try to stick to names that make since to make the code easier to read, but it could just as easily be
var max = function(Trex, Velociraptor) {
if (Trex > Velociraptor) {
return Trex;
} else {
return Velociraptor;
}
};
Sticking to things that make sense though, like one and two, make the code easier to read, and easier to come back to in the future.
Word to the wise, from personal experience, don't just use letters becasue they're quicker.... it gets really confusing really fast if you've been away from it for a few weeks XD
Leyton Parker
6,556 PointsLeyton Parker
6,556 PointsThanks Michael,
OK, I thought it was a generic or anonymous function, my first thought was to write it
function max(one, two) {}
then store it in a variable
var max = max(one, two);
so I'm just not sure why it is written
var max = function(one, two) {};
is my first thought incorrect?
Also, would changing the strings to numbers conflict with the strings in my function?